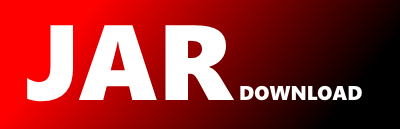
com.adobe.xfa.text.markup.RTFAttr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
//----------------------------------------------------------------------
// restore alignment to what it was before include
//
// File: textrtat.cpp
//
// Description
// Implementation file for class RTFAttr. This
// class defines details of the Rich Text Format (RTF).
//
// Change history
// Project Release Date Who Review What
// HawaiiRd 5.0 960411 EM Created.
//
//----------------------------------------------------------------------
package com.adobe.xfa.text.markup;
/*
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
class RTFAttr extends MarkupAttr {
final static RTFAttr DEFAULT_RTF_ATTR = new RTFAttr();
public RTFAttr () {
initializeMap();
}
//-----------------------------------------------------------------------------
// RTF commands having a font face name as their parameter.
//-----------------------------------------------------------------------------
public boolean IsFontNameSpecifier (String oStrCommand) {
return (oStrCommand.contains("alt"))
|| (oStrCommand.contains("fname"));
}
//----------------------------------------------------------------------
//
// GetDefault: Static member to return a default markup
// attribute object. Placed here for centralized access.
//
//----------------------------------------------------------------------
public static RTFAttr GetDefault () {
return DEFAULT_RTF_ATTR;
}
//-----------------------------------------------------------------------------
// Attributes of RTF markup
//-----------------------------------------------------------------------------
public char blockPrefix () {
return '{';
}
public char blockSuffix () {
return '}';
}
public char commandPrefix () {
return '\\';
}
///////////////////////////////////////////////////////////////////////////////
// Any non-lower case letter or non-digit is an RTF delimiter. However, some
// characters do have special meaning.
//
// Note: This test is only valid for rtf command strings, NOT text or
// quoted parameters.
///////////////////////////////////////////////////////////////////////////////
public boolean isDelimiter (char c) {
return (!((c == '*') || (c == '-') || (c == '\'') || (c == '\"') || (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z') || (c >= '0' && c <= '9'))); // Notation in newer command support // Used for negative numeric parameters // Used for specifying hex characters // Quote // RTF commands are ALWAYS lower case // Free form text may have upper case
}
//-----------------------------------------------------------------------------
// Disabling character for a boolean command parameter (e.g. bold/italic/etc.)
//-----------------------------------------------------------------------------
public char disablingChar () {
return '0';
}
public char delimiter () {
return ' ';
}
public int pointSizeFactor () {
return 2; // Half point increments
}
// If it's a valid RTF string we'll encounter something like \rtf1 early on
public boolean isValidMarkup (String sRtf) {
return sRtf.substring(0,8).contains("\\\rtf");
}
protected void initializeMap () {
// paragraph
// SetEntry (MARKUP_PARAGRAPH_START, jfLiteral ("")); // not supported
setEntry (MARKUP_PARAGRAPH_END, "par");
setEntry (MARKUP_PARAGRAPH_DEFAULT, "pard");
setEntry (MARKUP_PARAGRAPH_PLAIN, "plain");
setEntry (MARKUP_PICTURE, "pict");
// Header info.
setEntry (MARKUP_STYLESHEET, "stylesheet"); // not supported
setEntry (MARKUP_INFO, "info"); // not supported
setEntry (MARKUP_HEADER, "header"); // not supported
setEntry (MARKUP_HEADER_LEFT, "headerl"); // not supported
setEntry (MARKUP_HEADER_RIGHT, "headerr"); // not supported
setEntry (MARKUP_HEADER_FIRST, "headerf"); // not supported
setEntry (MARKUP_FOOTER, "footer"); // not supported
setEntry (MARKUP_FOOTER_LEFT, "footerl"); // not supported
setEntry (MARKUP_FOOTER_RIGHT, "footerr"); // not supported
setEntry (MARKUP_FOOTER_FIRST, "footerf"); // not supported
setEntry (MARKUP_CODE_PAGE, "ansicpg");
setEntry (MARKUP_CHARSET, "fcharset");
// Control sequences
setEntry (MARKUP_HEX_CHARACTER, "'", true);
setEntry (MARKUP_DESTINATION_GROUP, "*");
setEntry (MARKUP_UC_COUNT, "uc");
setEntry (MARKUP_UNICODE_CHARACTER, "u");
// Special Characters
setEntry (MARKUP_ENDASH, "endash");
setEntry (MARKUP_EMDASH, "emdash");
setEntry (MARKUP_ENSPACE, "emspace");
setEntry (MARKUP_EMSPACE, "enspace");
setEntry (MARKUP_BULLET, "bullet");
setEntry (MARKUP_LQUOTE, "lquote");
setEntry (MARKUP_RQUOTE, "rquote");
setEntry (MARKUP_LDBLQUOTE, "ldblquote");
setEntry (MARKUP_RDBLQUOTE, "rdblquote");
setEntry (MARKUP_LINE, "line");
// indentation
setEntry (MARKUP_INDENT_FIRST_LINE, "fi", true);
setEntry (MARKUP_INDENT_LEFT, "li", true);
setEntry (MARKUP_INDENT_RIGHT, "ri", true);
// SetEntry (MARKUP_OUTDENT, jfLiteral (""), TRUE); // not supported
setEntry (MARKUP_SPACE_BEFORE, "sb", true);
setEntry (MARKUP_SPACE_AFTER, "sa", true);
setEntry (MARKUP_LINE_SPACE, "sl", true);
// font
setEntry (MARKUP_FONT_NAME, "f", true);
setEntry (MARKUP_FONT_SIZE, "fs", true);
setEntry (MARKUP_DEFAULT_FONT, "deff", true);
// effects
setEntry (MARKUP_BOLD, "b");
setEntry (MARKUP_BOLD_END, "b0");
setEntry (MARKUP_ITALIC, "i");
setEntry (MARKUP_ITALIC_END, "i0");
setEntry (MARKUP_UNDERLINE_DASH_DOT_DOT, "uldashdd");
setEntry (MARKUP_UNDERLINE_DASH_DOT, "uldashd");
setEntry (MARKUP_UNDERLINE_DASH, "uldash");
setEntry (MARKUP_UNDERLINE_DOUBLE, "uldb");
setEntry (MARKUP_UNDERLINE_DOTTED, "uld");
setEntry (MARKUP_UNDERLINE, "ul");
setEntry (MARKUP_UNDERLINE_WORD, "ulw");
setEntry (MARKUP_UNDERLINE_END2, "ulnone");
setEntry (MARKUP_UNDERLINE_HEAVY_WAVE, "ulhwave");
setEntry (MARKUP_UNDERLINE_LONG_DASH, "ulldash");
setEntry (MARKUP_UNDERLINE_THICK, "ulth");
setEntry (MARKUP_UNDERLINE_THICK_DOT, "ulthd");
setEntry (MARKUP_UNDERLINE_THICK_DASH, "ulthdash");
setEntry (MARKUP_UNDERLINE_THICK_DASH_DOT, "ulthdashd");
setEntry (MARKUP_UNDERLINE_THICK_DASH_DOT_DOT, "ulthdashdd");
setEntry (MARKUP_UNDERLINE_THICK_LONG_DASH, "ulthldash");
setEntry (MARKUP_UNDERLINE_DOUBLE_WAVE, "ululdbwave");
setEntry (MARKUP_UNDERLINE_WAVE, "ulwave");
setEntry (MARKUP_UNDERLINE_END, "ul0");
setEntry (MARKUP_SUPER, "super");
setEntry (MARKUP_SUB, "sub");
setEntry (MARKUP_STRIKEOUT, "strike");
setEntry (MARKUP_STRIKEOUT_DOUBLE, "striked");
setEntry (MARKUP_STRIKEOUT_END, "strike0");
// SetEntry (MARKUP_UNDERLINE_WORD_DOUBLE, jfLiteral ("")); // not supported
setEntry (MARKUP_COLOUR_TABLE, "colortbl");
setEntry (MARKUP_COLOUR, "cf", true);
setEntry (MARKUP_DOWN, "dn", true);
setEntry (MARKUP_UP, "up", true);
// justification
setEntry (MARKUP_JUSTIFY_SPREAD, "qj");
// SetEntry (MARKUP_JUSTIFY_SPREAD_ALL, jfLiteral ("")); // not supported
setEntry (MARKUP_JUSTIFY_HORZ_LEFT, "ql");
setEntry (MARKUP_JUSTIFY_HORZ_CENTER, "qc");
setEntry (MARKUP_JUSTIFY_HORZ_RIGHT, "qr");
// SetEntry (MARKUP_JUSTIFY_VERT_TOP, jfLiteral ("")); // not supported
// SetEntry (MARKUP_JUSTIFY_VERT_CENTER, jfLiteral ("")); // not supported
// SetEntry (MARKUP_JUSTIFY_VERT_BOTTOM, jfLiteral ("")); // not supported
// tabs
setEntry (MARKUP_TAB, "tab");
setEntry (MARKUP_TAB_DEFAULT, "deftab", true);
setEntry (MARKUP_TAB_POSITION, "tx", true);
// SetEntry (MARKUP_TAB_ALIGN_LEFT, jfLiteral ("")); // not supported
setEntry (MARKUP_TAB_ALIGN_CENTER, "tqc");
setEntry (MARKUP_TAB_ALIGN_RIGHT, "tqr");
setEntry (MARKUP_TAB_ALIGN_DECIMAL, "tqd");
setEntry (MARKUP_STYLE_REF, "s");
setEntry (MARKUP_CHARACTER_STYLE, "cs");
setEntry (MARKUP_STYLE_BASED_ON, "sbasedon");
setEntry (MARKUP_STYLE_ADDITIVE, "additive");
setEntry (MARKUP_TABLE_ROW, "row");
setEntry (MARKUP_TABLE_CELL, "cell");
// Understood, but not supported
setMarkupEntry (MARKUP_NOT_SUPPORTED, "caps");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "deleted");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "nosupersub");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "expnd");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "expndtw");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "kerning");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "outl");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "shad");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "v");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "cb");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "rtlch");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "ltrch");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "cchs");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "lang");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "trowd");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "trleft");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "cl");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "ch");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "trowd");
setMarkupEntry (MARKUP_NOT_SUPPORTED, "cellx");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy