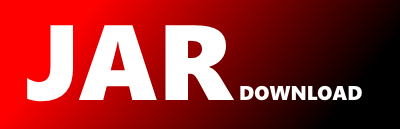
com.adobe.xfa.svg.SVGNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.svg;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.UnitSpan;
/**
* SVGNode is alot like GenericNode. It's a single class that represents many SVG elements.
*
* @exclude from published api.
*/
public class SVGNode extends Element {
public SVGNode(Element parent, Node prevSibling) {
super(parent, prevSibling, null, null, null, null, -1, null);
inhibitPrettyPrint(true);
}
/**
* @see Element#getAttribute(int)
* @exclude from published api.
*/
public Attribute defaultAttribute(int eTag) {
if ((getClassTag() == SVG.TSPANTAG ||
getClassTag() == SVG.ALTGLYPHTAG) &&
(eTag == SVG.FILLTAG ||
eTag == SVG.FONTFAMILYTAG ||
eTag == SVG.FONTSIZETAG ||
eTag == SVG.FONTSTYLETAG ||
eTag == SVG.FONTWEIGHTTAG ||
eTag == SVG.CODEPAGETAG))
{
Element poParentImpl = getXFAParent();
if (poParentImpl != null)
return poParentImpl.getAttribute(eTag);
}
// Not special case, so let the base class handle it
return super.defaultAttribute(eTag);
}
public Attribute getAttribute(int eTag, boolean bPeek, boolean bValidate) {
if (!bPeek && getClassTag() == SVG.TSPANTAG || getClassTag() == SVG.ALTGLYPHTAG) {
Attribute a = super.getAttribute(eTag, true, false);
// If this attribute is not specified, query our parent
// element
if (a == null &&
(eTag == SVG.FILLTAG ||
eTag == SVG.FONTFAMILYTAG ||
eTag == SVG.FONTSIZETAG ||
eTag == SVG.FONTSTYLETAG ||
eTag == SVG.FONTWEIGHTTAG ||
eTag == SVG.CODEPAGETAG))
{
Element poParentImpl = getXFAParent();
if (poParentImpl != null)
return poParentImpl.getAttribute(eTag, bPeek, bValidate);
}
}
return super.getAttribute(eTag, bPeek, bValidate);
}
/**
* Check if this node is an SVG g element and if it defines either the
* caption region or content region of the XFA box model.
*
* @param bCheckCaption --
* if TRUE check caption else check content
* @exclude from published api.
*/
boolean isRegionGroup(boolean bCheckCaption) {
// TODO: JavaPort
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "SVGNode#isRegionGroup");
// The default region group is "content",
// so if we don't find a element make sure our
// return value reflects the default.
// boolean bDefault = (bCheckCaption == false);
//
// if (getClassTag() != SVG.GTAG)
// return false;
//
// Element desc = peekElement(SVG.DESCTAG, false, 0);
//
// if (desc == null)
// return bDefault;
//
// TextData t = desc.peekElement(SVG.TEXTDATATAG, false, 0);
//
// if (t.isNull())
// return bDefault;
//
// if (bCheckCaption)
// return t.getValue().equals(SVG.XFACAPTION);
//
// return t.getValue().equals(SVG.XFACONTENT);
}
/**
* Find the SVG <gesc> element that corresponds to the caption region
* of the XFA box model. This node is identified by having a <desc>
* element with the text: "Caption"
*
* @param bCheckCaption
* if true get the caption node else get content
* @return the caption group. Null object if not found.
* @exclude from published api.
*/
public SVGNode getRegionGroup(boolean bCheckCaption) {
int eTag = getClassTag();
switch (eTag)
{
case SVG.GTAG:
{
if (isRegionGroup(bCheckCaption))
return this;
SVGNode parent = (SVGNode)getXFAParent();
if (parent != null) {
return parent.getRegionGroup(bCheckCaption);
}
}
case SVG.SVGTAG: {
// We expect to find the group under the svg element.
SVGNode child = (SVGNode)getFirstXFAChild();
while (child != null) {
if (child.getClassTag() == SVG.GTAG
&& child.isRegionGroup(bCheckCaption)) {
return child;
}
child = (SVGNode)child.getNextXFASibling();
}
return null;
}
default: {
SVGNode parent = (SVGNode)getXFAParent();
while (parent instanceof SVGNode) {
if (parent.getClassTag() == SVG.SVGTAG)
return parent.getRegionGroup(bCheckCaption);
parent = (SVGNode)parent.getXFAParent();
}
}
}
return null;
}
/**
* Convenience method for setting an attribute that's represented as a
* measurement value
*
* @param eTag -
* the attribute to set
* @param value -
* the value of the attribute
*/
void setMeasurement(int eTag, UnitSpan value) {
String sTemp = Integer.toString(value.valueAsUnit(UnitSpan.POINTS_1K));
setAttribute(new StringAttr(SVGSchema.getSVGSchema().getAtom(eTag), sTemp), eTag);
}
/**
* Convenience method for getting an attribute that's represented as a
* measurement value
*
* @param eTag -
* the attribute to get
* @return value - the value of the attribute
*/
public UnitSpan getMeasurement(int eTag) {
String sNum = getAttribute(eTag).toString();
int nNum = Integer.parseInt(sNum);
return new UnitSpan(UnitSpan.POINTS_1K, UnitSpan.POINTS_1K, nNum);
}
/**
* Convenience method for getting the offset and rotation out of a transform attribute
* in the form of transform="translate(x y) rotate(r)". Note that this method does
* not handle any other transform operations.
* @param x the parsed X offset
* @param y the parsed Y Offset
* @param r the rotation angle
*/
public void parseTransform(UnitSpan x, UnitSpan y, int r) {
// JavaPort: Need to figure out how to return these 3 values...
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "SVGNode#parseTransform");
// void parseTransform(jfUnitSpan& x, jfUnitSpan& y, long& r)
/* String transform = getAttribute(SVG.TRANSFORMTAG).toString();
final int X = 0;
final int Y = 1;
final int R = 2;
final int NONE = 3;
int parsingState=NONE; // NONE - nothing being parsed
// X - translate X being parsed
// Y - translate Y being parsed
// R - rotate being parsed
int vars[] = {0,0,0};
String[] tokens = transform.split("() ,\t");
for (int i=0; i 255) r = 255;
if (g < 0) g = 0;
if (g > 255) g = 255;
if (b < 0) b = 0;
if (b > 255) b = 255;
int nHexNum = b | (g << 8) | (r << 16);
String cHexNum = Integer.toString(nHexNum, 16);
setAttribute(new StringAttr(SVGSchema.getSVGSchema().getAtom(eTag), cHexNum), eTag);
}
/**
* Get a color encoded as #RRGGBB
* @param eTag the attribute to fetch
* @param r Red
* @param g Green
* @param b Blue
* @return The color object
*/
void getColor(int eTag, int r, int g, int b) {
// JavaPort: Figure out how to return 3 values.
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "SVGNode#getColor");
// void getColor(size_t eTag, jfS32& r, jfS32& g, jfS32& b) {
/* r=0;
g=0;
b=0;
String color = getAttribute(eTag).toString();
if (color.length() == 0)
return;
String sNum = color.substring(1,2);
r = Integer.parseInt(sNum, 16);
sNum = color.substring(3,2);
g = Integer.parseInt(sNum, 16);
sNum = color.substring(5,2);
b = Integer.parseInt(sNum, 16);*/
}
/**
* Get the width of this SVG fragment.
* @return the width attribute from the root
© 2015 - 2025 Weber Informatics LLC | Privacy Policy