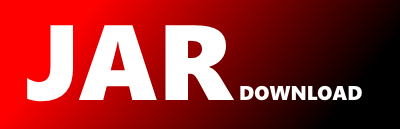
com.adobe.xfa.text.TextGfxAttr Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.text;
import com.adobe.xfa.gfx.GFXAttr;
import com.adobe.xfa.gfx.GFXColour;
import com.adobe.xfa.gfx.GFXGraphicContext;
import com.adobe.xfa.gfx.GFXTextAttr;
import com.adobe.xfa.gfx.GFXTextContext;
/**
* Describe graphic attributes that apply to text.
*
* The text graphic attribute object can represent any possible
* combination of graphic attributes that apply to text (e.g.,
* foreground colour, underline). This object is conceptually similar
* to the graphic attribute object for text (GfxTextAttr), except that
* this object can represent a sparse set of attributes.
*
*
* Each graphic attribute calue can be set or queried independently.
* Additionally, each attribute can be flagged as enabled or disabled.
* When enabled, the corresponding attribute value has meaning. When
* disabled, the value can be thought of as unknown or irrelevant.
*
*
* This object has a large number of accessors, which appear in groups
* of four, one such group for each attribute. For example, consider
* the colour attribute. There are two overloads each of Colour() and
* ColourEnable(). In each overload pair, one sets the value and one
* retrieves it. In other words, one Colour() overload returns the
* current colour value and one sets it, and one ColourEnable() overload
* returns the enabled state and one sets it. Enabled flags are all
* Boolean, with TRUE indicating the value is enabled. Setting an
* attribute value in a TextGfxAttr object (e.g., by second Colour()
* overload) automatically enables it. Enabling a value (e.g., by
* second ColourEnable() overload) that has never been set usually sets
* it to a default value. In rare cases, the uninitialized value may
* be left disabled.
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public class TextGfxAttr {
private final GFXTextAttr moGfxTextAttr = new GFXTextAttr();
private boolean mbColourEnable;
private boolean mbColourBgEnable;
private boolean mbShadeEnable;
private boolean mbShadeScaleEnable;
private boolean mbGraphicContextEnable;
private boolean mbTextContextEnable;
/**
* Default constructor.
*
* Populates the text graphic attribute object with all attributes
* disabled.
*/
public TextGfxAttr () {
gfxTextAttrInitialize();
setGfxTextAttrDefault (false);
}
/**
* Copy constructor.
*
* Copies all attributes and their enabled/disabled status.
* @param oSource - Source attribute object to copy.
*/
public TextGfxAttr (TextGfxAttr oSource) {
gfxTextAttrInitialize();
setGfxTextAttrDefault (false);
copyFrom (oSource);
}
/**
* Query the collected graphic text attributes
* @return - The collected graphic text attributes (GfxTextAttr)
* represented in this object.
*/
public GFXTextAttr getGfxTextAttr () {
return moGfxTextAttr;
}
/**
* Query whether the graphic text attributes are complete
* @return TRUE if all graphic text attributes are enabled; FALSE if any
* isn't.
*/
public boolean gfxTextAttrEnable () {
return underlineEnable()
&& overlineEnable()
&& strikeoutEnable()
&& textContextEnable()
&& graphicContextEnable()
&& colourEnable()
&& colourBgEnable()
&& styleEnable()
&& shadeEnable()
&& shadeScaleEnable();
}
/**
* Query whether any graphic text attribute is enabled.
* @return TRUE if any graphic text attribute is enabled; FALSE if all
* are disabled.
*/
public boolean anyGfxEnable () {
return underlineEnable()
|| overlineEnable()
|| strikeoutEnable()
|| textContextEnable()
|| graphicContextEnable()
|| colourEnable()
|| colourBgEnable()
|| styleEnable()
|| shadeEnable()
|| shadeScaleEnable();
}
/**
* Return the underline value if enabled
* @return Underline value.
*/
public int underline () {
return moGfxTextAttr.underline();
}
/**
* Set and enable the underline value
* @param eNewUnderline - New underline value.
*/
public void underline (int eNewUnderline) {
if (eNewUnderline != underline()) {
moGfxTextAttr.underline (eNewUnderline);
onUpdateGfxTextAttr();
}
}
/**
* Query whether the underline value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean underlineEnable () {
return moGfxTextAttr.underline() != GFXTextAttr.UNDER_UNKNOWN;
}
/**
* Enable/disable the underline value
* @param bNewEnable - TRUE if the underline value is to be enabled;
* FALSE if it is to be disabled.
*/
public void underlineEnable (boolean bNewEnable) {
if (bNewEnable != underlineEnable()) {
moGfxTextAttr.underline (bNewEnable ? GFXTextAttr.UNDER_NONE : GFXTextAttr.UNDER_UNKNOWN);
onUpdateGfxTextAttr();
}
}
/**
* Return the overline value if enabled
* @return Overline value.
*/
public int overline () {
return moGfxTextAttr.overline();
}
/**
* Set and enable the overline value
* @param eNewOverline - New overline value.
*/
public void overline (int eNewOverline) {
if (eNewOverline != overline()) {
moGfxTextAttr.overline (eNewOverline);
onUpdateGfxTextAttr();
}
}
/**
* Query whether the overline value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean overlineEnable () {
return moGfxTextAttr.overline() != GFXTextAttr.OVER_UNKNOWN;
}
/**
* Enable/disable the overline value
* @param bNewEnable - TRUE if the overline value is to be enabled;
* FALSE if it is to be disabled.
*/
public void overlineEnable (boolean bNewEnable) {
if (bNewEnable != overlineEnable()) {
moGfxTextAttr.overline (bNewEnable ? GFXTextAttr.OVER_NONE : GFXTextAttr.OVER_UNKNOWN);
onUpdateGfxTextAttr();
}
}
/**
* Return the strikeout value if enabled
* @return Strikeout value.
*/
public int strikeout () {
return moGfxTextAttr.strikeout();
}
/**
* Set and enable the strikeout value
* @param eNewStrikeout - New strikeout value.
*/
public void strikeout (int eNewStrikeout) {
if (eNewStrikeout != strikeout()) {
moGfxTextAttr.strikeout (eNewStrikeout);
onUpdateGfxTextAttr();
}
}
/**
* Query whether the strikeout value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean strikeoutEnable () {
return moGfxTextAttr.strikeout() != GFXTextAttr.STRIKEOUT_UNKNOWN;
}
/**
* Enable/disable the strikeout value
* @param bNewEnable - TRUE if the strikeout value is to be enabled;
* FALSE if it is to be disabled.
*/
public void strikeoutEnable (boolean bNewEnable) {
if (bNewEnable != strikeoutEnable()) {
moGfxTextAttr.strikeout (bNewEnable ? GFXTextAttr.STRIKEOUT_NONE : GFXTextAttr.STRIKEOUT_UNKNOWN);
onUpdateGfxTextAttr();
}
}
/**
* Return the graphic text context associated with this text attribute
* object.
* @return Pointer to the graphic text context. A null pointer is
* returned if the context has never been set.
*/
public GFXTextContext textContext () {
return mbTextContextEnable ? moGfxTextAttr.textContext() : null;
}
/**
* Set a new graphic text context for this text attribute object.
* @param poTextContext - Pointer to new graphic text context to
* associate with this text attribute object.
*/
public void textContext (GFXTextContext poTextContext) {
if ((poTextContext != moGfxTextAttr.textContext()) || (! mbTextContextEnable)) {
moGfxTextAttr.textContext (poTextContext);
mbTextContextEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the graphic text context attribute is enabled.
* @return True if the graphic text context is enabled; false if not.
* Note that it can be enabled but have a null value.
*/
public boolean textContextEnable () {
return mbTextContextEnable;
}
/**
* Enable/disable graphic text context for this text attribute object.
* @param bNewEnable - True if the graphic text context is to be
* enabled; false if it is to be disabled.
*/
public void textContextEnable (boolean bNewEnable) {
if (bNewEnable != mbTextContextEnable) {
mbTextContextEnable = bNewEnable;
if (! mbTextContextEnable) {
moGfxTextAttr.textContext (null);
}
onUpdateGfxTextAttr();
}
}
/**
* Return the graphic context associated with this text attribute
* object.
* @return Pointer to the graphic context. A null pointer is returned
* if the context has never been set.
*/
public GFXGraphicContext graphicContext () {
return mbGraphicContextEnable ? moGfxTextAttr.graphicContext() : null;
}
/**
* Set a new graphic context for this text attribute object.
* @param poGraphicContext - Pointer to new graphic context to associate
* with this text attribute object.
*/
public void graphicContext (GFXGraphicContext poGraphicContext) {
if ((poGraphicContext != moGfxTextAttr.graphicContext()) || (! mbGraphicContextEnable)) {
moGfxTextAttr.graphicContext (poGraphicContext);
mbGraphicContextEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the graphic context attribute is enabled.
* @return True if the graphic context is enabled; false if not. Note
* that it can be enabled but have a null value.
*/
public boolean graphicContextEnable () {
return mbGraphicContextEnable;
}
/**
* Enable/disable graphic context for this text attribute object.
* @param bNewEnable - True if the graphic context is to be enabled;
* false if it is to be disabled.
*/
public void graphicContextEnable (boolean bNewEnable) {
if (bNewEnable != mbGraphicContextEnable) {
mbGraphicContextEnable = bNewEnable;
if (! mbGraphicContextEnable) {
moGfxTextAttr.graphicContext (null);
}
onUpdateGfxTextAttr();
}
}
/**
* Return the foreground colour value if enabled
* @return Foreground colour value.
*/
public GFXColour colour () {
return moGfxTextAttr.colour();
}
/**
* Set and enable the foreground colour value
* @param oNewColour - New foreground colour value.
*/
public void colour (GFXColour oNewColour) {
if ((! moGfxTextAttr.colour().equals (oNewColour)) || (! mbColourEnable)) {
moGfxTextAttr.colour (oNewColour);
mbColourEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the foreground colour value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean colourEnable () {
return mbColourEnable;
}
/**
* Enable/disable the foreground colour value
* @param bNewEnable - TRUE if the colour value is to be enabled; FALSE
* if it is to be disabled.
*/
public void colourEnable (boolean bNewEnable) {
if (bNewEnable != mbColourEnable) {
mbColourEnable = bNewEnable;
onUpdateGfxTextAttr();
}
}
/**
* Return the background colour value if enabled
* @return Background colour value.
*/
public GFXColour colourBg () {
return moGfxTextAttr.colourBg();
}
/**
* Set and enable the background colour value
* @param oNewColourBg - New background colour value.
*/
public void colourBg (GFXColour oNewColourBg) {
if ((! moGfxTextAttr.colourBg().equals (oNewColourBg)) || (! mbColourBgEnable)) {
moGfxTextAttr.colourBg (oNewColourBg);
mbColourBgEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the background colour value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean colourBgEnable () {
return mbColourBgEnable;
}
/**
* Enable/disable the background colour value
* @param bNewEnable - TRUE if the background colour value is to be
* enabled; FALSE if it is to be disabled.
*/
public void colourBgEnable (boolean bNewEnable) {
if (bNewEnable != mbColourBgEnable) {
mbColourBgEnable = bNewEnable;
onUpdateGfxTextAttr();
}
}
/**
* Return the style value if enabled
* @return Style value.
*/
public int style () {
return moGfxTextAttr.style();
}
/**
* Set and enable the style value
* @param eNewStyle - New style value.
*/
public void style (int eNewStyle) {
if (eNewStyle != style()) {
moGfxTextAttr.style (eNewStyle);
onUpdateGfxTextAttr();
}
}
/**
* Query whether the style value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean styleEnable () {
return moGfxTextAttr.style() != GFXAttr.STYLE_UNKNOWN;
}
/**
* Enable/disable the style value
* @param bNewEnable - TRUE if the style value is to be enabled; FALSE
* if it is to be disabled.
*/
public void styleEnable (boolean bNewEnable) {
if (bNewEnable != styleEnable()) {
moGfxTextAttr.style (bNewEnable ? GFXTextAttr.STYLE_SOLID : GFXTextAttr.STYLE_UNKNOWN);
onUpdateGfxTextAttr();
}
}
/**
* Return the shade level if enabled
* @return Shade level.
*/
public int shade () {
return mbShadeEnable ? moGfxTextAttr.shade() : -1;
}
/**
* Set and enable the shade level
* @param lNewShade - New shade level.
*/
public void shade (int lNewShade) {
if ((lNewShade != shade()) || (! mbShadeEnable)) {
moGfxTextAttr.shade (lNewShade);
mbShadeEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the shade level is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean shadeEnable () {
return mbShadeEnable;
}
/**
* Enable/disable the shade level
* @param bNewEnable - TRUE if the shade level is to be enabled; FALSE
* if it is to be disabled.
*/
public void shadeEnable (boolean bNewEnable) {
if (bNewEnable != shadeEnable()) {
mbShadeEnable = bNewEnable;
onUpdateGfxTextAttr();
}
}
/**
* Return the shade scale value if enabled
* @return Shade scale value.
*/
public int shadeScale () {
return mbShadeScaleEnable ? moGfxTextAttr.shadeScale() : -1;
}
/**
* Set and enable the shade scale value
* @param lNewShadeScale - New shade scale value.
*/
public void shadeScale (int lNewShadeScale) {
if ((lNewShadeScale != shadeScale()) || (! mbShadeScaleEnable)) {
moGfxTextAttr.shadeScale (lNewShadeScale);
mbShadeScaleEnable = true;
onUpdateGfxTextAttr();
}
}
/**
* Query whether the shade scale value is enabled
* @return TRUE if enabled; FALSE if not.
*/
public boolean shadeScaleEnable () {
return mbShadeScaleEnable;
}
/**
* Enable/disable the shade scale value
* @param bNewEnable - TRUE if the shade scale value is to be enabled;
* FALSE if it is to be disabled.
*/
public void shadeScaleEnable (boolean bNewEnable) {
if (bNewEnable != shadeScaleEnable()) {
mbShadeScaleEnable = bNewEnable;
onUpdateGfxTextAttr();
}
}
/**
* Fill the text graphic attribute holder with default values.
*
* This method behaves like a Clear() or Reset() method might. It
* replaces all values in the text attribute object with defaults.
* @param bDefault - FALSE if all attributes are to be disabled; TRUE if
* they are to be enabled with default values.
*/
public void setGfxTextAttrDefault (boolean bDefault) {
if (bDefault) {
moGfxTextAttr.underline (GFXTextAttr.UNDER_NONE);
moGfxTextAttr.overline (GFXTextAttr.OVER_NONE);
moGfxTextAttr.strikeout (GFXTextAttr.STRIKEOUT_NONE);
moGfxTextAttr.textContext (null);
moGfxTextAttr.graphicContext (null);
moGfxTextAttr.colour (GFXColour.black());
moGfxTextAttr.colourBg (GFXColour.white());
moGfxTextAttr.style (GFXAttr.STYLE_SOLID);
moGfxTextAttr.shade (100);
moGfxTextAttr.shadeScale (100);
mbTextContextEnable = true;
mbGraphicContextEnable = true;
mbColourEnable = true;
mbColourBgEnable = true;
mbShadeEnable = true;
mbShadeScaleEnable = true;
onUpdateGfxTextAttr();
}
else {
underlineEnable (false);
overlineEnable (false);
strikeoutEnable (false);
textContextEnable (false);
graphicContextEnable (false);
colourEnable (false);
colourBgEnable (false);
styleEnable (false);
shadeEnable (false);
shadeScaleEnable (false);
}
}
/**
* Replace all attributes with those from the source object.
*
* The standard assignment copies everything, including enabled and
* disabled status.
* @param oSource - Source attribute object to copy.
*/
public void copyFrom (TextGfxAttr oSource) {
if (this != oSource) {
moGfxTextAttr.copyFrom (oSource.moGfxTextAttr);
mbTextContextEnable = oSource.mbTextContextEnable;
mbGraphicContextEnable = oSource.mbGraphicContextEnable;
mbColourEnable = oSource.mbColourEnable;
mbColourBgEnable = oSource.mbColourBgEnable;
mbShadeEnable = oSource.mbShadeEnable;
mbShadeScaleEnable = oSource.mbShadeScaleEnable;
}
}
/**
* Equality comparison operator.
*
* Compares on an attribute by attribute basis. Two attributes are
* considered equal if they are both disabled, or they are both enabled
* and their values compare for equality.
* @param object - Text attribute object to compare against.
* @return TRUE if the text attribute objects are considered equal;
* FALSE otherwise.
*/
public boolean equals (Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
TextGfxAttr cmp = (TextGfxAttr) object;
if (underlineEnable() != cmp.underlineEnable()) {
return false;
}
if (overlineEnable() != cmp.overlineEnable()) {
return false;
}
if (strikeoutEnable() != cmp.strikeoutEnable()) {
return false;
}
if (textContextEnable() != cmp.textContextEnable()) {
return false;
}
if (graphicContextEnable() != cmp.graphicContextEnable()) {
return false;
}
if (colourEnable() != cmp.colourEnable()) {
return false;
}
if (colourBgEnable() != cmp.colourBgEnable()) {
return false;
}
if (styleEnable() != cmp.styleEnable()) {
return false;
}
if (shadeEnable() != cmp.shadeEnable()) {
return false;
}
if (shadeScaleEnable() != cmp.shadeScaleEnable()) {
return false;
}
if (underlineEnable()) {
if (underline() != cmp.underline()) {
return false;
}
}
if (overlineEnable()) {
if (overline() != cmp.overline()) {
return false;
}
}
if (strikeoutEnable()) {
if (strikeout() != cmp.strikeout()) {
return false;
}
}
if (textContextEnable()) {
if (textContext() != cmp.textContext()) {
return false;
}
}
if (graphicContextEnable()) {
if (graphicContext() != cmp.graphicContext()) {
return false;
}
}
if (colourEnable()) {
if (colour() != cmp.colour()) {
return false;
}
}
if (colourBgEnable()) {
if (colourBg() != cmp.colourBg()) {
return false;
}
}
if (styleEnable()) {
if (style() != cmp.style()) {
return false;
}
}
if (shadeEnable()) {
if (shade() != cmp.shade()) {
return false;
}
}
if (shadeScaleEnable()) {
if (shadeScale() != cmp.shadeScale()) {
return false;
}
}
return true;
}
public int hashCode() {
int hash = 89;
if (underlineEnable()) {
hash = (hash * 31) ^ underline();
}
if (overlineEnable()) {
hash = (hash * 31) ^ overline();
}
if (strikeoutEnable()) {
hash = (hash * 31) ^ strikeout();
}
if (textContextEnable()) {
hash = (hash * 31) ^ textContext().hashCode();
}
if (graphicContextEnable()) {
hash = (hash * 31) ^ graphicContext().hashCode();
}
if (colourEnable()) {
hash = (hash * 31) ^ colour().hashCode();
}
if (colourBgEnable()) {
hash = (hash * 31) ^ colourBg().hashCode();
}
if (styleEnable()) {
hash = (hash * 31) ^ style();
}
if (shadeEnable()) {
hash = (hash * 31) ^ shade();
}
if (shadeScaleEnable()) {
hash = (hash * 31) ^ shadeScale();
}
return hash;
}
/**
* Inequality comparison operator.
*
* Compares on an attribute by attribute basis. Two attributes are
* considered unequal if their enabled/disabled settings don't match, or
* if they are both enabled and their values are unequal.
* @param oCompare - Text attribute object to compare against.
* @return TRUE if the text attribute objects are considered not equal;
* FALSE otherwise.
*/
public boolean notEqual (TextGfxAttr oCompare) {
return ! equals (oCompare);
}
//proprietary
void applyGfxTextAttrs (TextGfxAttr oSource) {
boolean bAnyChange = false;
if (oSource.underlineEnable()) {
moGfxTextAttr.underline (oSource.underline());
bAnyChange = true;
}
if (oSource.overlineEnable()) {
moGfxTextAttr.overline (oSource.overline());
bAnyChange = true;
}
if (oSource.strikeoutEnable()) {
moGfxTextAttr.strikeout (oSource.strikeout());
bAnyChange = true;
}
if (oSource.textContextEnable()) {
moGfxTextAttr.textContext (oSource.textContext());
mbTextContextEnable = true;
bAnyChange = true;
}
if (oSource.graphicContextEnable()) {
moGfxTextAttr.graphicContext (oSource.graphicContext());
mbGraphicContextEnable = true;
bAnyChange = true;
}
if (oSource.colourEnable()) {
moGfxTextAttr.colour (oSource.colour());
mbColourEnable = true;
bAnyChange = true;
}
if (oSource.colourBgEnable()) {
moGfxTextAttr.colourBg (oSource.colourBg());
mbColourBgEnable = true;
bAnyChange = true;
}
if (oSource.styleEnable()) {
moGfxTextAttr.style (oSource.style());
bAnyChange = true;
}
if (oSource.shadeEnable()) {
moGfxTextAttr.shade (oSource.shade());
mbShadeEnable = true;
bAnyChange = true;
}
if (oSource.shadeScaleEnable()) {
moGfxTextAttr.shadeScale (oSource.shadeScale());
mbShadeScaleEnable = true;
bAnyChange = true;
}
if (bAnyChange) {
onUpdateGfxTextAttr();
}
}
protected void setGfxTextAttr (GFXTextAttr oGfxTextAttr) {
moGfxTextAttr.copyFrom (oGfxTextAttr);
mbTextContextEnable = true;
mbGraphicContextEnable = true;
mbColourEnable = true;
mbColourBgEnable = true;
mbShadeEnable = true;
mbShadeScaleEnable = true;
}
protected void onUpdateGfxTextAttr () {
}
private void gfxTextAttrInitialize () {
mbTextContextEnable = false;
mbGraphicContextEnable = false;
mbColourEnable = false;
mbColourBgEnable = false;
mbShadeEnable = false;
mbShadeScaleEnable = false;
}
}