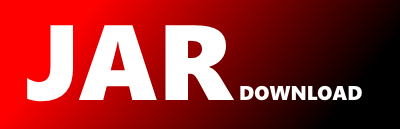
com.adobe.xfa.template.containers.PageArea Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.containers;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.EnumValue;
import com.adobe.xfa.Measurement;
import com.adobe.xfa.Node;
import com.adobe.xfa.NodeList;
import com.adobe.xfa.TagnameFilter;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.UnitSpan;
/**
* Functionality represented by the XFA element.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class PageArea extends Container {
Measurement mZeroDefaultUnit;
public PageArea(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.PAGEAREA, XFA.PAGEAREA, null,
XFA.PAGEAREATAG, XFA.PAGEAREA);
mZeroDefaultUnit = null;
}
public Attribute defaultAttribute(int eTag) {
if (eTag == XFA.WTAG || eTag == XFA.HTAG) {
if (mZeroDefaultUnit == null) {
mZeroDefaultUnit = new Measurement("", "0");
}
return mZeroDefaultUnit;
}
// Not special case, so let the base class handle it
return super.defaultAttribute(eTag);
}
/**
* Get the number of contentArea children.
*
* @return the number of contentArea children
*/
public int getContentAreaCount() {
int nCount = 0;
NodeList oChildren = getNodes();
for (int i = 0; i < oChildren.length(); i++) {
if (oChildren.item(i) instanceof ContentArea)
nCount++;
}
return nCount;
}
/**
* Return the height of the contentArea at given index
*
* @param nCA -
* the zero based index of contentArea
* @return the height as specified in contentArea
*/
public UnitSpan getContentAreaHeight(int nCA) {
ContentArea oCA = getContentAreaNode(nCA);
if (oCA != null)
return oCA.getHeight();
else
return UnitSpan.ZERO;
}
/**
* Get the contentArea child given an index.
*
* @param nCA -
* zero based index of child to return
* @return ContentAreanode at given index. A null node is returned if index
* is out of range.
*/
public ContentArea getContentAreaNode(int nCA) {
int nCount = 0;
NodeList oChildren = getNodes();
for (int i = 0; i < oChildren.length(); i++) {
if (oChildren.item(i) instanceof ContentArea) {
if (nCount == nCA)
return (ContentArea) oChildren.item(i);
nCount++;
}
}
// not found
return null;
}
/**
* Returns a list of all the contentArea child nodes.
*
* @return list of contentArea child nodes
*/
public List getContentAreaNodes() {
TagnameFilter filter = new TagnameFilter(XFA.CONTENTAREATAG);
List list = filter.filterNodes(this, 1);
return list;
}
/**
* Return the width of the contentArea at given index
*
* @param nCA -
* the zero based index of contentArea
* @return the width as specified in contentArea
*/
public UnitSpan getContentAreaWidth(int nCA) {
ContentArea oCA = getContentAreaNode(nCA);
if (oCA != null)
return oCA.getWidth();
else
return UnitSpan.ZERO;
}
/**
* Get the height of a pageArea
*
* @return the value of the 'h' attribute
*/
public UnitSpan getHeight() {
Element poMedium = getMedium();
if (poMedium != null) {
Measurement oMeas;
int eOrientation = ((EnumValue) poMedium
.getAttribute(XFA.ORIENTATIONTAG)).getInt();
if (eOrientation == EnumAttr.LANDSCAPE) {
oMeas = (Measurement) poMedium.getAttribute(XFA.SHORTTAG);
} else {
oMeas = (Measurement) poMedium.getAttribute(XFA.LONGTAG);
}
return oMeas.getUnitSpan();
}
return UnitSpan.ZERO;
}
Element getMedium() {
return getElement(XFA.MEDIUMTAG, true, 0, false, false);
}
/**
* Return the next contentArea specified given a contentArea
*
*/
public ContentArea getNextContentAreaNode(ContentArea oCA) {
boolean bNext = false;
NodeList oChildren = getNodes();
for (int i = 0; i < oChildren.length(); i++) {
if (bNext && oChildren.item(i) instanceof ContentArea) {
return (ContentArea) oChildren.item(i);
} else if (oCA == oChildren.item(i)) {
bNext = true;
}
}
// not found
return null;
}
/**
* Get the orientation of a pageArea
*
* @return the value of the 'orientation' attribute of the medium element,
* defaults to Portrait.
*/
public EnumAttr getOrientation() {
int eOrientation = EnumAttr.PORTRAIT;
Element poMedium = getMedium();
if (poMedium != null)
eOrientation = ((EnumValue) poMedium
.getAttribute(XFA.ORIENTATIONTAG)).getInt();
return EnumAttr.getEnum(eOrientation);
}
/**
* Access the page content nodes - subforms,fields,draws,areas.
*
* @return a list of the page content nodes
*/
public List getPageContentNodes() {
// Return areas, draws, fields, subforms, exclGroups associated with the page
// (if any)
List nodeList = new ArrayList();
TagnameFilter filter = new TagnameFilter();
filter.setTagname(XFA.AREATAG);
filter.filterNodes(this, 1, nodeList);
filter.setTagname(XFA.DRAWTAG);
filter.filterNodes(this, 1, nodeList);
filter.setTagname(XFA.FIELDTAG);
filter.filterNodes(this, 1, nodeList);
filter.setTagname(XFA.SUBFORMTAG);
filter.filterNodes(this, 1, nodeList);
filter.setTagname(XFA.EXCLGROUPTAG);
filter.filterNodes(this, 1, nodeList);
return nodeList;
}
/**
* Get the width of a pageArea
*
* @return the value of the 'w' attribute
*/
public UnitSpan getWidth() {
Element poMedium = getMedium();
if (poMedium != null) {
Measurement oMeas;
int eOrientation = ((EnumValue) poMedium
.getAttribute(XFA.ORIENTATIONTAG)).getInt();
if (eOrientation == EnumAttr.LANDSCAPE) {
oMeas = (Measurement) poMedium.getAttribute(XFA.LONGTAG);
} else {
oMeas = (Measurement) poMedium.getAttribute(XFA.SHORTTAG);
}
return oMeas.getUnitSpan();
}
return UnitSpan.ZERO;
}
/**
* Return TRUE if this pageArea has a medium that is compatible with the
* given pageArea's medium. PageArea have compatible mediums if
* they can represent different surfaces of the same sheet.
* @param pPageArea the page area to compare with
* @return true if compatible
* @exclude
*/
boolean hasCompatibleMedium(PageArea pPageArea) {
//Compare stock, short and long attributes
if(null != pPageArea) {
Element poMedium1 = getMedium();
Element poMedium2 = pPageArea.getMedium();
if(null != poMedium1 && null != poMedium2) {
// Check stock
String s1 = poMedium1.getAttribute(XFA.STOCKTAG).toString();
String s2 = poMedium2.getAttribute(XFA.STOCKTAG).toString();
if (s1.equals(s2)) {
// Check short
Measurement oM1 = (Measurement)poMedium1.getAttribute(XFA.SHORTTAG);
Measurement oM2 = (Measurement)poMedium2.getAttribute(XFA.SHORTTAG);
if (oM1.getUnitSpan() == oM2.getUnitSpan()) {
// Check long
oM1 = (Measurement)poMedium1.getAttribute(XFA.LONGTAG);
oM2 = (Measurement)poMedium2.getAttribute(XFA.LONGTAG);
if (oM1.getUnitSpan() == oM2.getUnitSpan()) {
return true;
}
}
}
}
}
return false;
}
/**
* Query whether the pageArea has any content children.
*
* @return true if it does and false otherwise.
*/
public boolean hasPageContent() {
return (0 < getPageContentNodes().size());
}
public boolean isHeightGrowSupported() {
return false;
}
public boolean isWidthGrowSupported() {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy