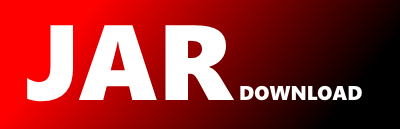
com.adobe.xfa.template.containers.Field Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.containers;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Arg;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.EnumValue;
import com.adobe.xfa.Int;
import com.adobe.xfa.LogMessage;
import com.adobe.xfa.Model;
import com.adobe.xfa.Node;
import com.adobe.xfa.NodeList;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.XFA;
import com.adobe.xfa.content.BooleanValue;
import com.adobe.xfa.content.Content;
import com.adobe.xfa.content.DateTimeValue;
import com.adobe.xfa.content.DateValue;
import com.adobe.xfa.content.DecimalValue;
import com.adobe.xfa.content.ExDataValue;
import com.adobe.xfa.content.FloatValue;
import com.adobe.xfa.content.ImageValue;
import com.adobe.xfa.content.IntegerValue;
import com.adobe.xfa.content.TextValue;
import com.adobe.xfa.content.TimeValue;
import com.adobe.xfa.data.DataNode;
import com.adobe.xfa.form.FormChoiceListField;
import com.adobe.xfa.template.Items;
import com.adobe.xfa.template.TemplateModel;
import com.adobe.xfa.template.formatting.Picture;
import com.adobe.xfa.template.ui.UI;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.LcData;
import com.adobe.xfa.ut.LcLocale;
import com.adobe.xfa.ut.MsgFormatPos;
import com.adobe.xfa.ut.Peer;
import com.adobe.xfa.ut.PictureFmt;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringHolder;
import com.adobe.xfa.ut.StringUtils;
/**
* A class to represent the XFA field
object. A field describes a
* container capable of capturing and presenting data content.
*/
public class Field extends Container {
/**
* @exclude
*/
public static class ItemPair {
public Items mDisplayItems;
public Items mSaveItems;
}
/**
* Instantiates a field container.
*
* @param parent
* the field's parent, if any.
* @param prevSibling
* the field's previous sibling, if any.
* @exclude
*/
public Field(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.FIELD, XFA.FIELD, null,
XFA.FIELDTAG, XFA.FIELD);
}
/**
* @exclude from public api.
*/
public void clearItems() {
// Optimization - Mute messages when deleting all of the items in a
// list, otherwise we will get a message
// for each individual item, in both lists, as they are being deleted.
boolean bSaveMute = isMute();
Element boundItems=null;
try{
if (!bSaveMute)
mute();
ItemPair items = new ItemPair();
// Retrieve the bound and text item lists.
getItemLists(false, items, false);
boundItems = items.mSaveItems;
Element textItems = items.mDisplayItems;
if (boundItems != null)
((Items) boundItems).clearItems(false);
if (textItems != null)
((Items) textItems).clearItems(false);
// Null out the data value to clear out any selected items.
// no longer clear the value of the items list. If we do this we
// loose the data value. In the future we should expose a new script method
// that clears the items and the value.
//setIsNull(true, true);
}finally{
// Restore mute state to unmuted, if it was in unmute state originally
if (!bSaveMute)
unMute();
// Send notification that items have been removed.
notifyPeers(Peer.CHILD_REMOVED, XFA.ITEMS, boundItems);
}
}
/**
* @see Element#defaultElementImpl(int, int, boolean)
* @exclude
*/
protected Node defaultElementImpl(int eTag, int nOccurrence, boolean bAppend /* = true */) {
if (eTag == XFA.ITEMSTAG && nOccurrence == 1) {
// get the first list. don't ask the proto.
Element list1 = super.getElementLocal(XFA.ITEMSTAG, true, 0, false, false);
// If we're asked for the second yet we don't have the first one yet, then
// we must assume they're both default. The second default is defined as
// a copy of the first with the save and presence states flipped.
if (list1 == null) {
list1 = (Element)defaultElementImpl(XFA.ITEMSTAG, 0, false);
}
if (list1 != null) {
// populate the new column with the same element that are in the
// first column
Element list2 = getModel().createElement(bAppend ? this : null,
getLastXMLChild(), null, XFA.ITEMS);
for (Node child = list1.getFirstXFAChild(); child != null; child = child.getNextXFASibling())
child.clone(list2);
// watson bug 1614438 set the presence and save attrs, this will avoid ambiguity later.
int eSave = list1.getEnum(XFA.SAVETAG);
int ePresence = list1.getEnum(XFA.PRESENCETAG);
if (eSave == EnumAttr.BOOL_TRUE)
list2.setAttribute(EnumAttr.BOOL_FALSE, XFA.SAVETAG);
else
list2.setAttribute(EnumAttr.BOOL_TRUE, XFA.SAVETAG);
if (ePresence == EnumAttr.PRESENCE_HIDDEN || ePresence == EnumAttr.PRESENCE_INACTIVE)
list2.setAttribute(EnumAttr.PRESENCE_VISIBLE, XFA.PRESENCETAG);
else
list2.setAttribute(EnumAttr.PRESENCE_HIDDEN, XFA.PRESENCETAG);
return list2;
}
}
return super.defaultElementImpl(eTag, nOccurrence, bAppend);
}
/**
* Delete an item at an index
*
* @param nIndex -
* the index to delete
* @return true if deleted.
* @exclude
*/
public boolean deleteItem(int nIndex) {
boolean bRet = false;
// Retrieve the bound and text item lists.
ItemPair items = new ItemPair();
getItemLists(false, items, false);
Items boundItems = items.mSaveItems;
Items textItems = items.mDisplayItems;
if (boundItems != null && nIndex < boundItems.getXFAChildCount()) {
// De-select the item to remove it from the data.
setItemState(nIndex, false);
boundItems.removeItem(nIndex, false);
if (boundItems != textItems)
textItems.removeItem(nIndex, false);
bRet = true;
}
return bRet;
}
/**
* @exclude from public api.
*/
public void execEvent(String sActivity) {
// overridden by FormField
}
/**
* @exclude from public api.
*/
public boolean execValidate() {
// overridden by FormField
return true;
}
/**
* Get the access/accelerator key (single character)
*
* @return single character value of the keyboard access key
*/
String getAccessKey() {
if (isValidAttr(XFA.ACCESSKEYTAG, false, null)
&& isPropertySpecified(XFA.ACCESSKEYTAG, true, 0)) {
Attribute oAttr = getAttribute(XFA.ACCESSKEYTAG, true, false);
if (oAttr != null && !oAttr.isEmpty()) {
String sAccessKey = oAttr.toString();
return sAccessKey;
}
}
return "";
}
/**
* @exclude from published api.
*/
public Attribute getAttribute(int eTag, boolean bPeek, boolean bValidate) {
Attribute oProperty = super.getAttribute(eTag, bPeek, bValidate);
if (eTag == XFA.LOCALETAG && !bPeek) {
if (oProperty != null && !oProperty.isEmpty())
return oProperty;
//
// Check ancestors for the locale attribute,
//
String sLocale = getInstalledLocale();
return newAttribute(eTag, sLocale);
} else if (oProperty != null && eTag == XFA.LAYOUTTAG) {
String oValue = oProperty.toString();
if (!oValue.equals("lr-tb") && !oValue.equals("delegate")) {
foundBadAttribute(eTag, oValue);
return defaultAttribute(eTag);
}
}
return oProperty;
}
String getBackColor() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pFillNode = pBorderNode.getElement(XFA.FILLTAG, true, 0,
true, false);
if (pFillNode != null) {
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, true,
0, true, false);
if (pColorNode != null)
sRet = pColorNode.getAttribute(XFA.VALUETAG).toString();
}
}
return sRet;
}
String getBorderColor() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, true, 0,
true, false);
if (pEdgeNode != null) {
Element pColorNode = pEdgeNode.getElement(XFA.COLORTAG, true,
0, true, false);
if (pColorNode != null)
sRet = pColorNode.getAttribute(XFA.VALUETAG).toString();
}
}
return sRet;
}
String getBorderWidth() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, true, 0,
true, false);
if (pEdgeNode != null)
sRet = pEdgeNode.getAttribute(XFA.THICKNESSTAG).toString();
}
return sRet;
}
String getBoundItem(String sDisplayText) {
ItemPair items = new ItemPair();
getItemLists(true, items, false);
Element oBoundItems = items.mSaveItems;
Element oTextItems = items.mDisplayItems;
if (oTextItems == null || oBoundItems == null)
return "";
// Find the item with the matching display value
boolean bFound = false;
int nFoundAt = 0;
for (TextValue child = (TextValue)oTextItems.getFirstXFAChild(); child != null; child = (TextValue)child.getNextXFASibling(), nFoundAt++) {
if (child.getValue().equals(sDisplayText)) {
bFound = true;
break;
}
}
// Return the bound value corresponding to the display item index
if (bFound) {
int nIndex = 0;
for (TextValue child = (TextValue)oBoundItems.getFirstXFAChild(); child != null; child = (TextValue)child.getNextXFASibling(), nIndex++) {
if (nIndex == nFoundAt) {
return child.getValue();
}
}
}
return "";
}
String getBoundItem(int nIndex) {
// Retrieve the bound and text item lists.
ItemPair items = new ItemPair();
getItemLists(true, items, false);
// Return the data value for the item at the specified index.
Element oBoundItems = items.mSaveItems;
if (oBoundItems == null)
return "";
// Return the bound value at the specified index.
int nCurrentIndex = 0;
for (TextValue oBoundText = (TextValue)oBoundItems.getFirstXFAChild(); oBoundText != null; oBoundText = (TextValue)oBoundText.getNextXFASibling(), nCurrentIndex++) {
if (nCurrentIndex == nIndex) {
return oBoundText.getValue();
}
}
return "";
}
/**
* @exclude from public api.
*/
public DataNode getDataNode() {
// overridden by XFAFormField
return null;
}
String getDisplayItem(int nIndex) {
// Retrieve the bound and text item lists.
ItemPair items = new ItemPair();
getItemLists(true, items, false);
// Return the display text for the item at the specified index.
Element oTextItems = items.mDisplayItems;
if (oTextItems == null)
return "";
// Return the display value at the specified index.
int nCurrentIndex = 0;
for (Content oDisplayText = (Content)oTextItems.getFirstXFAChild(); oDisplayText != null; oDisplayText = (Content)oDisplayText.getNextXFASibling(), nCurrentIndex++) {
if (nCurrentIndex == nIndex) {
return oDisplayText.toString();
}
}
return "";
}
String getDisplayItem(String sBoundText) {
// Retrieve the bound and text item lists.
ItemPair items = new ItemPair();
getItemLists(true, items, false);
Items boundItems = items.mSaveItems;
Items textItems = items.mDisplayItems;
if (textItems == null || boundItems == null)
return "";
// Find the item with the matching bound value.
boolean bFound = false;
int nFoundAt = 0;
for (Node child = boundItems.getFirstXFAChild(); child != null; child = child.getNextXFASibling()) {
if (child.toString().equals(sBoundText)) {
bFound = true;
break;
}
nFoundAt++;
}
// Return the text item corresponding to the bound value index.
if (bFound) {
int nCurrentIndex = 0;
for (Node oDisplayText = textItems.getFirstXFAChild(); oDisplayText != null; oDisplayText = oDisplayText.getNextXFASibling(), nCurrentIndex++) {
if (nCurrentIndex == nFoundAt) {
String sDisplay = oDisplayText.toString();
Element pUI = getElement(XFA.UITAG, 0);
if (pUI != null){
Node poCurrentUI = pUI.getOneOfChild(true, false);
if (poCurrentUI != null && pUI.isSameClass(XFA.CHOICELISTTAG)){
boolean bLegacyRender = false;
AppModel oAppModel = getAppModel();
if (oAppModel != null){
TemplateModel oTemplateModel = TemplateModel.getTemplateModel(oAppModel, false);
if (oTemplateModel != null){
if (oTemplateModel.getLegacySetting(AppModel.XFA_LEGACY_V32_RENDERING))
bLegacyRender = true;
}
}
if (!bLegacyRender && (sDisplay == null || sDisplay.equals("")))
return new String(" ");
}
}
return sDisplay;
}
}
}
return "";
}
/**
* Get the edit value for this field.
*
* @return the edit value for this field
* @exception UnsupportedOperationException
* if you try and set the value of a boilerplate content. The
* returned edit value will be either
*
* - in the field's edit picture representation, or,
*
- in the field's short locale picture representation,
* or,
*
- in any representation for works-in-progress.
*
*/
String getEditValue() {
// Adobe patent application tracking # B136, entitled "Applying locale
// behaviors to regions of a form", inventors: Gavin McKenzie, Mike
// Tardif, John Brinkman"
// Return the raw value after having any edit
// picture format applied.
// New: If format fails return the raw value
String sRawValue;
try {
sRawValue = getRawValue();
} catch (ExFull oErr) {
// If we caught an exception trying to get a proper edit
// value, then we likely have bad data.
// Issue a warning and then continue.
getModel().addErrorList(oErr, LogMessage.MSG_WARNING, this);
Element pValueNode = getElement(XFA.VALUETAG, true, 0, true, false);
// get the value's content
if (pValueNode != null) {
Content pContent = (Content) pValueNode.getOneOfChild(false,
true);
if (pContent != null)
return pContent.getStrValue();
}
return "";
}
// Check if we are a drop down box with bound values
// and get the corresponding display value
// getDisplayValue will return an empty string if we are not.
String sDisplay = getDisplayItem(sRawValue);
if (!StringUtils.isEmpty(sDisplay))
return sDisplay;
Element poUI = getElement(XFA.UITAG, true, 0, false, false);
Picture poEditPicture = null;
if (poUI != null)
poEditPicture = (Picture) poUI.getElement(XFA.PICTURETAG, true, 0,
false, false);
if (poEditPicture != null) {
StringBuilder sFormatted = new StringBuilder();
String sLocale = getInstalledLocale();
boolean bSuccess = poEditPicture.formatString(sRawValue, sLocale,
sFormatted);
if (bSuccess)
return sFormatted.toString();
}
else if (!StringUtils.isEmpty(sRawValue)) {
Element poValueNode = getElement(XFA.VALUETAG, true, 0, true, false);
Element poContentNode = null;
if (poValueNode != null)
poContentNode = (Element) poValueNode.getOneOfChild(false, true);
//
// If this is a time, date, datetime, float or decimal
// field w/o edit pictures, Then try to format the
// raw value using the locale's short formats.
//
if (poContentNode != null) {
StringBuilder sFormatted = new StringBuilder();
//
// Avoid fetching the locale and constructing the LcData
// object until absolutely needed.
//
if (poContentNode.isSameClass(XFA.DATETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateFormat(LcData.SHORT_FMT);
PictureFmt.formatDate(sRawValue, sPict, sLocale,
sFormatted, true);
} else if (poContentNode.isSameClass(XFA.TIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getTimeFormat(LcData.SHORT_FMT);
PictureFmt.formatTime(sRawValue, sPict, sLocale,
sFormatted, true);
} else if (poContentNode.isSameClass(XFA.DATETIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateTimeFormat(LcData.SHORT_FMT,
LcData.WITH_CATEGORIES);
String sDatePict = oData.getDateFormat(LcData.SHORT_FMT);
String sTimePict = oData.getTimeFormat(LcData.SHORT_FMT);
PictureFmt.formatDateTime(sRawValue, sPict, sDatePict,
sTimePict, sLocale, sFormatted, true);
} else if (poContentNode.isSameClass(XFA.INTEGERTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale integral format w/o grouping symbols.
//
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
int nOptn = LcData.WITHOUT_GROUPINGS;
String sPict = oData.getNumberFormat(LcData.INTEGRAL_FMT,
nOptn);
PictureFmt.formatNumeric(sRawValue, sPict, sLocale,
sFormatted);
} else if (poContentNode.isSameClass(XFA.FLOATTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale decimal format w/o grouping symbols
// to the field's value width and precision.
//
String sLocale = getInstalledLocale();
int nOptn = LcData.WITHOUT_GROUPINGS;
LcData oData = new LcData(LcLocale.DEFAULT_LOCALE);
int nPrec = oData.getNumberPrecision(sRawValue);
nOptn |= LcData.withPrecision(nPrec);
int nWidth = sRawValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
if (sLocale != LcLocale.DEFAULT_LOCALE)
oData = new LcData(sLocale);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT,
nOptn);
PictureFmt.formatNumeric(sRawValue, sPict, sLocale,
sFormatted);
} else if (poContentNode.isSameClass(XFA.DECIMALTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale decimal format w/o grouping symbols
// to the field's value width and precision. If the field's
// fracDigits attribute is -1, then use picture 8 symbols.
//
Int oFrac = (Int) poContentNode
.getAttribute(XFA.FRACDIGITSTAG);
String sLocale = getInstalledLocale();
int nOptn = LcData.WITHOUT_GROUPINGS;
if (oFrac.getValue() == -1)
nOptn |= LcData.WITH_EIGHTS;
LcData oData = new LcData(LcLocale.DEFAULT_LOCALE);
int nPrec = oData.getNumberPrecision(sRawValue);
nOptn |= LcData.withPrecision(nPrec);
int nWidth = sRawValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
if (sLocale != LcLocale.DEFAULT_LOCALE)
oData = new LcData(sLocale);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT,
nOptn);
PictureFmt.formatNumeric(sRawValue, sPict, sLocale,
sFormatted);
}
//
// If successful Then return the formatted result.
//
if (!StringUtils.isEmpty(sFormatted))
return sFormatted.toString();
}
}
return sRawValue;
}
String getForeColor() {
String sRet = "";
Element pFontNode = getElement(XFA.FONTTAG, true, 0, true, false);
if (pFontNode != null) {
Element pFillNode = pFontNode.getElement(XFA.FILLTAG, true, 0,
true, false);
if (pFillNode != null) {
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, true,
0, true, false);
if (pColorNode != null)
sRet = pColorNode.getAttribute(XFA.VALUETAG).toString();
}
}
return sRet;
}
//
// XFA Subset convinience methods
//
/**
* Gets the formatted value for this field.
* The returned formatted value will be either
*
* - the field's format picture representation, or,
*
- the field's default locale picture representation, or,
*
- any representation for works-in-progress.
*
* @return the formatted value for this field,
* or null if the content is null.
*/
public String getFormattedValue() {
String sValue = getRawValue();
// Check if we are a drop down box with bound values
// and get the corresponding display value
// getDisplayValue will return an empty string if we are not.
String sDisplay = getDisplayItem(sValue);
if (!StringUtils.isEmpty(sDisplay))
return sDisplay;
Element pContentNode = null;
// get the value
Element pValueNode = getElement(XFA.VALUETAG, true, 0, true, false);
// get the value's content
if (pValueNode != null)
pContentNode = (Element) pValueNode.getOneOfChild(false, true);
boolean bNumericField = (pContentNode instanceof DecimalValue
|| pContentNode instanceof IntegerValue || pContentNode instanceof FloatValue);
// check for any picture formatting
Element poFormatNode = getElement(XFA.FORMATTAG, true, 0, false, false);
if (sValue != null && poFormatNode != null) {
Picture poPictureNode = (Picture) poFormatNode.getElement(
XFA.PICTURETAG, true, 0, false, false);
if (poPictureNode != null && !StringUtils.isEmpty(poPictureNode.getValue())) {
// Adobe patent application tracking # B136, entitled "Applying
// locale behaviors to regions of a form",
// inventors: Gavin McKenzie, Mike Tardif, John Brinkman"
String sLocale = getInstalledLocale();
StringBuilder sFormatted = new StringBuilder();
boolean bPictureSucceed = poPictureNode.formatString(sValue,
sLocale, sFormatted);
double dVal = 0.0;
//
// If formatting succeeded Then display formatted result.
//
if (bPictureSucceed)
return sFormatted.toString();
//
// Else if field is numeric and input is numeric then...
//
else if (bNumericField) {
boolean bValidDouble = true;
try {
dVal = Double.parseDouble(sValue);
} catch (NumberFormatException e) {
bValidDouble = false;
}
if (bValidDouble) {
//
// If input is a zero value, e.g., 0.00
// then display a localized "0".
//
if (dVal == 0) {
LcData oData = new LcData(sLocale);
return oData.getZeroSymbol();
}
}
}
}
}
if (StringUtils.isEmpty(sValue))
return null;
//
// If this is a time, date, datetime, float or decimal
// field w/o format pictures, Then try to format the
// raw value using the locale's default formats.
//
if (pContentNode != null) {
StringBuilder sFormatted = new StringBuilder();
//
// Avoid fetching the locale and constructing the LcData
// object until absolutely needed.
//
// Adobe patent application tracking # B136, entitled "Applying
// locale behaviors to regions of a form", inventors: Gavin
// McKenzie, Mike Tardif, John Brinkman"
if (pContentNode.isSameClass(XFA.DATETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateFormat(LcData.MED_FMT);
PictureFmt.formatDate(sValue, sPict, sLocale, sFormatted, true);
} else if (pContentNode.isSameClass(XFA.TIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getTimeFormat(LcData.MED_FMT);
PictureFmt.formatTime(sValue, sPict, sLocale, sFormatted, true);
} else if (pContentNode.isSameClass(XFA.DATETIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateTimeFormat(LcData.MED_FMT,
LcData.WITH_CATEGORIES);
String sDatePict = oData.getDateFormat(LcData.MED_FMT);
String sTimePict = oData.getTimeFormat(LcData.MED_FMT);
PictureFmt.formatDateTime(sValue, sPict, sDatePict, sTimePict,
sLocale, sFormatted, true);
} else if (pContentNode.isSameClass(XFA.INTEGERTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale integral format w/ grouping symbols.
//
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
int nOptn = LcData.WITH_GROUPINGS;
String sPict = oData
.getNumberFormat(LcData.INTEGRAL_FMT, nOptn);
PictureFmt.formatNumeric(sValue, sPict, sLocale, sFormatted);
} else if (pContentNode.isSameClass(XFA.FLOATTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale decimal format w/ grouping symbols
// to the field's value width and precision.
//
String sLocale = getInstalledLocale();
int nOptn = LcData.WITH_GROUPINGS;
LcData oData = new LcData(LcLocale.DEFAULT_LOCALE);
int nPrec = oData.getNumberPrecision(sValue);
nOptn |= LcData.withPrecision(nPrec);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
if (sLocale != LcLocale.DEFAULT_LOCALE)
oData = new LcData(sLocale);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT, nOptn);
PictureFmt.formatNumeric(sValue, sPict, sLocale, sFormatted);
} else if (pContentNode.isSameClass(XFA.DECIMALTAG)) {
//
// Try to format the field's canonical value according to
// the field's locale decimal format w/ grouping symbols
// to the field's value width and precision. If the field's
// fracDigits attribute is -1, then use picture 8 symbols.
//
Int oFrac = (Int) pContentNode.getAttribute(XFA.FRACDIGITSTAG);
String sLocale = getInstalledLocale();
int nOptn = LcData.WITH_GROUPINGS;
if (oFrac.getValue() == -1)
nOptn |= LcData.WITH_EIGHTS;
LcData oData = new LcData(LcLocale.DEFAULT_LOCALE);
int nPrec = oData.getNumberPrecision(sValue);
nOptn |= LcData.withPrecision(nPrec);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
if (sLocale != LcLocale.DEFAULT_LOCALE)
oData = new LcData(sLocale);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT, nOptn);
PictureFmt.formatNumeric(sValue, sPict, sLocale, sFormatted);
}
//
// If successful Then return the formatted value.
//
if (!StringUtils.isEmpty(sFormatted))
return sFormatted.toString();
}
return sValue;
}
/**
* Get the display and save lists
*
* @param bPeek -
* if TRUE return lists that must not be modified
* @param items -
* a structure holding the bound and display elements
* @param bEnforceMultiColumn -
* @exclude from public api.
*/
public void getItemLists(boolean bPeek, ItemPair items,
boolean bEnforceMultiColumn /* = false */) {
// First find the node that contains the bound elements
// (value returned when selected)
// This node will have a save attribute (save="1")
Items saveList = null;
Items displayList = null;
// get the first list
Items list1 = (Items) getElement(XFA.ITEMSTAG, bPeek, 0, false, false);
// get the second list.
Items list2 = (Items) getElement(XFA.ITEMSTAG, true, 1, false, false);
if (!bPeek) {
if (list2 != null && list2.getXFAParent() != this) {
// if we are not peeking copy it over if we don't have the
// correct parent
list2 = (Items) list2.createProto(this, false);
}
if (list2 == null && bEnforceMultiColumn) {
// populate the new column with the same element that are in the
// first column
list2 = (Items) defaultElementImpl(XFA.ITEMSTAG, 1, true);
}
}
if (list1 == null)
return;
// we have two lists
if (list2 != null) {
int ePresence1 = list1.getEnum(XFA.PRESENCETAG);
int eSave1 = list1.getEnum(XFA.SAVETAG);
int ePresence2 = list2.getEnum(XFA.PRESENCETAG);
int eSave2 = list2.getEnum(XFA.SAVETAG);
// if both have the same settings
if (ePresence1 == ePresence2 && eSave1 == eSave2) {
// default to the first list
displayList = list1;
saveList = list1;
// update the attributes so they are correct
bEnforceMultiColumn = true;
} else if (ePresence1 == ePresence2) {
if (eSave1 == EnumAttr.BOOL_TRUE) {
saveList = list1;
displayList = list2;
} else {
saveList = list2;
displayList = list1;
}
// update the attributes so they are correct
if (!bPeek) {
displayList.setAttribute(EnumAttr.PRESENCE_VISIBLE, XFA.PRESENCETAG);
saveList.setAttribute(EnumAttr.PRESENCE_HIDDEN, XFA.PRESENCETAG);
}
} else if (eSave1 == eSave2) {
if (ePresence1 != EnumAttr.PRESENCE_HIDDEN && ePresence1 != EnumAttr.PRESENCE_INACTIVE) {
displayList = list1;
saveList = list2;
} else {
displayList = list2;
saveList = list1;
}
// update the attributes so they are correct
if (!bPeek) {
saveList.setAttribute(EnumAttr.BOOL_TRUE, XFA.SAVETAG);
displayList.setAttribute(EnumAttr.BOOL_FALSE, XFA.SAVETAG);
}
} else { // both have different settings
if (ePresence1 != EnumAttr.PRESENCE_HIDDEN && ePresence1 != EnumAttr.PRESENCE_INACTIVE)
displayList = list1;
else
displayList = list2;
if (eSave1 == EnumAttr.BOOL_TRUE)
saveList = list1;
else
saveList = list2;
if (displayList == saveList)
bEnforceMultiColumn = true;
}
} else { // only one list
displayList = list1;
saveList = list1;
}
// JAVAPORT: dead code - displayList and saveList are always non-null at this point
// // if there are no visible columns, use the first column
// if (displayList == null)
// displayList = list1;
//
// // if there is no save column, use the first column, even if
// // it has presence="visible"
// if (saveList == null)
// saveList = list1;
// both lists point to the same node
if (!bPeek && bEnforceMultiColumn) {
// ensure we have different lists
if (displayList == saveList) {
if (displayList == list1 && list2 != null)
saveList = list2;
else
saveList = list1;
// update the lists with the correct attrs
saveList.setAttribute(EnumAttr.BOOL_TRUE, XFA.SAVETAG);
saveList.setAttribute(EnumAttr.PRESENCE_HIDDEN,
XFA.PRESENCETAG);
displayList.setAttribute(EnumAttr.BOOL_FALSE, XFA.SAVETAG);
displayList.setAttribute(EnumAttr.PRESENCE_VISIBLE,
XFA.PRESENCETAG);
}
// ensure we have the correct item counts
int nSaveCount = saveList.getXFAChildCount();
int nDisplayCount = displayList.getXFAChildCount();
if (nDisplayCount != nSaveCount) {
saveList.clearItems(false);
// replace the values
for (Node child = displayList.getFirstXFAChild(); child != null; child = child.getNextXFASibling()) {
child.clone(saveList);
}
}
}
items.mSaveItems = saveList;
items.mDisplayItems = displayList;
}
boolean getItemState(String sValue, boolean bDisplay) {
Element oSearch;
ItemPair items = new ItemPair();
// Retrieve the bound and text item lists.
getItemLists(true, items, false);
Element boundItems = items.mSaveItems;
Element oDisplayItems = items.mDisplayItems;
if (bDisplay)
oSearch = oDisplayItems;
else
oSearch = boundItems;
int i = 0;
for (TextValue oText = (TextValue)oSearch.getFirstXFAChild(); oText != null; oText = (TextValue)oText.getNextXFASibling(), i++) {
String sItemValue = oText.getValue();
if (sValue.equals(sItemValue)) {
return getItemState(i);
}
}
return false;
}
boolean getItemState(int nIndex) {
// Return TRUE if the item at the specified index is currently selected,
// FALSE otherwise.
// Get the bound value at the selected index.
String sBoundItem = getBoundItem(nIndex);
if (StringUtils.isEmpty(sBoundItem))
return false;
// get the value
Element pValueNode = getElement(XFA.VALUETAG, true, 0, false, false);
// get the value's content
if (pValueNode != null) {
Content pContentNode = (Content) pValueNode.getOneOfChild(true,
false);
if (pContentNode == null || pContentNode.getIsNull()) {
return false;
}
// Single-select listbox.
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
String sValue = ((TextValue) pContentNode).getValue();
if (sBoundItem.equals(sValue))
return true;
}
// Multi-select listbox.
else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
List oValues = new ArrayList();
ExDataValue oExData = (ExDataValue) pContentNode;
oExData.getValues(oValues);
for (int i = 0; i < oValues.size(); i++) {
if (sBoundItem.equals(oValues.get(i)))
return true;
}
}
}
return false;
}
/**
* return the item value
*
* @param sItemsIndex
* @param sItemIndex
* @return null if there's no item value, else the item value string.
*/
String getItemValue(int nItemsIndex, int nItemIndex) {
Element pItems = getElement(XFA.ITEMSTAG, true, nItemsIndex, false,
false);
if (pItems != null) {
Node pText = pItems.getFirstXFAChild();
int nItemCount = 0;
while (pText != null) {
// 'On' value is first in
// 'Off' value is second in
if (pText.isSameClass(XFA.TEXTTAG)
|| pText.isSameClass(XFA.INTEGERTAG)) {
if (nItemCount == nItemIndex) {
return pText.toString();
}
nItemCount++;
if (nItemCount > nItemIndex)
return null;
}
pText = pText.getNextXFASibling();
}
}
return null;
}
int getItemsLength() {
ItemPair items = new ItemPair();
getItemLists(true, items, false);
Element oBoundItems = items.mSaveItems;
if (oBoundItems != null)
return oBoundItems.getXFAChildCount();
return 0;
}
String getMandatory() {
String sRet = "";
Element pValidateNode = getElement(XFA.VALIDATETAG, true, 0, true,
false);
if (pValidateNode != null)
sRet = pValidateNode.getAttribute(XFA.NULLTESTTAG).toString();
return sRet;
}
String getMessage(String aType) {
String sRet = "";
Element pValidateNode = getElement(XFA.VALIDATETAG, true, 0, false,
false);
if (pValidateNode != null)
sRet = TemplateModel.getValidationMessage(pValidateNode, aType);
return sRet;
}
/**
* Get the neutral value of this field
*
* @return String for Neutral value, null if undefined. This method is for
* checkbutton fields only.
*
* @exclude from published api
*/
public String getNeutralValue() {
Element oUI = getElement(XFA.UITAG, true, 0, false, false);
// get current ui
if (oUI != null) {
Node oCurrentUI = oUI.getOneOfChild();
if (oCurrentUI != null) {
if (oCurrentUI.isSameClass(XFA.CHECKBUTTONTAG))
return getItemValue(0, 2);
}
}
return null;
}
/**
* @see Element#getNodes()
* @exclude
*/
public NodeList getNodes() {
Model oModel = getModel();
assert(oModel != null);
// Watson bug 1333106 ensure we copy over all the items when we call get nodes
// we do this because in Version 7 getNodes() would return the items
// for performance only resolve the items after the load is complete
if (oModel != null && !oModel.isLoading())
lazyResolveItemsArrays(false);
return super.getNodes();
}
/**
* @see ProtoableNode#resolveAndEnumerateChildren(boolean bAllProperties, boolean bFirstDefaultOnly)
* @exclude from public api.
*/
public NodeList resolveAndEnumerateChildren(boolean bAllProperties /* = false */, boolean bFirstDefaultOnly /* = false */) {
// Our get-default-elements code for can't handle two-step
// proto resolution at once, so make sure we resolve the
// arrays first.
if (bAllProperties)
lazyResolveItemsArrays(bAllProperties);
return super.resolveAndEnumerateChildren(bAllProperties, bFirstDefaultOnly);
}
/**
* Get the off value of this field
*
* @return The Off value. If none, null This method is for checkbutton
* fields only.
*
* @exclude from public api.
*/
public String getOffValue() {
Element oUI = getElement(XFA.UITAG, true, 0, false, false);
// get current ui
if (oUI != null) {
Node oCurrentUI = oUI.getOneOfChild();
if (oCurrentUI != null) {
if (oCurrentUI.isSameClass(XFA.CHECKBUTTONTAG))
return getItemValue(0, 1);
}
}
return null;
}
/**
* Get the on value of this field
*
* @return The On value, null if there isn't one. This method is for
* checkbutton fields only.
*
* @exclude from public api.
*/
public String getOnValue() {
Element oUI = getElement(XFA.UITAG, true, 0, false, false);
// get current ui
if (oUI != null) {
Node oCurrentUI = oUI.getOneOfChild();
if (oCurrentUI != null) {
if (oCurrentUI.isSameClass(XFA.CHECKBUTTONTAG))
return getItemValue(0, 0);
}
}
return null;
}
Subform getParentSubform() {
Element pParent = getXFAParent();
while (pParent != null) {
if (pParent.isSameClass(XFA.SUBFORMTAG)) {
EnumValue eScope = (EnumValue) pParent
.getAttribute(XFA.SCOPETAG);
if (eScope.getInt() == EnumAttr.SCOPE_NONE)
return (Subform) pParent;
}
pParent = pParent.getXFAParent();
}
return null;
}
/**
* Gets the raw value for this field.
* The returned unformatted value will be either
*
* - the field's canonical representation, or,
*
- any representation for works-in-progress.
*
* @return the unformatted raw value for this field,
* or null if the content is null.
*/
public String getRawValue() {
Arg oRawValue = new Arg();
getTypedRawValue(oRawValue);
if (oRawValue.getArgType() != Arg.NULL)
return oRawValue.getAsString(false);
return null;
}
/**
* @exclude from published api.
*/
public ScriptTable getScriptTable() {
return FieldScript.getScriptTable();
}
int getSelectedIndex() {
// Retrieve the bound and text item lists.
ItemPair items = new ItemPair();
getItemLists(true, items, false);
// Return the index of the first selected item.
Element oBoundItems = items.mSaveItems;
if (oBoundItems == null)
return -1;
// get the value's content
Element pValueNode = getElement(XFA.VALUETAG, true, 0, false, false);
if (pValueNode != null) {
Node pContentNode = pValueNode.getOneOfChild(true, false);
if (pContentNode == null || ((Content) pContentNode).getIsNull()) {
return -1;
}
// Single-select listbox.
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
String sDataValue = ((TextValue) pContentNode).getValue();
// Find the first selected item
int nFoundAt = 0;
for (TextValue oText = (TextValue)oBoundItems.getFirstXFAChild(); oText != null; oText = (TextValue)oText.getNextXFASibling(), nFoundAt++) {
String sItemValue = oText.getValue();
if (sItemValue.equals(sDataValue))
return nFoundAt;
}
}
// Multi-select listbox.
else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
List oValues = new ArrayList();
ExDataValue oExData = (ExDataValue) pContentNode;
oExData.getValues(oValues);
// Find the first selected item
int nFoundAt = 0;
for (TextValue oText = (TextValue)oBoundItems.getFirstXFAChild(); oText != null; oText = (TextValue)oText.getNextXFASibling(), nFoundAt++) {
String sItemValue = oText.getValue();
for (int i = 0; i < oValues.size(); i++) {
if (sItemValue.equals(oValues.get(i)))
return nFoundAt;
}
}
}
}
return -1;
}
void getTypedRawValue(Arg oValue) {
// get the value
Element pValueNode = getElement(XFA.VALUETAG, true, 0, false, false);
// get the value's content
if (pValueNode != null) {
Content pContentNode = (Content) pValueNode.getOneOfChild(true, false);
if (pContentNode == null || (pContentNode instanceof Content && ((Content) pContentNode).getIsNull())) {
oValue.setNull();
return;
}
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
oValue.setString(((TextValue) pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
// watson bug 1795969 old forms need to use the legacy XHTML processing
boolean bLegacy = getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V27_XHTMLVERSIONPROCESSING);
oValue.setString(((ExDataValue) pContentNode).getValue(false, false, bLegacy));
}
else if (pContentNode.isSameClass(XFA.BOOLEANTAG)) {
if (((BooleanValue)pContentNode).valueHasTypeMismatch()) {
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING)) {
// legacy behavior is to return false
oValue.setBool(false);
}
else {
// never return a value to the user different than the data; if the
// data is invalid then just return it as a string
oValue.setString(((Content)pContentNode).getStrValue());
}
}
else
oValue.setBool(((BooleanValue)pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.DATETAG)) {
oValue.setString(((DateValue) pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.DATETIMETAG)) {
oValue.setString(((DateTimeValue) pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.TIMETAG)) {
oValue.setString(((TimeValue) pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.INTEGERTAG)) {
String sValue = ((Content)pContentNode).getStrValue();
if (StringUtils.isEmpty(sValue))
oValue.setNull();
else if (((IntegerValue)pContentNode).valueHasTypeMismatch()) {
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING)) {
// legacy behavior is to return zero
oValue.setInteger(((IntegerValue)pContentNode).getValue());
}
else {
// never return a value to the user different than the data; if the
// data is invalid then just return it as a string
oValue.setString(sValue);
}
}
else
oValue.setInteger(((IntegerValue)pContentNode).getValue());
}
else if (pContentNode.isSameClass(XFA.FLOATTAG)) {
String sValue = ((Content)pContentNode).getStrValue();
if (StringUtils.isEmpty(sValue))
oValue.setNull();
else if (((FloatValue)pContentNode).valueHasTypeMismatch()) {
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING)) {
// legacy behavior is to return zero
oValue.setDouble(((FloatValue)pContentNode).getValue());
}
else {
// never return a value to the user different than the data; if the
// data is invalid then just return it as a string
oValue.setString(sValue);
}
}
else
oValue.setDouble(((FloatValue)pContentNode).getValue());
} else if (pContentNode.isSameClass(XFA.DECIMALTAG)) {
String sValue = ((Content)pContentNode).getStrValue();
if (StringUtils.isEmpty(sValue))
oValue.setNull();
else if (((DecimalValue)pContentNode).valueHasTypeMismatch()) {
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V29_SCRIPTING)) {
// legacy behavior is to return raw string if fracDigits is -1,
// and zero otherwise
Int oFracInt = (Int) pContentNode.getAttribute(XFA.FRACDIGITSTAG);
if (oFracInt.getValue() == -1)
oValue.setString(sValue);
else
oValue.setDouble(((DecimalValue)pContentNode).getValue());
}
else {
// never return a value to the user different than the data; if the
// data is invalid then just return it as a string
oValue.setString(sValue);
}
}
else {
Int oFracInt = (Int)pContentNode.getAttribute(XFA.FRACDIGITSTAG);
if (oFracInt.getValue() == -1)
oValue.setString(sValue);
else
oValue.setDouble(((DecimalValue)pContentNode).getValue());
}
}
else if (pContentNode.isSameClass(XFA.IMAGETAG)) {
oValue.setString(((ImageValue)pContentNode).getValue());
}
} else
oValue.setNull();
}
/**
* @exclude from public api.
*/
public boolean hasValidFormattedValue() {
// This is necessary since getFormattedVAlue
// now returns the raw value when value is incompatible
// with picture clause. This function lets you know
// if picture format is valid wrt rawvalue.
String sValue = getRawValue();
if (isPropertySpecified(XFA.VALIDATETAG, true, 0)) {
// check for any picture formatting
Element poFormatNode = getElement(XFA.VALIDATETAG, true, 0, false,
false);
if (poFormatNode != null) {
Picture poPictureNode = (Picture) poFormatNode.getElement(
XFA.PICTURETAG, true, 0, false, false);
if (poPictureNode != null) {
String sLocale = getInstalledLocale();
StringBuilder s = new StringBuilder();
return poPictureNode.formatString(sValue, sLocale, s);
}
}
}
return true;
}
/**
* @see Container#isConnectSupported()
* @exclude
*/
public boolean isConnectSupported() {
return true;
}
/**
* @exclude from published api.
*/
public boolean isHeightGrowSupported() {
return isWidthGrowSupported();
}
/**
* Return whether or not this container is 'on' in its ExclGroup
*
* @return true if this container is 'on'.
*/
boolean isOn() {
boolean bRet = false;
String sTemp = getOnValue();
if (sTemp != null) {
String sValue = getFormattedValue();
if (sTemp.equals(sValue))
bRet = true;
} else if (!getIsNull())
bRet = true;
return bRet;
}
/**
* @exclude from published api.
*/
public boolean isWidthGrowSupported() {
// Although element always supports min/max[w/h] attributes,
// not all fields support growability. Eg text fields do but
// list box fields and image fields do not.
// button=yes
// checkbutton=yes
// choicelist=no
// datetime=yes
// defaultUI=no?
// exobject=no
// numericedit=yes
// passwordedit=yes
// texedit=yes
// image field=no
Element poContentNode = null;
UI poUI = (UI) getElement(XFA.UITAG, true, 0, false, false);
if (poUI != null) {
poContentNode = poUI.getUIElement(true);
}
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.CHOICELISTTAG)
|| poContentNode.isSameClass(XFA.EXOBJECTTAG)
|| poContentNode.isSameClass(XFA.BARCODETAG)) {
return false;
}
}
Element poValue = getElement(XFA.VALUETAG, true, 0, false, false);
if (poValue != null) {
poContentNode = (Element) poValue.getOneOfChild(true, false);
if (poContentNode != null) {
if (poContentNode.isSameClass(XFA.RECTANGLETAG)
|| poContentNode.isSameClass(XFA.ARCTAG)) {
return false;
}
}
}
return true;
}
/**
* @exclude from public api.
*/
protected boolean notifyParent() {
Element pParent = getXFAParent();
if (pParent instanceof ExclGroup)
return true;
return false;
}
void setBackColor(String sString) {
Element pBorderNode = getElement(XFA.BORDERTAG, false, 0, false, false);
Element pFillNode = pBorderNode.getElement(XFA.FILLTAG, false, 0,
false, false);
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, false, 0,
false, false);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString),
XFA.VALUETAG);
}
void setBorderColor(String sString) {
Element pBorderNode = getElement(XFA.BORDERTAG, false, 0, false, false);
// need to set the 4 edges
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.EDGETAG, true, i))
break;
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, false, i,
false, false);
// set the color
Element pColorNode = pEdgeNode.getElement(XFA.COLORTAG, false, 0,
false, false);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString),
XFA.VALUETAG);
}
// need to set the 4 corners
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.CORNERTAG, true, i))
break;
Element pCornerNode = pBorderNode.getElement(XFA.CORNERTAG, false,
i, false, false);
// set the color
Element pColorNode = pCornerNode.getElement(XFA.COLORTAG, false, 0,
false, false);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString),
XFA.VALUETAG);
}
}
void setBorderWidth(String sString) {
// TODO change back to getElement once prot changes are in;
Element pBorderNode = getElement(XFA.BORDERTAG, false, 0, false, false);
// need to set the 4 edges
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.EDGETAG, true, i))
break;
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, false, i,
false, false);
// set the thickness
pEdgeNode.setAttribute(new StringAttr(XFA.THICKNESS, sString),
XFA.THICKNESSTAG);
}
// need to set the 4 corners
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.CORNERTAG, true, i))
break;
Element pCornerNode = pBorderNode.getElement(XFA.CORNERTAG, false,
i, false, false);
// set the thickness
pCornerNode.setAttribute(new StringAttr(XFA.THICKNESS, sString),
XFA.THICKNESSTAG);
}
}
/**
* Set the edit value for this field.
*
* @param sValue
* the formatted value for this field.
* @exception UnsupportedOperationException
* if you try and set the value of a boilerplate content. The
* given edit value will be either
*
* - in the field's edit picture representation, or,
*
- in the field's short locale picture representation,
* or,
*
- in any representation for works-in-progress.
*
* If the given edit value can be successfully unformatted
* into its canonical representation, then the field's raw
* value is set to this canonical representation. Otherwise
* the field's raw value is set to the given edit value.
*/
void setEditValue(String sValue) {
// Adobe patent application tracking # B136, entitled "Applying locale
// behaviors to regions of a form", inventors: Gavin McKenzie, Mike
// Tardif, John Brinkman"
// Use the edit picture format to untransform
// the given edit value , then set it as the
// raw value.
// Take care not to force empty string as rawvalue
// when the edit value does not untransform wrt to
// the edit picture clause. In such cases we just set the
// raw value.
StringHolder sCanonical = new StringHolder();
Element poUI = getElement(XFA.UITAG, true, 0, false, false);
Picture poEditPicture = null;
if (poUI != null)
poEditPicture = (Picture) poUI.getElement(XFA.PICTURETAG, true, 0,
false, false);
if (poEditPicture != null) {
String sLocale = getInstalledLocale();
sCanonical.value = poEditPicture.unformatString(sValue, sLocale, null);
}
else if (!StringUtils.isEmpty(sValue)) {
Element poValueNode = getElement(XFA.VALUETAG, true, 0, true, false);
Element poContentNode = null;
if (poValueNode != null)
poContentNode = (Element) poValueNode.getOneOfChild(false, true);
//
// If this is a time, date, datetime, float or decimal
// field w/o edit pictures, Then try to parse the
// raw value using the locale's short formats.
//
if (poContentNode != null) {
//
// Avoid fetching the locale and constructing the LcData
// object until absolutely needed.
//
if (poContentNode.isSameClass(XFA.DATETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateFormat(LcData.SHORT_FMT);
PictureFmt.parseDate(sValue, sPict, sLocale, sCanonical, true);
} else if (poContentNode.isSameClass(XFA.TIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getTimeFormat(LcData.SHORT_FMT);
PictureFmt.parseTime(sValue, sPict, sLocale, sCanonical, true);
} else if (poContentNode.isSameClass(XFA.DATETIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateTimeFormat(LcData.SHORT_FMT, LcData.WITHOUT_CATEGORIES);
String sDatePict = oData.getDateFormat(LcData.SHORT_FMT);
String sTimePict = oData.getTimeFormat(LcData.SHORT_FMT);
PictureFmt.parseDateTime(sValue, sPict, sDatePict, sTimePict, sLocale, sCanonical, true);
} else if (poContentNode.isSameClass(XFA.INTEGERTAG)) {
//
// Try to parse the given locale-sensitive value according
// to the field's locale integral format w/o grouping
// symbols.
//
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
int nOptn = LcData.WITHOUT_GROUPINGS;
String sPict = oData.getNumberFormat(LcData.INTEGRAL_FMT, nOptn);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
} else if (poContentNode.isSameClass(XFA.FLOATTAG)) {
//
// Try to parse the given locale-sensitive value according
// to the field's locale decimal format w/o grouping symbols
// to the given value's width and precision.
//
String sLocale = getInstalledLocale();
int nOptn = LcData.WITHOUT_GROUPINGS;
LcData oData = new LcData(sLocale);
int nPrec = oData.getNumberPrecision(sValue);
if (nPrec == 0) {
//
// Fix for Watson 1233947. Request that the radix
// symbol be included in the numeric picture.
//
if (sValue.contains(oData.getRadixSymbol()))
nOptn |= LcData.WITH_RADIX;
}
nOptn |= LcData.withPrecision(nPrec | 0x80);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT,
nOptn);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
} else if (poContentNode.isSameClass(XFA.DECIMALTAG)) {
//
// Try to parse the given locale-sensitive value according
// to the field's locale decimal format w/o grouping symbols
// to the field's value width and precision. If the field's
// fracDigits attribute is -1, then use picture 8 symbols.
//
Int oFrac = (Int) poContentNode.getAttribute(XFA.FRACDIGITSTAG);
String sLocale = getInstalledLocale();
int nOptn = LcData.WITHOUT_GROUPINGS;
if (oFrac.getValue() == -1)
nOptn |= LcData.WITH_EIGHTS;
LcData oData = new LcData(sLocale);
int nPrec = oData.getNumberPrecision(sValue);
if (nPrec == 0) {
//
// Fix for Watson 1233947. Request that the radix
// symbol be included in the numeric picture.
//
if (sValue.contains(oData.getRadixSymbol()))
nOptn |= LcData.WITH_RADIX;
}
nOptn |= LcData.withPrecision(nPrec | 0x80);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT, nOptn);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
}
}
}
//
// If successful Then let the canonical result be the new raw value.
//
if (!StringUtils.isEmpty(sCanonical.value))
setRawValue(sCanonical.value);
else
setRawValue(sValue);
}
void setForeColor(String sString) {
Element pFontNode = getElement(XFA.FONTTAG, false, 0, false, false);
Element pFillNode = pFontNode.getElement(XFA.FILLTAG, false, 0, false,
false);
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, false, 0,
false, false);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString),
XFA.VALUETAG);
}
/**
* Set the formatted value for this field.
*
* @param sValue
* the formatted value for this field.
* @exception UnsupportedOperationException
* if you try and set the value of a boilerplate content. The
* given formatted value should be in the either
*
* - in the field's format picture representation, or,
*
- in the field's default locale picture representation,
* or,
*
- in any representation for works-in-progress.
*
* If the given formatted value can be successfully
* unformatted into its canonical representation, then the
* field's raw value is set to this canonical representation.
* Otherwise the field's raw value is set to the given
* formatted value.
* @exclude from public api.
*/
public void setFormattedValue(String sValue) {
// Adobe patent application tracking # B136, entitled "Applying locale
// behaviors to regions of a form", inventors: Gavin McKenzie, Mike
// Tardif, John Brinkman"
StringHolder sCanonical = new StringHolder();
if (isPropertySpecified(XFA.FORMATTAG, true, 0)) {
// check for any picture formatting
Element poFormatNode = getElement(XFA.FORMATTAG, true, 0, false,
false);
if (poFormatNode != null) {
Picture pPictureNode = (Picture) poFormatNode.getElement(
XFA.PICTURETAG, true, 0, false, false);
if (pPictureNode != null) {
String sLocale = getInstalledLocale();
sCanonical.value = pPictureNode.unformatString(sValue, sLocale, null);
}
}
}
else if (!StringUtils.isEmpty(sValue)) {
Element poValueNode = getElement(XFA.VALUETAG, true, 0, true, false);
Element poContentNode = null;
if (poValueNode != null)
poContentNode = (Element) poValueNode.getOneOfChild(false, true);
//
// If this is a time, date, datetime, float or decimal
// field w/o format pictures, Then try to parse the
// raw value using the locale's default formats.
//
if (poContentNode != null) {
//
// Avoid fetching the locale and constructing the LcData
// object until absolutely needed.
//
if (poContentNode.isSameClass(XFA.DATETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateFormat(LcData.MED_FMT);
PictureFmt.parseDate(sValue, sPict, sLocale, sCanonical, true);
}
else if (poContentNode.isSameClass(XFA.TIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getTimeFormat(LcData.MED_FMT);
PictureFmt.parseTime(sValue, sPict, sLocale, sCanonical, true);
}
else if (poContentNode.isSameClass(XFA.DATETIMETAG)) {
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getDateTimeFormat(LcData.MED_FMT, LcData.WITHOUT_CATEGORIES);
String sDatePict = oData.getDateFormat(LcData.MED_FMT);
String sTimePict = oData.getTimeFormat(LcData.MED_FMT);
PictureFmt.parseDateTime(sValue, sPict, sDatePict,
sTimePict, sLocale, sCanonical, true);
}
else if (poContentNode.isSameClass(XFA.INTEGERTAG)) {
//
// Try to parse the given locale-sensitive value according
// to
// the field's locale integral format w/ grouping symbols.
//
String sLocale = getInstalledLocale();
LcData oData = new LcData(sLocale);
String sPict = oData.getNumberFormat(LcData.INTEGRAL_FMT, LcData.WITH_GROUPINGS);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
}
else if (poContentNode.isSameClass(XFA.FLOATTAG)) {
//
// Try to parse the given locale-sensitive value according
// to
// the field's locale decimal format w/ grouping symbols
// to the given value's width and precision.
//
String sLocale = getInstalledLocale();
int nOptn = LcData.WITH_GROUPINGS;
LcData oData = new LcData(sLocale);
int nPrec = oData.getNumberPrecision(sValue);
nOptn |= LcData.withPrecision(nPrec | 0x80);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT, nOptn);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
}
else if (poContentNode.isSameClass(XFA.DECIMALTAG)) {
//
// Try to parse the given locale-sensitive value according
// to
// the field's locale decimal format w/ grouping symbols
// to the given value's width and precision. If the field's
// fracDigits attribute is -1, then use picture 8 symbols.
//
Int oFrac = (Int) poContentNode.getAttribute(XFA.FRACDIGITSTAG);
String sLocale = getInstalledLocale();
int nOptn = LcData.WITH_GROUPINGS;
if (oFrac.getValue() == -1)
nOptn |= LcData.WITH_EIGHTS;
LcData oData = new LcData(sLocale);
int nPrec = oData.getNumberPrecision(sValue);
nOptn |= LcData.withPrecision(nPrec | 0x80);
int nWidth = sValue.length();
if (nWidth > 0)
nOptn |= LcData.withWidth(nWidth);
String sPict = oData.getNumberFormat(LcData.DECIMAL_FMT, nOptn);
PictureFmt.parseNumeric(sValue, sPict, sLocale, sCanonical);
}
}
}
//
// If successful Then let the canonical result be the new raw value.
//
if (!StringUtils.isEmpty(sCanonical.value))
setRawValue(sCanonical.value);
else
setRawValue(sValue);
}
/**
* Set this node to contain a null value.
*
* @param bNull
* true if the node contains a null value, false otherwise.
* @exclude from public api.
*/
public void setIsNull(boolean bNull, boolean bNotify/* = true */) {
// watson bug 1378947
// we can skip the work only if the node doesn't have a proto and the
// element is not present
if (bNull && !hasProto() && !isPropertySpecified(XFA.VALUETAG, true, 0))
return;
Element poValue = getElement(XFA.VALUETAG, false, 0, false, false);
// check for one of child first;
Node poNullCheck = poValue.getOneOfChild(true, false);
if (bNull && (poNullCheck == null))
return;
Content poValueContent = (Content) poValue.getOneOfChild();
// content doesn't need to notify
poValueContent.setIsNull(bNull, bNotify, false);
}
void setItemState(int nIndex, boolean bSelected) {
// Set the selection state of the item at the specified index.
// Get the bound value at the selected index.
String sBoundItem = getBoundItem(nIndex);
if (StringUtils.isEmpty(sBoundItem))
return;
// get the value
Element pValueNode = getElement(XFA.VALUETAG, true, 0, false, false);
// get the value's content
if (pValueNode != null) {
Element pContentNode = (Content) pValueNode.getOneOfChild(true,
false);
if (pContentNode == null) {
return;
}
// Single-select listbox
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
String sValue = ((TextValue) pContentNode).getValue();
if (bSelected) {
// Select the value if it's not currently selected.
if (!sBoundItem.equals(sValue))
((TextValue) pContentNode).setValue(sBoundItem);
} else {
// De-select the value if it is the currently selected
// value.
if (sBoundItem.equals(sValue))
((TextValue) pContentNode).setIsNull(true, true, false);
}
}
// Multi-select listbox
else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
// Check for a match with a multi-select listbox value.
boolean bAddItem = bSelected; // Add item if we're told to
// 'select' it.
List oValues = new ArrayList();
ExDataValue oExData = (ExDataValue) pContentNode;
oExData.getValues(oValues);
for (int i = oValues.size(); i > 0; i--) {
if (sBoundItem.equals(oValues.get(i - 1))) {
if (bSelected) {
bAddItem = false; // If the item's already
// selected, don't add it again.
break;
} else {
oValues.remove(i - 1); // Remove item if it's
// currently selected.
oExData.setValue(oValues); // Update form with new
// list.
break;
}
}
}
// Add item if necessary.
if (bAddItem) {
oValues.add(sBoundItem);
oExData.setValue(oValues); // Update form with new list.
}
}
}
}
void setMandatory(String sString) {
Element pValidateNode = getElement(XFA.VALIDATETAG, false, 0, false,
false);
pValidateNode.setAttribute(new StringAttr(XFA.NULLTEST, sString),
XFA.NULLTESTTAG);
}
void setMessage(String sMessage, String aType) {
Element pValidateNode = getElement(XFA.VALIDATETAG, false, 0, false,
false);
TemplateModel.setValidationMessage(pValidateNode, sMessage, aType);
}
/**
* Set whether or not this container is 'on' in its ExclGroup
*
* @param bOn true if this container is 'on', false if the is not 'on'.
*
* @exclude from public api.
*/
public void setOn(boolean bOn) {
String sValue = getOnValue();
if (bOn && sValue != null)
setRawValue(sValue);
if (!bOn) {
sValue = getOffValue();
if (sValue != null)
setRawValue(sValue);
else
setIsNull(true, true);
}
}
/**
* Set the raw value for this field, no formatting.
*
* @param sString
* the unformatted raw value for this field.
* @exception UnsupportedOperationException
* if you try and set the value of a boilerplate content. The
* given unformatted value should be in the either
*
* - in the field's canonical representation, or,
*
- in any representation for works-in-progress.
*
*
* @exclude from public api.
*/
public void setRawValue(String sString) {
Node pContentNode = null;
// get the value
Element pValueNode = getElement(XFA.VALUETAG, false, 0, false, false);
// get the value's content
if (pValueNode != null) {
pContentNode = pValueNode.getOneOfChild();
}
if (pContentNode != null) {
if (pContentNode.isSameClass(XFA.TEXTTAG)) {
((TextValue) pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.EXDATATAG)) {
((ExDataValue) pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.DATETAG)) {
((DateValue) pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.DATETIMETAG)) {
((DateTimeValue) pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.TIMETAG)) {
((TimeValue) pContentNode).setValue(sString);
} else if (pContentNode.isSameClass(XFA.INTEGERTAG)) {
((IntegerValue) pContentNode).setValue(sString, false);
} else if (pContentNode.isSameClass(XFA.FLOATTAG)) {
((FloatValue) pContentNode).setValue(sString, false, true, false);
} else if (pContentNode.isSameClass(XFA.DECIMALTAG)) {
((DecimalValue) pContentNode).setValue(sString, false, true, false);
} else if (pContentNode.isSameClass(XFA.IMAGETAG)) {
((ImageValue) pContentNode).setValue(sString, "");
// JEY TODO: really, no string support for XFA:BOOLEANTAG?
} else {
// "%1 is an unsupported operation for the %2 object%3"
// Note: %3 is extra text if necessary
MsgFormatPos oMessage = new MsgFormatPos(
ResId.UnsupportedOperationException);
oMessage.format("setRawValue");
oMessage.format(pContentNode.getClassAtom());
throw new ExFull(oMessage);
}
}
}
void setSelectedIndex(int nIndex) {
// Value of -1 will clear the list of selected items.
if (nIndex == -1) {
setIsNull(true, true);
}
else {
// Set the item at the specified index to be the only one that is
// selected.
String sBoundItem = getBoundItem(nIndex);
if (!StringUtils.isEmpty(sBoundItem))
setRawValue(sBoundItem);
}
}
/**
* For performance we don't normally copy over the arrays for check buttons and
* lists. This method copies them when needed.
*/
private void lazyResolveItemsArrays(boolean bCreateDefaults) {
// Watson bug 1333106 ensure we copy over all the items when we call get nodes
// we do this because in Version 7 getNodes() would return the items
ProtoableNode poNode = (ProtoableNode) getElement(XFA.ITEMSTAG, true, 0, bCreateDefaults, false);
if (poNode != null) {
// we have an instance on the proto, so lets copy it over
// Mark the dom document as loading to ensure that the nodes
// are not marked as dirty.
final boolean previousWillDirty = getWillDirty();
setWillDirty(false);
try {
// copy over the items
if (poNode.getXFAParent() != this)
poNode.createProto(this, false);
// look for a second instance of items
poNode = (ProtoableNode) getElement(XFA.ITEMSTAG, true, 1, bCreateDefaults, false);
// copy over the second items
if (poNode != null && poNode.getXFAParent() != this)
poNode.createProto(this, false);
}
finally {
setWillDirty(previousWillDirty);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy