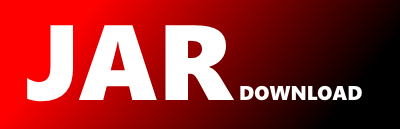
com.adobe.xfa.text.TextBreakFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.text;
import com.adobe.xfa.ut.LcLocale;
/**
* @exclude from published api.
*/
public abstract class TextBreakFinder {
public abstract void setBreakCandidates (int pcText[], int peBreakData[], boolean pbCandidates[], boolean bLegacyPositioning);
abstract public String getCurrentLocale ();
public static TextBreakFinder recycle (LcLocale poLocale, TextBreakFinder poOld) {
// Need to recycle if switching between dictionary-based and normal.
// Following initializations will help in that process.
TextBreakFinder poResult = null;
String pcOldName = null;
if (poOld != null) {
pcOldName = poOld.getCurrentLocale();
}
// If we need dictionary-based breaking, we may have an appropriate break
// finder already in poOld, if it has a non-NULL locale name and that
// matches the new name. Otherwise, we'll have to create a new one.
// Note that we don't delete the old right away. It may have come from
// the same dynamic library and we can avoid an implicit unload and
// reload. Wrap the library-based finder in our own object which holds a
// reference to the library.
// if (poLocale.needsDictionaryBreaking()) { // TODO: how to replace the C++ implementation
// String pcNewName = poLocale.getName();
//
// if ((pcOldName == null) || (! pcOldName.equals (pcNewName) != 0)) {
// DynamicLibrary poLibrary = DynamicLibrary.new (gsLibraryName, true);
// if (poLibrary == null) {
// JfExThrow (TEXT_ERR_DICT_BREAK);
// }
//
// ExDeleter oDeleteLibrary (poLibrary);
//
// BreakFinderProc pfnCreate;
// DynamicLibraryGetFunc (poLibrary, gsEntryName, pfnCreate, true);
//
// if (pfnCreate != null) {
// BreakFinder poDelegate = (pfnCreate) (pcNewName);
// if (poDelegate != null) {
// ExDeleter oDeleteDelegate (poDelegate);
// poResult = new TextLibraryBreakFinder (poLibrary, poDelegate);
// oDeleteDelegate.detach();
// oDeleteLibrary.detach();
// }
// }
// }
// }
// Non-dictionary-based finder: We need to create a new one only if the
// old one had a real locale name (was dictionary-based).
// else {
if (pcOldName != null) {
poResult = new DefaultBreakFinder();
}
// }
// Now we can finish up. If we've created a new one, get rid of the old
// (it may be NULL). Otherwise, if there is an old one, simply use it
// again. Otherwise, create a default one.
if (poResult == null) {
if (poOld != null) {
poResult = poOld;
} else {
poResult = new DefaultBreakFinder();
}
}
return poResult;
}
}
class DefaultBreakFinder extends TextBreakFinder {
public void setBreakCandidates (int pcText[], int peBreakData[], boolean pbCandidates[], boolean bLegacyPositioning) {
assert (peBreakData.length == pcText.length);
assert (pbCandidates.length >= pcText.length); // TODO: shouldn't length be passed in?
int nChars = pcText.length;
if (nChars == 0)
return;
int i;
for (i = 0; i < nChars; i++)
pbCandidates[i] = false;
int cPrev = pcText[0];
int ePrevNonSpace = peBreakData[0];
int nSpaces = 0;
for (i = 1; i < nChars; i++)
{
int c = pcText[i];
int eNextData = peBreakData[i];
if (TextCharProp.getBreakClass (eNextData) == TextCharProp.BREAK_SP) {
nSpaces++;
}
else {
int eRule = TextCharProp.getPairRule (ePrevNonSpace, eNextData);
switch (eRule) {
case TextCharProp.BREAK_PROHIBIT:
break;
case TextCharProp.BREAK_IF_SPACE:
if (nSpaces > 0) {
pbCandidates[i] = true;
}
break;
case TextCharProp.BREAK_COMBINING_MARK:
if (nSpaces > 0) {
pbCandidates[i - 1] = true;
}
break;
case TextCharProp.BREAK_ALLOW:
if (TextCharProp.kinsokuAllowEnd (cPrev) && TextCharProp.kinsokuAllowStart (c)) {
if (bLegacyPositioning) {
if ((nSpaces > 0) || ((cPrev != '.') && (cPrev != ',') && (cPrev != '-') && (cPrev != '/'))) {
pbCandidates[i] = true;
}
} else {
pbCandidates[i] = true;
}
}
break;
}
nSpaces = 0;
ePrevNonSpace = eNextData;
cPrev = c;
}
}
}
public String getCurrentLocale () {
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy