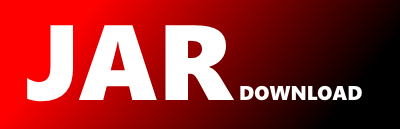
com.adobe.xfa.text.DispChange Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
//----------------------------------------------------------------------
//
// File: textdcha.h
//
// Description
// Public header file for class DispChange
//
// This is actually a private Text Service class used by the
// text display to track changes made to the stream
// (hierarchy). The display can then use the information here
// to decide whether it can optimize its regeneration.
//
// Change history
// Project Release Date Who Review What
// HawaiiRd 5.0 960222 RD Created.
//
//----------------------------------------------------------------------
package com.adobe.xfa.text;
/**
* @exclude from published api -- Mike Tardif, May 2006.
*/
class DispChange {
static final int CHANGE_NONE = 0;
static final int CHANGE_INSERT = 1;
static final int CHANGE_DELETE = 2;
static final int CHANGE_FRAME = 3;
static final int CHANGE_OTHER = 4;
static final int CHANGE_TO_END = 5;
static final int CHANGE_FULL = 6;
static final int CHANGE_FULL_FORCE_FRAMES = 7;
private TextStream mpoStream;
private int mnIndex;
private int mnCount;
private int meType;
private boolean mbJustify;
DispChange () {
reset();
}
final int type () {
return meType;
}
final TextStream stream () {
return mpoStream;
}
final int index () {
return mnIndex;
}
final int count () {
return mnCount;
}
final boolean justify () {
return mbJustify;
}
final void insert (TextStream poStream, int nIndex, int nCount) {
simpleChange (CHANGE_INSERT, poStream, nIndex, nCount);
}
final void delete (TextStream poStream, int nIndex, int nCount) {
simpleChange (CHANGE_DELETE, poStream, nIndex, nCount);
}
final void frame (TextStream poStream, int nIndex, int nCount) {
simpleChange (CHANGE_FRAME, poStream, nIndex, nCount);
}
final void other (TextStream poStream, int nIndex, int nCount) {
if (meType == CHANGE_NONE) {
meType = CHANGE_OTHER;
mpoStream = poStream;
mnIndex = nIndex;
mnCount = nCount;
}
else if ((meType != CHANGE_FULL) && (meType != CHANGE_TO_END) && (poStream == mpoStream)) {
meType = CHANGE_OTHER;
int nOldEnd = mnIndex + mnCount;
int nNewEnd = nIndex + nCount;
if (nIndex < mnIndex) {
mnIndex = nIndex;
}
if (nNewEnd < nOldEnd) {
nNewEnd = nOldEnd;
}
mnCount = nNewEnd - mnIndex;
}
else {
Full (meType == CHANGE_TO_END);
}
}
final void other (TextStream poStream, int nIndex) {
other (poStream, nIndex, 0);
}
final void toEnd (TextStream poStream, int nIndex) {
if (meType != CHANGE_NONE) {
other (poStream, nIndex);
}
else if (meType == CHANGE_TO_END) {
if (poStream == mpoStream) {
if (nIndex < mnIndex) {
mnIndex = nIndex;
}
}
else {
Full (true); // TBD: it would be nice if we could figure out which change came first
}
}
else {
meType = CHANGE_TO_END;
mpoStream = poStream;
mnIndex = nIndex;
mnCount = 0;
}
}
final void setJustify () {
mbJustify = true;
}
final void Full (boolean bForceAllFrames) {
meType = bForceAllFrames ? CHANGE_FULL_FORCE_FRAMES : CHANGE_FULL;
}
final void full () {
Full (false);
}
final void reset () {
meType = CHANGE_NONE;
mpoStream = null;
mnIndex = 0;
mnCount = 0;
mbJustify = false;
}
private final void simpleChange (int eType, TextStream poStream, int nIndex, int nCount) {
if (meType != CHANGE_NONE) {
other (poStream, nIndex);
}
else {
meType = eType;
mpoStream = poStream;
mnIndex = nIndex;
mnCount = nCount;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy