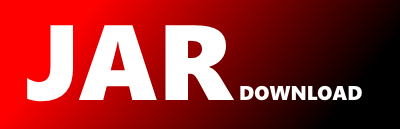
com.adobe.xfa.text.TextStreamIterator Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.text;
/**
*
* Implementation of TextCharPropIterator for iterating over text stream
* content.
*
*
* This class allows the caller to do grapheme cluster and word break
* analysis over text stream content. Used in conjunction with a {@link
* TextBreakIterator}, the caller can find those key break points in
* any text stream.
*
*
* The text break iterator returns index numbers of grapheme cluster or
* word break points in the text. When used with a text stream
* iterator, these are actual index numbers in the text and can be used
* to populate text position (classes {@link TextPosnBase} and {@link
* TextPosn}), range (class {@link TextRange}) and editor (class
* {TODO: link TextEditor}).
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public class TextStreamIterator implements TextCharPropIterator {
private final TextPosnBase moPosition;
private final boolean mbForward;
private final boolean mbVisual;
private final int mnStartIndex;
/**
* Construct a stream iterator from stream pointer and index.
*
* This overload take stream position and initial index value and
* populates the stream iterator with them.
*
* @param poStream - Text stream to iterate over. Must not be null.
* @param nIndex - Item index number within the stream to initializate
* the iterator. If it is out of bounds, it will be truncated to the
* end of the stream. Note that iteration need not start at the start
* of the text stream.
* @param bForward - True (default) for forward iteration over the
* stream; false for backward iteration.
* @param bVisual - True if movements are to occur visually over
* bidirectional text; false (default) for logical positioning.
*/
public TextStreamIterator (TextStream poStream, int nIndex, boolean bForward, boolean bVisual) {
moPosition = new TextPosnBase (poStream, nIndex);
mbForward = bForward;
mbVisual = bVisual;
mnStartIndex = nIndex;
}
/**
* Construct a stream iterator from an existing position.
* @param oSourcePosn - Initial position to start iteration at. Need
* not be the start of the stream, but must be properly associated with
* a stream.
* @param bForward - True (default) for forward iteration over the
* stream; false for backward iteration.
* @param bVisual - True if movements are to occur visually over
* bidirectional text; false (default) for logical positioning.
*/
public TextStreamIterator (TextPosnBase oSourcePosn, boolean bForward, boolean bVisual) {
moPosition = new TextPosnBase (oSourcePosn);
mbForward = bForward;
mbVisual = bVisual;
mnStartIndex = oSourcePosn.index();
}
/**
* Return the index at the start of the iteration.
*
* This method is not normally called by the creator of the stream
* iterator. Instead, it is used by {@link TextBreakIterator} to
* obtain character property data in a polymorphic manner. For more
* information, please see the base class, {@link TextCharPropIterator}.
*
* @return First element's index value.
*/
public int first () {
return mnStartIndex;
}
/**
* Advance or back up one position.
*
* This method is not normally called by the creator of the stream
* iterator. Instead, it is used by {@link TextBreakIterator} to
* obtain character property data in a polymorphic manner. For more
* information, please see the base class, {@link TextCharPropIterator}.
*
* @param nIndex - Current index value.
* @return True if there were character properties at the given index
* value; false if past the logical end of the array.
*/
public int next (int nIndex) {
moPosition.index (nIndex);
return next();
}
public int next () {
int c = moPosition.charMove (mbForward, mbVisual);
return (c == '\0') ? TextCharProp.INVALID
: TextCharProp.getCharProperty (c);
}
public int getNextIndex () {
return moPosition.index();
}
}