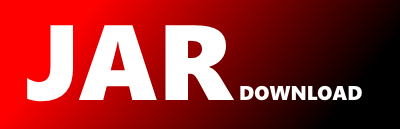
com.adobe.xfa.text.MultiMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.text;
import com.adobe.xfa.gfx.GFXMapping;
import com.adobe.xfa.gfx.GFXMappingList;
/**
* @exclude from published api.
*/
class MultiMapper {
static final int GLYPH_CAN_ADD = 0;
static final int GLYPH_FLUSH_FIRST = 1;
static final int GLYPH_NOT_MULTIPLE = 2;
private boolean mbAccumulating;
private int mnCharIndex;
private int mnGlyphFirst;
private int mnGlyphNext;
MultiMapper () {
}
MultiMapper (MultiMapper source) {
mbAccumulating = source.mbAccumulating;
mnCharIndex = source.mnCharIndex;
mnGlyphFirst = source.mnGlyphFirst;
mnGlyphNext = source.mnGlyphNext;
}
boolean isAccumulating () {
return mbAccumulating;
}
int canAccumulate (GFXMapping oMapping, int nGlyphOffset) {
if (oMapping.getCharCount() != 1) {
return GLYPH_NOT_MULTIPLE;
}
if (oMapping.getGlyphCount() == 0) {
return GLYPH_NOT_MULTIPLE;
}
MultiMapper oTester = new MultiMapper (this);
int nCharIndex = oMapping.getCharIndex (0);
for (int i = 0; i < oMapping.getGlyphCount(); i++) {
int nGlyphIndex = oMapping.getGlyphIndex (i) + nGlyphOffset;
if (! oTester.canAccumulate (nCharIndex, nGlyphIndex)) {
if (i == 0) {
return GLYPH_FLUSH_FIRST;
} else {
return GLYPH_NOT_MULTIPLE;
}
}
oTester.accumulate (nCharIndex, nGlyphIndex);
}
return GLYPH_CAN_ADD;
}
boolean canAccumulate (int nCharIndex, int nGlyphIndex) {
if (! mbAccumulating) {
return true;
}
if (nCharIndex != mnCharIndex) {
return false;
}
return (nGlyphIndex == mnGlyphNext)
|| ((nGlyphIndex + 1) == mnGlyphFirst);
}
int getCharIndex () {
return mnCharIndex;
}
int getGlyphIndex () {
return mnGlyphFirst;
}
int getGlyphLength () {
return mnGlyphNext - mnGlyphFirst;
}
void accumulate (GFXMapping oMapping, int nGlyphOffset) {
assert (oMapping.getCharCount() == 1);
assert (oMapping.getGlyphCount() != 0);
int nCharIndex = oMapping.getCharIndex (0);
for (int i = 0; i < oMapping.getGlyphCount(); i++) {
accumulate (nCharIndex, oMapping.getGlyphIndex (i) + nGlyphOffset);
}
}
void accumulate (int nCharIndex, int nGlyphIndex) {
if (! mbAccumulating) {
mnCharIndex = nCharIndex;
mnGlyphFirst = nGlyphIndex;
mnGlyphNext = nGlyphIndex + 1;
mbAccumulating = true;
} else {
assert (nCharIndex == mnCharIndex);
if (nGlyphIndex == mnGlyphNext) {
mnGlyphNext++;
} else {
assert ((nGlyphIndex + 1) == mnGlyphFirst);
mnGlyphFirst--;
}
}
}
void flush (GFXMappingList oMappingList) {
if (! mbAccumulating) {
return;
}
oMappingList.addMapping (mnCharIndex, mnGlyphFirst, 1, mnGlyphNext - mnGlyphFirst);
mbAccumulating = false;
}
void reset () {
mbAccumulating = false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy