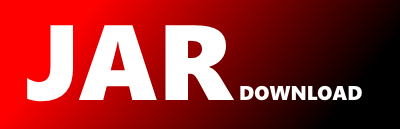
com.adobe.xfa.template.sequencing.Traverse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.sequencing;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.XFA;
import com.adobe.xfa.template.TemplateModel;
/**
* This class implements the functionality behind the XFA element.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class Traverse extends ProtoableNode {
public Traverse(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.TRAVERSE, XFA.TRAVERSE, null,
XFA.TRAVERSETAG, XFA.TRAVERSE);
}
// is a [0..n] child of but doesn't have a name attribute
// which can cause some difficulty when merging properties from a proto
// (they get overridden in the order they appear regardless of the operation specified).
// Here we are overloading the getPrivateName method to treat the operation attribute
// as the private name (used for proto resolution) so overrides will only occur on
// elements representing the same operation.
// This change is being introduced to accommodate a change in the XFATraversalService
// where it will now support operation="next" as well as operation="first" on
// elements which in turn was added to accommodate tab order handling of fragments
// where the fragment contains a "first" and the host document contains a "next" and
// both are needed to properly traverse the form.
public String getPrivateName() {
Attribute oOperation = null;
// Watson 1738361: Check proto if there is one.
if (getProto() != null)
oOperation = getProto().getAttribute(XFA.OPERATIONTAG);
if (oOperation == null)
oOperation = getAttribute(XFA.OPERATIONTAG);
if (oOperation == null)
return defaultAttribute(XFA.OPERATIONTAG).toString();
else
return oOperation.getAttrValue();
}
// Here we are overriding the getName/setName methods to return the value of
// the operation attribute as per the XFATraverseSOMAccessProposal.
// The change in behavior is disabled if the v2.7-scripting legacy flag
// is on, where we would simply defer to the base class.
public String getName() {
if (getAppModel().getLegacySetting(AppModel.XFA_LEGACY_V27_SCRIPTING))
return super.getName();
else
return getPrivateName();
}
public void setPrivateName(String sNewName) {
setAttribute(new StringAttr(XFA.OPERATION, sNewName), XFA.OPERATIONTAG);
}
public void setName(String sNewName) {
boolean bLegacyScripting = true;
AppModel oAppModel = getAppModel();
TemplateModel oModel = TemplateModel.getTemplateModel(oAppModel, false);
if (oModel != null)
bLegacyScripting = oModel.getLegacySetting(AppModel.XFA_LEGACY_V27_SCRIPTING);
if (bLegacyScripting)
super.setName(sNewName);
else
setPrivateName(sNewName);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy