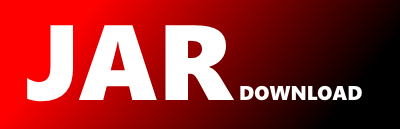
com.adobe.xfa.text.Units Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.text;
import com.adobe.xfa.ut.UnitSpan;
/**
* Unit conversions for AXTE.
*
* This class handles the functions of the c++ implementation's
* jfTextDispUnit and jfTextDispUnitSpan classes. It provides unit and
* type conversions for AXTE.
*
*
* AXTE works in three different unit types:
*
*
* -
* jfUnitSpan - A measurement fully qualified by unit type
*
* -
* float - Representing a value measured in points (class jfTextDispUnit
* in the C++ implementation)
*
* -
* int - Representing a value measured in points/1000 (class
* jfTextDispUnitSpan in the C++ implementation)
*
*
*
* This class provides a number of static methods for converting units
* based on the conventions listed above.
*
* @exclude from published api.
*/
public final class Units { // made public only for test framework
/**
* Convert points/1000 to points.
* @param pt1000 Integer value representing a value in thousandths of a
* point.
* @return Floating point value representing number of points.
*/
static float toFloat (int pt1000) {
return ((float) pt1000) / 1000.0f;
}
/**
* Convert UnitSpan to points.
* @param us UnitSpan value representing a fully-qualified measurement.
* @return Floating point value representing number of points.
*/
static float toFloat (UnitSpan us) {
return toFloat (toInt (us));
}
/**
* Convert points to points/1000.
* @param pts Floating point value representing number of points.
* @return Integer value representing a value in thousandths of a point.
*/
static int toInt (float pts) {
return Math.round (pts * 1000.0f);
}
/**
* Convert UnitSpan to points/1000.
* @param us UnitSpan value representing a fully-qualified measurement.
* @return Integer value representing a value in thousandths of a point.
*/
static int toInt (UnitSpan us) {
return UnitSpan.convertUnit (UnitSpan.POINTS_1K, us.units(), us.value());
}
/**
* Convert points/1000 to UnitSpan.
* @param pt1000 Integer value representing a value in thousandths of a
* point.
* @return UnitSpan value representing a fully-qualified measurement.
* The given point/1000 value is assumed to represent a font
* measurement. Therefore, the unit type will be UnitSpan.PICA_PT_1K.
*/
static UnitSpan toUnitSpan (int pt1000) {
return toUnitSpan (UnitSpan.POINTS_1K, pt1000);
}
/**
* Convert points to UnitSpan.
* @param pts Floating point value representing number of points.
* @return UnitSpan value representing a fully-qualified measurement.
* The given point value is assumed to represent a line measurement.
* Therefore, the unit type will be UnitSpan.INCHES_72K.
*/
static UnitSpan toUnitSpan (float pts) {
return toUnitSpan (UnitSpan.INCHES_72K, toInt (pts));
}
/**
* Convert points to UnitSpan, rounding up. This method guarantees that
* the generated UnitSpan will not be smaller than the given point
* value.
* @param f Floating point value representing number of points.
* @return UnitSpan value representing a fully-qualified measurement.
* The given point value is assumed to represent a line measurement.
* Therefore, the unit type will be UnitSpan.INCHES_72K.
*/
static UnitSpan forceUnitSpan (float f) {
return new UnitSpan (UnitSpan.INCHES_72K, forceInt (f));
}
/**
* Convert points to points/1000, rounding up. This method guarantees
* that the result will not represent a measurement smaller than the
* given point value.
* @param f Floating point value representing number of points.
* @return Integer value representing a value in thousandths of a point.
*/
static int forceInt (float f) {
int result = toInt (f);
float reconvert = toFloat (result);
if (reconvert < f) {
result++;
}
return result;
}
public static String doubleToString (double value, int decimals) {
// TODO: this should part of some string utility class
StringBuilder result = new StringBuilder();
int i;
for (i = 0; i < decimals; i++) { // TODO: use Math.pow()?
value *= 10;
}
long rounded = Math.round (value);
prependZeros (result, Long.toString (rounded), decimals, true);
int decimalPos = result.length() - decimals;
int lastZero = result.length();
while (lastZero > decimalPos) {
i = lastZero - 1;
if (result.charAt (i) != '0') {
break;
}
lastZero = i;
}
result.delete (lastZero, result.length());
if (lastZero > decimalPos) {
result.insert (decimalPos, '.');
}
return result.toString();
}
static String toString (int pt1000) {
return toUnitSpan(pt1000).toString();
}
static String toString (float pts) {
return toUnitSpan(pts).toString();
}
static String intToString (int value, int minDigits, boolean zeroFill) {
StringBuilder result = new StringBuilder();
prependZeros (result, Integer.toString (value), minDigits, zeroFill);
return result.toString();
}
static String intToString (int value, int minDigits) {
return intToString (value, minDigits, false);
}
static String intToString (int value, boolean zerofill) {
return intToString (value, 5, zerofill);
}
static String intToString (int value) {
return intToString (value, 5, false);
}
static String hexToString (int hex, int minDigits, String prefix) {
StringBuilder result = new StringBuilder (prefix);
prependZeros (result, Integer.toHexString (hex), minDigits, true);
return result.toString();
}
static String hexToString (int hex, int minDigits) {
return hexToString (hex, minDigits, "\\x");
}
static String hexToString (int hex) {
return hexToString (hex, 4, "\\x");
}
private static UnitSpan toUnitSpan (int units, int pt1000) {
return new UnitSpan (units, pt1000);
}
private static void prependZeros (StringBuilder result, String formatted, int minDigits, boolean zeroFill) {
char fill = zeroFill ? '0' : ' ';
for (int i = formatted.length(); i < minDigits; i++) {
result.append (fill);
}
result.append (formatted);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy