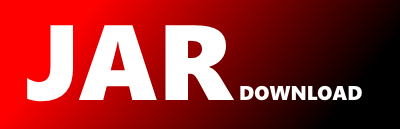
com.adobe.xfa.scripthandler.rhino.ScriptObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.scripthandler.rhino;
import org.mozilla.javascript.Context;
import org.mozilla.javascript.Script;
import org.mozilla.javascript.Scriptable;
import org.mozilla.javascript.ScriptableObject;
import org.mozilla.javascript.debug.DebuggableScript;
import java.util.ArrayList;
import java.util.List;
/**
* A class to deconstruct a compiled script.
* @auther Mike Tardif
* @exclude from published api.
*/
class ScriptObject {
private ScriptableObject moScriptableObj;
private final List mMethods;
private final List mVars;
public ScriptObject(Script oScript) {
assert (oScript != null);
//
// Grab functions and vars.
//
Context context = RhinoEngine.getThreadLocalRuntimeContext();
DebuggableScript view = Context.getDebuggableView(oScript);
mVars = new ArrayList();
mMethods = new ArrayList();
if (view != null) {
//
// Extracting variables and functions...
//
int nVars = view.getParamAndVarCount();
for (int i = 0; i < nVars; i++) {
String name = view.getParamOrVarName(i);
// add a variable to our script object
mVars.add(name);
}
int nFuncs = view.getFunctionCount();
for (int i = 0; i < nFuncs; i++) {
DebuggableScript func = view.getFunction(i);
String name = func.getFunctionName();
// add a method to our script object
mMethods.add(name);
}
}
//
// Compile and create the object.
//
compile(context.decompileScript(oScript, 0));
}
private void compile(String sSource) {
Context context = RhinoEngine.getThreadLocalRuntimeContext();
Scriptable scope = RhinoEngine.getTopLevelScope();
moScriptableObj = (ScriptableObject) context.newObject(scope);
boolean bSuccess = (moScriptableObj != null);
if (! bSuccess)
return;
//
// Tack a newline on the script before we start appending code.
// Otherwise if it ends in a //... style comment,
// our code won't be executed.
//
StringBuilder sBuf = new StringBuilder(sSource);
sBuf.append('\n');
//
// Construct some strings outside the loop for efficiency.
//
final String sThisDot = "this.";
final String sEquals = " = ";
final String sSemiColon = ";\n";
//
// Get the type for the id.
//
for (String sName: mMethods) {
sBuf.append(sThisDot);
sBuf.append(sName);
sBuf.append(sEquals);
sBuf.append(sName);
sBuf.append(sSemiColon);
}
for (String sName: mVars) {
sBuf.append(sThisDot);
sBuf.append(sName);
sBuf.append(sEquals);
sBuf.append(sName);
sBuf.append(sSemiColon);
}
context.evaluateString(moScriptableObj, sBuf.toString(), null, 0, null);
}
public Object get(String name, Scriptable start) {
if (mVars.contains(name))
return ScriptableObject.getProperty(moScriptableObj, name);
return Scriptable.NOT_FOUND;
}
public void put(String name, Scriptable start, Object value) {
if (mVars.contains(name))
ScriptableObject.putProperty(moScriptableObj, name, value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy