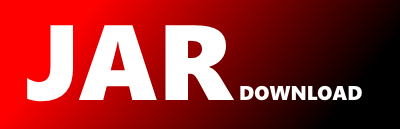
com.adobe.xfa.template.TemplateResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template;
import com.adobe.xfa.template.formatting.Color;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xfa.content.ImageValue;
/**
* Base class for implementing a template resolver. The job of a template
* resolver is to collect different types of objects, etc. for the current job
* being processed. This info can later be used to build structures for devices
* that have a specific requirement, without re-traversing the template.
*
* @exclude from published api.
*/
public final class TemplateResolver {
/**
* A class to represent red/green/blue values.
*
* @exclude from published api.
*/
public static class RGB {
public final int mnBlue;
public final int mnGreen;
public final int mnRed;
RGB(int red, int green, int blue) {
mnRed = red;
mnGreen = green;
mnBlue = blue;
}
public boolean equals(Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
RGB other = (RGB) object;
return other.mnRed == mnRed &&
other.mnGreen == mnGreen &&
other.mnBlue == mnBlue;
}
public int hashCode() {
int hash = 7;
hash = (hash * 31) ^ mnBlue;
hash = (hash * 31) ^ mnGreen;
hash = (hash * 31) ^ mnRed;
return hash;
}
}
private final List moActiveColorList = new ArrayList();
private final List moImageList = new ArrayList();;
public TemplateResolver() {
// Seed the color list with black and white.
// Order for the default black and white pens
// is significant for color PCL, so keep it this way!
RGB oWhite = new RGB(255, 255, 255);
RGB oBlack = new RGB(0, 0, 0);
moActiveColorList.add(oWhite);
moActiveColorList.add(oBlack);
}
/*----------------
Image Methods
----------------*/
/**
* Add a new Color to the list of known colors.
*
* @param oColor
* the color to add to the list.
*/
public void addActiveColor(Color oColor) {
addActiveColor(oColor.getRed(), oColor.getGreen(), oColor.getBlue());
}
/**
* Add a new Color to the list of known colors.
*
* @param nRed
* The red RGB value of the color
* @param nGreen
* The green RGB value of the color.
* @param nBlue
* The blue RGB value of the color.
*/
public void addActiveColor(int nRed, int nGreen, int nBlue) {
boolean bFound = false;
int nSize = moActiveColorList.size();
for (int i = 0; i < nSize; i++) {
RGB color = moActiveColorList.get(i);
if (color.mnRed == nRed && color.mnGreen == nGreen && color.mnBlue == nBlue) {
bFound = true;
break;
}
}
if (!bFound)
moActiveColorList.add(new RGB(nRed, nGreen, nBlue));
}
/**
* Add a reference to a known Imagein the XFATemplate
*
* @param oImage -
* a reference to an Image.
*/
public void addImage(ImageValue oImage) {
boolean bFound = false;
int nSize = moImageList.size();
if (nSize > 0) {
for (int i = 0; i < nSize; i++) {
if (moImageList.get(i) == oImage) {
bFound = true;
break;
}
}
}
if (!bFound)
moImageList.add(oImage);
}
/**
* Remove all image references for this template.
*/
public void cleanupImages() {
moImageList.clear();
}
/**
* Enumerate the list of active colors as RGB structures.
*
* @return an array of the active colors. The list will not contain
* duplicates.
*/
public List enumActiveColors() {
return moActiveColorList;
}
/**
* Return a list of Imagereferences.
*
* @return a list of Imagereferences.
*/
public List getImages() {
return moImageList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy