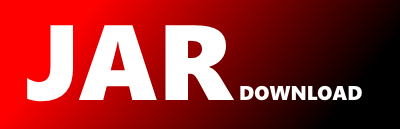
com.adobe.xfa.template.formatting.Picture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.template.formatting;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.TextNode;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.BooleanHolder;
import com.adobe.xfa.ut.Peer;
import com.adobe.xfa.ut.PictureFmt;
import com.adobe.xfa.ut.StringHolder;
import com.adobe.xfa.ut.StringUtils;
/**
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class Picture extends ProtoableNode {
public Picture(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.PICTURE, XFA.PICTURE, null,
XFA.PICTURETAG, XFA.PICTURE);
}
/**
* formatString formats a data string using the defined picture formatting
* mask for current context.
*
* @param sSource -
* The data string to be formatted
* @param sLocale
* The name of the locale
* @param sResult -
* the StringBuilder to be formatted.
*
* @return true if successfully formatted, false otherwise.
*/
public boolean formatString(String sSource,
String sLocale/* = LcLocale.getLocale() */, StringBuilder sResult) {
String sMask = "";
TextNode oTextNode = getText(true, false, false);
if (oTextNode != null)
sMask = oTextNode.getValue();
// watson bug 1729758, if there is no picture clause, return the original value and success.
if (StringUtils.isEmpty(sMask))
return true;
//
// Get field's value content.
//
Element poParent = getXFAParent();
while (poParent != null) {
if (poParent.isSameClass(XFA.FIELDTAG))
break;
poParent = poParent.getXFAParent();
}
Element poValueNode = null;
if (poParent != null)
poValueNode = poParent.getElement(XFA.VALUETAG, true, 0, true,
false);
Node poContentNode = null;
if (poValueNode != null)
poContentNode = poValueNode.getOneOfChild(false, true);
//
// Text and numeric picture share a common symbol namespace,
// and PictureFmt will give precedence to numeric pictures.
// So if it's a text-valued node then try formatting as a text
// picture first.
//
if (poContentNode != null && poContentNode.isSameClass(XFA.TEXTTAG)
&& PictureFmt.isTextPicture(sMask)) {
return PictureFmt.formatText(sSource, sMask, sLocale, sResult);
}
PictureFmt oPict = new PictureFmt(sLocale);
return oPict.format(sSource, sMask, sResult);
}
/**
* Get the text stored in this element
*
* @return the text string
*/
public String getValue() {
String sValue = "";
TextNode oTextNode = getText(true, false, false);
if (oTextNode != null) {
sValue = oTextNode.getValue();
}
return sValue;
}
public void setNumericAttributes() {
}
/**
* Set the text value within this element
*
* @param sText
* a string to set/replace the text stored in this element
*/
public void setValue(String sText) {
if (sText == null)
return;
TextNode oTextNode = getText(false, false, false);
oTextNode.setValue(sText,true,false);
// update the field with the new picture clause
notifyPeers(Peer.VALUE_CHANGED, "", this);
}
/**
* unformatString unformats a data string using the defined picture
* formatting mask for current context.
*
* @param sSource
* The data string to be unformatted
* @param sLocale
* The locale in which to perform the unformatting
* @param pbSuccess
* The result of the unformatting operation
*
* @return true if successfully parsed, false otherwise.
*/
public String unformatString(String sSource,
String sLocale/* = LcLocale.getLocale() */, BooleanHolder pbSuccess) {
String sMask = "";
TextNode oTextNode = getText(true, false, false);
if (oTextNode != null)
sMask = oTextNode.getValue();
//
// Get field's text content.
//
Element poParent = getXFAParent();
while (poParent != null) {
if (poParent.isSameClass(XFA.FIELDTAG))
break;
poParent = poParent.getXFAParent();
}
Element poValueNode = null;
if (poParent != null)
poValueNode = poParent.getElement(XFA.VALUETAG, true, 0, true,
false);
Node poContentNode = null;
if (poValueNode != null)
poContentNode = poValueNode.getOneOfChild(false, true);
//
// Text and numeric picture share a common symbol namespace,
// and PictureFmt will give precedence to numeric pictures.
// So if it's a text-valued node then try parsing as a text
// picture first.
//
if (poContentNode != null && poContentNode.isSameClass(XFA.TEXTTAG)
&& PictureFmt.isTextPicture(sMask)) {
StringHolder sResult = new StringHolder();
boolean bSuccess = PictureFmt.parseText(sSource, sMask, sLocale, sResult);
if (pbSuccess != null)
pbSuccess.value = bSuccess;
return sResult.value;
}
PictureFmt oPict = new PictureFmt(sLocale);
return oPict.parse(sSource, sMask, pbSuccess);
}
/**
* @exclude from published api.
*/
public ScriptTable getScriptTable() {
return PictureScript.getScriptTable();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy