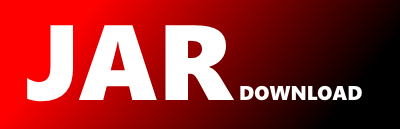
com.adobe.xfa.template.containers.Subform Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.containers;
import com.adobe.xfa.Arg;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.DependencyTracker;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.Obj;
import com.adobe.xfa.Schema;
import com.adobe.xfa.ScriptDynamicPropObj;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.XFA;
import com.adobe.xfa.template.InstanceManager;
import com.adobe.xfa.template.TemplateModel;
import com.adobe.xfa.data.DataNode; // Javaport: TODO this violated packaging hierarchy.
/**
* A class to represent the XFA subform
object.
* A subform describes a container capable of enclosing other containers.
*/
public class Subform extends Container {
/**
* index Used
overflowLeader[ | ]
overflowTtrailer[ | ]
* bookendLeader[ | ]
bookendTrailer[ | ]
*/
//int[][] mppnUsedTable;
/**
* Instantiates a subform container.
* @param parent the field's parent, if any.
* @param prevSibling the field's previous sibling, if any.
*/
public Subform(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.SUBFORM, XFA.SUBFORM, null,
XFA.SUBFORMTAG, XFA.SUBFORM);
//mppnUsedTable = null;
}
/**
* @exclude from public api.
*/
public Obj getInstanceManager() {
// create a new instance manager
return new InstanceManager(this);
}
/**
* @exclude from public api.
*/
public DataNode getDataNode() {
// Form method only
return null;
}
/**
* @exclude from public api.
*/
public void execEvent(String sActivity) {
// overridden by XFAFormSubform
}
/**
* @exclude from public api.
*/
public boolean execValidate() {
// overridden by XFAFormSubform
return true;
}
/**
* @exclude from published api.
*/
public Attribute getAttribute(int eTag, boolean bPeek, boolean bValidate) {
Attribute oProperty = super.getAttribute(eTag, bPeek, bValidate);
if (eTag == XFA.LOCALETAG && !bPeek) {
if (oProperty != null && !oProperty.isEmpty())
return oProperty;
//
// Check ancestors for the locale attribute,
//
String sLocale = getInstalledLocale();
return newAttribute(eTag, sLocale);
}
return oProperty;
}
String getBackColor() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pFillNode = pBorderNode.getElement(XFA.FILLTAG, true, 0,
true, false);
if (pFillNode != null) {
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, true,
0, true, false);
if (pColorNode != null)
sRet = pColorNode.getAttribute(XFA.VALUETAG).toString();
}
}
return sRet;
}
String getBorderColor() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, true, 0,
true, false);
if (pEdgeNode != null) {
Element pColorNode = pEdgeNode.getElement(XFA.COLORTAG, true,
0, true, false);
if (pColorNode != null)
sRet = pColorNode.getAttribute(XFA.VALUETAG).toString();
}
}
return sRet;
}
String getBorderWidth() {
String sRet = "";
Element pBorderNode = getElement(XFA.BORDERTAG, true, 0, true, false);
if (pBorderNode != null) {
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, true, 0,
true, false);
if (pEdgeNode != null)
sRet = pEdgeNode.getAttribute(XFA.THICKNESSTAG).toString();
}
return sRet;
}
/**
* @exclude from public api.
*/
protected int getInstanceIndex(DependencyTracker pDepTrack) {
// Form method only
return 0;
}
String getMessage() {
String sRet = "";
Element pValidateNode = getElement(XFA.VALIDATETAG, true, 0, false,
false);
if (pValidateNode != null)
sRet = TemplateModel.getValidationMessage(pValidateNode,
XFA.SCRIPTTEST);
return sRet;
}
/**
* @see Container#isConnectSupported()
* @exclude
*/
public boolean isConnectSupported() {
return true;
}
/**
* @exclude from published api.
*/
public boolean isHeightGrowSupported() {
return true;
}
/**
* @exclude from published api.
*/
public boolean isWidthGrowSupported() {
return true;
}
/**
* @exclude from public api.
*/
public void reset() {
//mppnUsedTable = null;
}
void setBackColor(String sString) {
Element pBorderNode = getElement(XFA.BORDERTAG, 0);
Element pFillNode = pBorderNode.getElement(XFA.FILLTAG, 0);
Element pColorNode = pFillNode.getElement(XFA.COLORTAG, 0);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString), XFA.VALUETAG);
}
void setBorderColor(String sString) {
Element pBorderNode = getElement(XFA.BORDERTAG, 0);
// need to set the 4 edges
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.EDGETAG, true, i))
break;
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, false, i,
false, false);
// set the color
Element pColorNode = pEdgeNode.getElement(XFA.COLORTAG, 0);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString), XFA.VALUETAG);
}
// need to set the 4 corners
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.CORNERTAG, true, i))
break;
Element pCornerNode = pBorderNode.getElement(XFA.CORNERTAG, false,
i, false, false);
// set the color
Element pColorNode = pCornerNode.getElement(XFA.COLORTAG, 0);
pColorNode.setAttribute(new StringAttr(XFA.VALUE, sString), XFA.VALUETAG);
}
}
void setBorderWidth(String sString) {
// TODO change back to getElement once prot changes are in;
Element pBorderNode = getElement(XFA.BORDERTAG, 0);
// need to set the 4 edges
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.EDGETAG, true, i))
break;
Element pEdgeNode = pBorderNode.getElement(XFA.EDGETAG, false, i,
false, false);
// set the thickness
pEdgeNode.setAttribute(new StringAttr(XFA.THICKNESS, sString), XFA.THICKNESSTAG);
}
// need to set the 4 corners
for (int i = 0; i < 4; i++) {
if (!pBorderNode.isPropertySpecified(XFA.CORNERTAG, true, i))
break;
Element pCornerNode = pBorderNode.getElement(XFA.CORNERTAG, false,
i, false, false);
// set the thickness
pCornerNode.setAttribute(new StringAttr(XFA.THICKNESS, sString), XFA.THICKNESSTAG);
}
}
/**
* set the instance index
* @param nIndex the index to set to
* @exclude
*/
public void setInstanceIndex(int nIndex) {
// Form method only
}
void setMessage(String sString) {
Element pValidateNode = getElement(XFA.VALIDATETAG, 0);
TemplateModel.setValidationMessage(pValidateNode, sString,
XFA.SCRIPTTEST);
}
/**
* @exclude from published api.
*/
public ScriptTable getScriptTable() {
return SubformScript.getScriptTable();
}
/**
* @exclude from published api.
*/
protected ScriptDynamicPropObj getDynamicScriptProp(String sPropertyName,
boolean bPropertyOverride, boolean bPeek) {
// if the first char of sPropertyName is '_' check for a same name
// subform or subformset child. If we find one return the instance manager.
if (sPropertyName.length() > 0 && sPropertyName.charAt(0) == '_') {
String sNewName = sPropertyName.substring(1);
if (sNewName.length() > 0) {
Node oChild = locateChildByName(sNewName, 0);
if ((oChild != null) &&
((oChild instanceof Subform) ||
(oChild instanceof SubformSet))) {
return getInstanceManagerScriptObj;
}
}
}
return super.getDynamicScriptProp(sPropertyName, bPropertyOverride, bPeek);
}
private final static ScriptDynamicPropObj getInstanceManagerScriptObj =
new ScriptDynamicPropObj(Schema.XFAVERSION_21, Schema.XFAAVAILABILITY_ALL) {
public boolean invokeGetProp(Obj scriptThis, Arg retValue, String sPropertyName) {
return Subform.getInstanceManagerFunc(scriptThis, retValue, sPropertyName);
}
};
private static boolean getInstanceManagerFunc(Obj oObj, Arg oRetVal, String sProp) {
Subform oSubform = (Subform)oObj;
oRetVal.setObject(oSubform.getInstanceManager());
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy