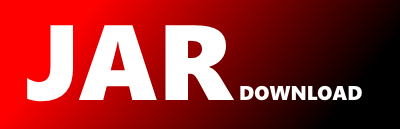
com.adobe.xfa.text.markup.XMLParserImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.xfa.text.markup;
import java.io.StringReader;
import java.io.IOException;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import org.xml.sax.Attributes;
import org.xml.sax.ContentHandler;
import org.xml.sax.ErrorHandler;
import org.xml.sax.InputSource;
import org.xml.sax.Locator;
import org.xml.sax.XMLReader;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
/**
* @exclude from published api.
*/
class XMLParserImpl implements ContentHandler, ErrorHandler {
private final XMLParserBase mClient;
XMLParserImpl (XMLParserBase client) {
mClient = client;
}
public void processText (String sText) {
try {
StringReader stringReader = new StringReader (sText);
InputSource inputSource = new InputSource (stringReader);
SAXParser parser = javax.xml.parsers.SAXParserFactory.newInstance().newSAXParser();
XMLReader xmlReader = parser.getXMLReader();
xmlReader.setContentHandler (this);
xmlReader.setErrorHandler (this);
xmlReader.parse (inputSource);
} catch (SAXException e) {
} catch (ParserConfigurationException e) {
} catch (IOException e) {
}
}
public void characters (char[] content, int start, int length) throws SAXException {
mClient.onContent (new String (content, start, length));
}
public void endDocument () throws SAXException {
}
public void endElement (String uri, String localName, String qName) throws SAXException {
mClient.onEndTag (resolveElement (uri, localName, qName));
}
public void endPrefixMapping (String arg0) throws SAXException {
}
public void ignorableWhitespace (char[] content, int start, int length) throws SAXException {
characters (content, start, length);
}
public void processingInstruction (String arg0, String arg1) throws SAXException {
}
public void setDocumentLocator (Locator arg0) {
}
public void skippedEntity (String arg0) throws SAXException {
}
public void startDocument () throws SAXException {
}
public void startElement (String uri, String localName, String qName, Attributes attributes) throws SAXException {
mClient.onStartTag (resolveElement (uri, localName, qName), attributes);
}
public void startPrefixMapping (String arg0, String arg1) {
}
public void error (SAXParseException exception) throws SAXException {
}
public void fatalError (SAXParseException exception) throws SAXException {
throw exception;
}
public void warning (SAXParseException exception) throws SAXException {
}
private String resolveElement (String uri, String localName, String qName) {
if ((localName != null) && (localName.length() > 0)) {
return localName;
}
if ((qName != null) && (qName.length() > 0)) {
return qName;
}
return uri;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy