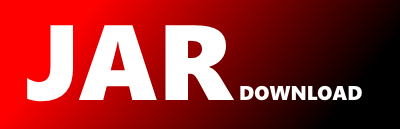
com.adobe.xfa.template.binding.BindingNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.binding;
import org.xml.sax.Attributes;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.XFA;
import com.adobe.xfa.template.containers.Container;
import com.adobe.xfa.ut.StringUtils;
/**
* Generic binding node implementation
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public class BindingNode extends ProtoableNode {
private String msAbsoluteDataRef;
public BindingNode(Element parent, Node prevSibling, String uri, String name,
String qname, Attributes attrs, int classTag, String className) {
super(parent, prevSibling, null, name, qname, attrs, classTag, className);
}
// clone will establish any proto relationships
public Element clone(Element pParent, boolean bDeep) {
BindingNode oClone = (BindingNode) super.clone(pParent, bDeep); // should
// use
// ProtoableNode.clone()
oClone.msAbsoluteDataRef = msAbsoluteDataRef;
return oClone;
}
/**
* Get the absolute data reference for this node
*
* @return The absolute data reference
*/
String getAbsoluteDataRef() {
return msAbsoluteDataRef;
}
/**
* Calculate the current binding context for this node
*
* @return The binding context
*/
String getBindingContext() {
// this should be overridden
return "";
}
String getContextRef(Element oNode, String sConnectionName, int eUsage) {
String sExistingRef = "";
if (oNode == null)
return "";
for (Element oParent = oNode.getXFAParent(); oParent != null
&& oParent != getModel(); oParent = oParent.getXFAParent()) {
if (oParent.isSameClass(XFA.SUBFORMTAG)
|| oParent.isSameClass(XFA.FIELDTAG)
|| oParent.isSameClass(XFA.EXCLGROUPTAG)) {
if (StringUtils.isEmpty(sConnectionName)) {
// default binding
Element oBind = oParent.getElement(XFA.BINDTAG, false, 0,
false, false);
int eMatch = oBind.getEnum(XFA.MATCHTAG);
if (eMatch == EnumAttr.MATCH_DATAREF) {
String sRef = oBind.getAttribute(XFA.REFTAG).toString();
sRef = cleanRelativeRef(sRef);
String sConnector = "";
if ((sRef.length() > 0) && (sExistingRef.length() > 0))
sConnector = ".";
sExistingRef = sRef + sConnector + sExistingRef;
} else if (eMatch == EnumAttr.MATCH_NONE
|| (eMatch == EnumAttr.MATCH_ONCE && oParent
.getName() == "")) {
// continue search upwards
}
else if (oParent.isSameClass(XFA.SUBFORMTAG) && oParent.getXFAParent() == oParent.getModel()) {
// we're at the root subform - implied record binding
if (sExistingRef.length() > 0)
sExistingRef = "$record." + sExistingRef;
else
sExistingRef = "$record";
} else {
sExistingRef = "";
break;
}
} else {
Element oConnect = null;
if (oParent.isSameClass(XFA.SUBFORMTAG) ||
oParent.isSameClass(XFA.FIELDTAG) ||
oParent.isSameClass(XFA.EXCLGROUPTAG)) {
oConnect = ((Container)oParent).getConnectNode(sConnectionName, eUsage, false);
}
if (oConnect == null) {
continue; // no connection matching - contine upwards
}
String sRef = oConnect.getAttribute(XFA.REFTAG).toString();
sRef = cleanRelativeRef(sRef);
String sConnector = "";
if ((sRef.length() > 0) && (sExistingRef.length() > 0))
sConnector = ".";
sExistingRef = sRef + sConnector + sExistingRef;
}
}
if (isAbsoluteDataRef(sExistingRef)) {
return sExistingRef;
}
}
return "";
}
private boolean isAbsoluteDataRef(String sDataRef) {
if (StringUtils.isEmpty(sDataRef))
return false;
if (sDataRef.charAt(0) == '$') {
if (sDataRef.length() > 1 && sDataRef.charAt(1) == '.')
return false;
}
else if (sDataRef.charAt(0) == '!')
return true;
if (sDataRef.startsWith("xfa.datasets."))
return true;
return false;
}
private String cleanRelativeRef(String sDataRef) {
String sCleanRef = sDataRef;
if ((sCleanRef.length() > 1) && (sCleanRef.charAt(0) == '$') && (sCleanRef.charAt(1) == '.'))
sCleanRef = sCleanRef.substring(2);
return sCleanRef;
}
/**
* Set the ref attribute (updates the cache)
* @param sRef the ref attribute to set
* @exclude
*/
void setRef(String sRef) {
// clear any cached absolute reference
msAbsoluteDataRef = "";
// set new ref attribute
setAttribute(new StringAttr(XFA.REF, sRef), XFA.REFTAG);
}
void updateAbsoluteDataRef() {
// this should be overridden
}
//
// inherited from Node
/**
* Determine the absolute data reference for this node and cache it
*
*/
void updateAbsoluteDataRef(String sRef, String sConnection, int eUsage,
boolean bIsPropertyBinding /* =false */) {
msAbsoluteDataRef = sRef;
if ((sRef.length() != 0) && !isAbsoluteDataRef(sRef)) {
String sContextRef = null;
if (bIsPropertyBinding)
sContextRef = getContextRef(this, sConnection, eUsage);
else
sContextRef = getContextRef(getXFAParent(), sConnection, eUsage);
if (sContextRef.length() != 0)
msAbsoluteDataRef = sContextRef + "." + cleanRelativeRef(sRef);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy