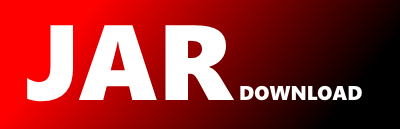
com.adobe.xfa.template.containers.ExclGroupScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.containers;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Arg;
import com.adobe.xfa.Node;
import com.adobe.xfa.NodeList;
import com.adobe.xfa.Obj;
import com.adobe.xfa.Schema;
import com.adobe.xfa.ScriptPropObj;
import com.adobe.xfa.ScriptFuncObj;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
/**
* This class contains all the script functionality associated with the
* ExclGroup class. Broken out into a separate class for easier maintainability.
*
* @exclude from published api.
*/
public class ExclGroupScript extends ContainerScript {
protected static final ScriptTable moScriptTable = new ScriptTable(
ContainerScript.moScriptTable,
"exclGroup",
new ScriptPropObj[] {
new ScriptPropObj(ExclGroupScript.class, "rawValue", "getRawValue", "setRawValue", Arg.INVALID, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_EXCLGRP_RAWVALUE_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, null, "getRawValue", "setRawValue", Arg.INVALID, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_EXCLGRP_RAWVALUE_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "members", "getMembers", null, Arg.OBJECT, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_EXCLGRP_MEMBERS_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "dataNode", "dataNode", null, Arg.OBJECT, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_FORM_DATANODE_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "validationMessage", "getValidationMessage", "setValidationMessage", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_MESSAGE_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "mandatoryMessage", "getMandatoryMessage", "setMandatoryMessage", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_MANDATORYMESSAGE_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "mandatory", "getMandatory", "setMandatory", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_MANDATORY_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "fillColor", "getBackColor", "setBackColor", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_BACKCOLOR_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "borderColor", "getBorderColor", "setBorderColor", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_BORDERCOLOR_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "borderWidth", "getBorderWidth", "setBorderWidth", Arg.STRING, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_BORDERWIDTH_DESC, 0 */, 0),
new ScriptPropObj(ExclGroupScript.class, "errorText", "getErrorText", null, Arg.STRING, Schema.XFAVERSION_30, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_EXCLGRP_ERRORTEXT_DESC, 0 */, 0)
},
new ScriptFuncObj[] {
new ScriptFuncObj(ExclGroupScript.class, "selectedMember", "selectedMember", Arg.OBJECT,
new int[] { Arg.STRING/*, XFA_IS_EXCLGRP_SELECTEDMEMBER_PARAM1*/ }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_CORE|Schema.XFAAVAILABILITY_PLUGIN/*, XFA_IS_EXCLGRP_SELECTEDMEMBER_DESC, XFA_IS_EXCLGRP_SELECTEDMEMBER_RET*/, "selectedMemberPermsCheck", 0),
new ScriptFuncObj(ExclGroupScript.class, "execCalculate", "execCalculate", Arg.EMPTY,
new int[] { }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_FORM_EXECCALCULATE_DESC, 0, null */, 0),
new ScriptFuncObj(ExclGroupScript.class, "execValidate", "execValidate", Arg.BOOL,
new int[] { }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_FORM_EXECVALIDATE_DESC, XFA_IS_FORM_EXECVALIDATE_RET, null */, 0),
new ScriptFuncObj(ExclGroupScript.class, "execInitialize", "execInitialize", Arg.EMPTY,
new int[] { }, 0, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_FORM_EXECINITIALIZE_DESC, 0, null */, 0),
new ScriptFuncObj(ExclGroupScript.class, "execEvent", "execEvent", Arg.EMPTY,
new int[] { Arg.STRING/*, XFA_IS_FORM_EXECEVENT_PARAM1*/ }, 1, Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL/*, XFA_IS_FORM_EXECEVENT_DESC, 0, null */, 0),
}
);
public static ScriptTable getScriptTable() {
return moScriptTable;
}
public static void getRawValue(Obj pObj, Arg oRetVal) {
if (((ExclGroup)pObj).getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING))
oRetVal.setString(((ExclGroup) pObj).getRawValue());
else {
if (!((ExclGroup)(pObj)).getIsNull())
((ExclGroup)pObj).getTypedRawValue(oRetVal);
else
oRetVal.setNull();
}
}
public static void setRawValue(Obj pObj, Arg propertyValue) {
if (((ExclGroup)pObj).getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING))
((ExclGroup) pObj).setRawValue(propertyValue.getString());
else {
String sValue = "";
if (propertyValue.getArgType() != Arg.NULL)
sValue = propertyValue.getAsString(false);
((ExclGroup)pObj).setRawValue(sValue);
}
}
public static void getMembers(Obj pObj, Arg oRetVal) {
oRetVal.setObject(((ExclGroup) pObj).getMembers());
}
public static void getMandatory(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getMandatory());
}
public static void setMandatory(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setMandatory(propertyValue.getString());
}
public static void getValidationMessage(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getMessage(XFA.SCRIPTTEST));
}
public static void setValidationMessage(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setMessage(propertyValue.getString(), XFA.SCRIPTTEST);
}
public static void getMandatoryMessage(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getMessage(XFA.NULLTEST));
}
public static void setMandatoryMessage(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setMessage(propertyValue.getString(), XFA.NULLTEST);
}
public static void getBackColor(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getBackColor());
}
public static void setBackColor(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setBackColor(propertyValue.getString());
}
public static void getBorderColor(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getBorderColor());
}
public static void setBorderColor(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setBorderColor(propertyValue.getString());
}
public static void getBorderWidth(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getBorderWidth());
}
public static void setBorderWidth(Obj pObj, Arg propertyValue) {
((ExclGroup) pObj).setBorderWidth(propertyValue.getString());
}
public static void dataNode(Obj pObj, Arg oRetVal) {
oRetVal.setObject(((ExclGroup) pObj).getDataNode());
}
public static void getErrorText(Obj pObj, Arg oRetVal) {
oRetVal.setString(((ExclGroup) pObj).getErrorText());
}
public static boolean selectedMemberPermsCheck(Obj obj, Arg[] args) {
// if the param count equals 1
// Check permissions on the exclusion group and all its ancestors.
// Check permissions on the current selection.
// Check permissions on the intended selection.
if (args.length != 0 && obj instanceof ExclGroup) {
ExclGroup exclGroup = (ExclGroup)obj;
if (! exclGroup.checkAncestorPerms())
return false;
if (! exclGroup.getSelectedMember().checkDescendentPerms())
return false;
NodeList members = exclGroup.getMembers();
assert members != null;
Node onNode = exclGroup.resolveNode(args[0].getString());
assert onNode != null;
int nLength = members.length();
for (int nIndex = 0; nIndex < nLength; nIndex++) {
Node member = (Node)members.item(nIndex);
assert member != null;
if (member == onNode) {
if (! member.checkDescendentPerms())
return false;
}
}
}
return true;
}
public static void selectedMember(Obj pObj, Arg oRetVal, Arg[] pArgs) {
if (pArgs.length == 1)
((ExclGroup) pObj).setSelectedMember(pArgs[0].getString());
oRetVal.setObject(((ExclGroup) pObj).getSelectedMember());
}
public static void execCalculate(Obj pObj, Arg oRetVal, Arg[] pArgs) {
((ExclGroup) pObj).execEvent(XFA.CALCULATE);
}
public static void execValidate(Obj pObj, Arg oRetVal, Arg[] pArgs) {
oRetVal.setBool(Boolean.valueOf(((ExclGroup) pObj).execValidate()));
}
public static void execInitialize(Obj pObj, Arg oRetVal, Arg[] pArgs) {
((ExclGroup) pObj).execEvent("initialize");
}
public static void execEvent(Obj pObj, Arg oRetVal, Arg[] pArgs) {
((ExclGroup) pObj).execEvent(pArgs[0].getString());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy