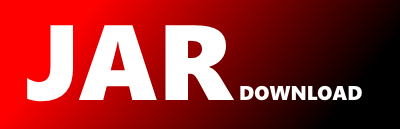
com.adobe.xfa.template.containers.Draw Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.containers;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
import com.adobe.xfa.content.Content;
import com.adobe.xfa.content.DateTimeValue;
import com.adobe.xfa.content.DateValue;
import com.adobe.xfa.content.DecimalValue;
import com.adobe.xfa.content.ExDataValue;
import com.adobe.xfa.content.FloatValue;
import com.adobe.xfa.content.IntegerValue;
import com.adobe.xfa.content.ImageValue;
import com.adobe.xfa.content.TextValue;
import com.adobe.xfa.content.TimeValue;
import com.adobe.xfa.template.ui.UI;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.Numeric;
import com.adobe.xfa.ut.ResId;
/**
* A class to represent the XFA draw
object.
* A draw describes a container capable of non-interactive data content.
*/
public final class Draw extends Container {
/**
* Instantiates a draw container.
* @param parent the draw's parent, if any.
* @param prevSibling the draw's previous sibling, if any.
*/
public Draw(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.DRAW, XFA.DRAW, null, XFA.DRAWTAG, XFA.DRAW);
}
/**
* Get the raw value for this draw, no formatting.
*
* @return the unformatted raw value for this draw, an empty string for
* boilerplate content, or null if the content is null.
*
* @exclude from published api.
*/
public String getRawValue() {
String sValue="";
// get the value
Element pValueNode = getElement(XFA.VALUETAG, true,0,false,false);
// get the value's content
if (pValueNode != null) {
Content pContentNode = (Content)pValueNode.getOneOfChild(true,false);
if (pContentNode == null)
return "";
if (pContentNode.isSameClass(XFA.IMAGETAG)
|| pContentNode.isSameClass(XFA.LINETAG)
|| pContentNode.isSameClass(XFA.RECTANGLETAG)
|| pContentNode.isSameClass(XFA.ARCTAG))
return "";
if (pContentNode.getIsNull())
return "";
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
sValue = ((TextValue)pContentNode).getValue();
} else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
sValue = ((ExDataValue)pContentNode).getValue(false, false, true);
} else if (pContentNode.isSameClass(XFA.DATETAG)) {
sValue = ((DateValue)pContentNode).getValue();
} else if (pContentNode.isSameClass(XFA.DATETIMETAG)) {
sValue = ((DateTimeValue)pContentNode).getValue();
} else if (pContentNode.isSameClass(XFA.TIMETAG)) {
sValue = ((TimeValue)pContentNode).getValue();
} else if (pContentNode.isSameClass(XFA.INTEGERTAG)) {
sValue = ((IntegerValue)pContentNode).toString();
} else if (pContentNode.isSameClass(XFA.FLOATTAG)) {
sValue = Numeric.doubleToString(((FloatValue)pContentNode).getValue(), 8, false);
} else if (pContentNode.isSameClass(XFA.DECIMALTAG)) {
sValue = Numeric.doubleToString(((DecimalValue)pContentNode).getValue(), 8, false);
}
}
return sValue;
}
/**
* Set the raw value for this draw, no formatting.
*
* @param sString
* the unformatted raw value for this draw.
* @exception UnsupportedOperationException
* if you try and set the value of a boilerplate content.
*
* @exclude from published api.
*/
public void setRawValue(String sString) {
Node pContentNode = null;
// get the value
Element pValueNode = getElement(XFA.VALUETAG,0);
// get the value's content
if (pValueNode != null) {
pContentNode = pValueNode.getOneOfChild();
}
if (pContentNode != null) {
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
((TextValue)pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
((ExDataValue)pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.DATETAG)) {
((DateValue)pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.DATETIMETAG)) {
((DateTimeValue)pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.TIMETAG)) {
((TimeValue)pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.INTEGERTAG)) {
((IntegerValue)pContentNode).setValue(sString, false);
} else if (pContentNode.isSameClass(XFA.FLOATTAG)) {
((FloatValue)pContentNode).setValue(sString, false, true, false);
} else if (pContentNode.isSameClass(XFA.DECIMALTAG)) {
((DecimalValue)pContentNode).setValue(sString, false, true, false);
} else if (pContentNode.isSameClass(XFA.IMAGETAG)) {
((ImageValue)pContentNode).setValue(sString, "");
} else {
// "%1 is an unsupported operation for the %2 object%3"
// Note: %3 is extra text if necessary
MsgFormatPos oMessage = new MsgFormatPos(
ResId.UnsupportedOperationException);
oMessage.format("setRawValue");
oMessage.format(pContentNode.getClassAtom());
throw new ExFull(oMessage);
}
}
}
/**
* @exclude from published api.
*/
public Attribute getAttribute(int eTag, boolean bPeek, boolean bValidate) {
Attribute oProperty = super.getAttribute(eTag, bPeek, bValidate);
if (oProperty != null && eTag == XFA.LAYOUTTAG) {
String oValue = oProperty.toString();
if (!oValue.equals("lr-tb") && !oValue.equals("delegate")) {
foundBadAttribute(eTag, oValue);
return defaultAttribute(eTag);
}
}
return oProperty;
}
/**
* @exclude from published api.
*/
public ScriptTable getScriptTable() {
return DrawScript.getScriptTable();
}
/**
* @exclude from published api.
*/
public boolean isWidthGrowSupported() {
// Although the min/max attributes are valid for
// draw elements, not all draw elements should be considered
// growable - arcs/lines/rects etc.
Node poContentNode = null;
// get the value
Element poValueNode = getElement(XFA.VALUETAG, true,0,false,false);
// get the value's content
if (poValueNode != null) {
poContentNode = poValueNode.getOneOfChild(true,false);
}
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.ARCTAG)
|| poContentNode.isSameClass(XFA.LINETAG)
|| poContentNode.isSameClass(XFA.RECTANGLETAG))
return false;
}
// get the ui
UI poUI = (UI) getElement(XFA.UITAG, true,0,false,false);
// get the ui's content
if (poUI != null) {
poContentNode = poUI.getUIElement(true);
}
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.CHOICELISTTAG)
|| poContentNode.isSameClass(XFA.EXOBJECTTAG))
return false;
}
return true;
}
/**
* @exclude from published api.
*/
public boolean isHeightGrowSupported() {
// Although the min/max attributes are valid for
// draw elements, not all draw elements should be considered
// growable - arcs/lines/rects etc.
Node poContentNode = null;
// get the value
Element poValueNode = getElement(XFA.VALUETAG, true,0,false,false);
// get the value's content
if (poValueNode != null) {
poContentNode = poValueNode.getOneOfChild(true,false);
}
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.ARCTAG)
|| poContentNode.isSameClass(XFA.LINETAG)
|| poContentNode.isSameClass(XFA.RECTANGLETAG))
return false;
}
// get the ui
UI poUI = (UI) getElement(XFA.UITAG, true,0,false,false);
// get the ui's content
if (poUI != null) {
poContentNode = poUI.getUIElement(true);
}
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.CHOICELISTTAG)
|| poContentNode.isSameClass(XFA.EXOBJECTTAG))
return false;
}
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy