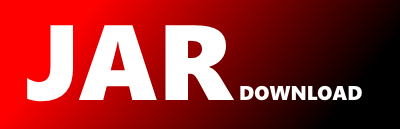
com.adobe.xfa.text.markup.MarkupIn Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.text.markup;
import com.adobe.xfa.font.FontService;
import com.adobe.xfa.text.TextAttr;
import com.adobe.xfa.text.TextField;
import com.adobe.xfa.text.TextGfxSource;
import com.adobe.xfa.text.TextMarkupBase;
import com.adobe.xfa.ut.Storage;
/**
* This class provides common base class support for input markup
* engines. It caches a pointer to the markup target (class
* TextMkBase) and provides a simple set of methods that the input
* engine can call to build up the rich text.
*
* For more information, please see the extenral documentation.
*
* @exclude from published api.
*/
abstract public class MarkupIn {
private final static Byte NULL_BYTE = Byte.valueOf((byte) '\0');
private final TextAttr moPrevAttr = new TextAttr();
private final TextAttr moPendingAttr = new TextAttr();
private TextMarkupBase mpoBase;
private boolean mbFirstPara;
private Storage moMBText;
// private CharConverter mpoCharConverter;
private TextGfxSource moGfxSource;
private boolean mbLegacyBlankLineMode;
/**
* Pure virtual: Perform the markup translation.
*
* The derived class implements this to perform the actual translation
* from markup to rich text. Note that the derived class must be
* prepopulated with any translation parameters.
*/
abstract public void translate ();
public void setup (TextMarkupBase poBase, TextGfxSource poGfxSource) {
moPrevAttr.setDefault (false);
moPendingAttr.setDefault (false);
mpoBase = poBase;
if (poGfxSource != null) {
moGfxSource = poGfxSource;
}
}
/**
* Protected defualt constructor.
*
* Initializes this base class. The target pointer is set to NULL.
*/
protected MarkupIn () {
mbFirstPara = true;
mbLegacyBlankLineMode = true;
// mpoCharConverter = CharConverter.default().clone (CharConverter.default().codePage());
}
/**
* Process raw text.
*
* The derived class calls this to accumulate a string of raw text at
* the target.
* @param sText - Text to accumulate.
*/
protected void text (String sText) {
if (sText.length() == 0) {
return;
}
commitPending (true); // flushes MB text
mpoBase.text (sText);
mbFirstPara = false;
}
/**
* Process attribute change.
*
* The derived class calls this method to set an attribute change for
* subsequent text. The change remains in effect until the next call to
* this method.
* @param oAttr - Attribute object to accumulate. Only enabled
* attributes apply; the target retains previous values for disabled
* attributes.
*/
protected void attr (TextAttr oAttr) {
moPendingAttr.override (oAttr);
}
/**
* Process raw text in multibyte format.
*
* The derived class calls this to accumulate a string of raw text at
* the target. The raw text is passed in multibyte format, using the
* current character converter (see the CharConverter() overloads).
* @param cText - Multi-byte text string.
*/
protected void mbText (byte[] cText) {
if (cText[0] == '\0') {
return;
}
commitPending();
if (moMBText == null) {
moMBText = new Storage();
}
if (moMBText.size() == 0) {
moMBText.add (NULL_BYTE);
}
for (int i = 0; cText[i] != '\0'; i++) {
moMBText.removeLast();
moMBText.add (Byte.valueOf(cText[i])); // TODO: UGH
moMBText.add (NULL_BYTE);
}
}
/**
* Returns the pending multibyte text.
* @return The pending multibyte text.
*/
protected String mbText () {
if (hasPendingMBText()) {
byte[] bytes = new byte [moMBText.size()];
for (int i = 0; i < moMBText.size(); i++) {
Byte b = moMBText.get (i);
bytes[i] = b.byteValue();
}
moMBText.clear();
return new String (bytes); // TODO: need to handle character conversions
}
return "";
}
/**
* Determine whether there is multibyte text pending.
* @return TRUE if there is multibyte text pending; FALSE if not.
*/
protected boolean hasPendingMBText () {
return (moMBText != null) && (moMBText.size() > 0);
}
/**
* Process paragraph break.
*
* The derived class calls this method to send a paragraph break to the
* target.
*/
protected void para () {
if (mpoBase.issueFirstPara() || (! mbFirstPara)) {
commitPending();
mpoBase.para();
}
mbFirstPara = false;
}
/**
* Process embedded field.
*
* The derived class calls this method to add an embedded field to the
* target.
* @param poField - Pointer to field to be accumulated. The target will
* clone a copy; ownership of the parameter remains with the caller.
*/
protected void field (TextField poField) {
commitPending (true);
mpoBase.field (poField);
if (! mbLegacyBlankLineMode) {
mbFirstPara = false;
}
}
/**
* Open scoped block.
*
* Only RTF cares about scoped blocks; this call is a null operation
* unless the target happens to be the RTF output engine.
*/
protected void openScopedBlock () {
mpoBase.openScopedBlock();
}
/**
* Open scoped block.
*
* Only RTF cares about scoped blocks; this call is a null operation
* unless the target happens to be the RTF output engine.
*/
protected void closeScopedBlock () {
mpoBase.closeScopedBlock();
}
/**
* Return the target.
* @return Pointer to the target for the markup operation
*/
protected TextMarkupBase posn () {
return mpoBase;
}
// protected void CharConverter (CharConverter poConv) {
// mpoCharConverter = null;
// mpoCharConverter = poConv.clone (poConv.codePage());
// }
// protected CharConverter CharConverter () {
// return mpoCharConverter;
// }
protected void commitPending (boolean bForceMBText) {
if (bForceMBText || (! moPendingAttr.isEmpty())) {
if ((moMBText != null) && (moMBText.size() > 0)) {
mpoBase.text (mbText());
}
}
if (! moPendingAttr.isEmpty()) {
TextAttr oDiffs = new TextAttr();
moPrevAttr.override (moPendingAttr, oDiffs);
if (! oDiffs.isEmpty()) {
mpoBase.attr (oDiffs);
}
moPendingAttr.setDefault (false);
}
}
protected void commitPending () {
commitPending (false);
}
// protected GFXAttrPool attrPool () {
// return moGfxSource.pool();
// }
protected FontService fontService () {
return moGfxSource.getFontService();
}
protected boolean legacyPositioning () {
return (mpoBase == null) ? false : mpoBase.legacyPositioning();
}
protected boolean legacyBlankLineMode () {
return mbLegacyBlankLineMode;
}
protected void setLegacyBlankLineMode (boolean bLegacyBlankLineMode) {
mbLegacyBlankLineMode = bLegacyBlankLineMode;
}
}