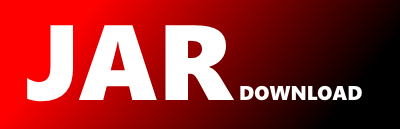
com.adobe.xfa.ut.ISOTime Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut;
import java.util.Calendar;
import java.util.TimeZone;
/**
* The ISOTime class derives from LcTime to
* define ISO8601/XFA time patterns.
*
* Valid ISO8601/XFA time strings are in any one
* of the following time patterns:
*
* - HH[MM[SS[.FFF][z]]]
*
- HH[MM[SS[.FFF][+HH[MM]]]]
*
- HH[MM[SS[.FFF][-HH[MM]]]]
*
- HH[:MM[:SS[.FFF][z]]]
*
- HH[:MM[:SS[.FFF][+HH[:MM]]]]
*
- HH[:MM[:SS[.FFF][-HH[:MM]]]]
*
* where [...] denotes optional elements.
* See {@link LcTime} for the meaning of the various metasymbols used above.
*
* Here's a snippet of code illustrating the use of
* {@link ISOTime} to reformat an ISO8601/XFA time string
*
* import com.adobe.xfa.ut.ISOTime;
* import com.adobe.xfa.ut.LcTime;
* ...
* ISOTime t = new ISOTime("11:12:13-05:00", "");
* fString s = "Unknown";
* if (t.isValid())
* s = t.format(LcTime.XFA_TIME_FMT1);
* System.out.println(s);
*
*
* @exclude from published api.
*/
public final class ISOTime extends LcTime {
/**
* Instantiate an ISOTime object from today's time.
*/
public ISOTime() {
super(LcLocale.DEFAULT_LOCALE);
}
/**
* Instantiate an ISOTime object from the given number of milliseconds
* from the epoch. The epoch is such that millisecond 1 corresponds
* to midnight, 00:00:00 GMT.
* @param millis the number of milliseconds from epoch.
*/
public ISOTime(int millis) {
super(millis, LcLocale.DEFAULT_LOCALE);
}
/**
* Instantiate an ISOTime object from today's time given a locale string
* and a possible century split year.
* @param locale a locale string. When empty, it will default
* to the current locale.
* @param centurySplit a century split year.
*/
public ISOTime(String locale, int centurySplit) {
super(locale);
}
/**
* Instantiate an ISOTime object from the given time string.
* @param time an ISO8601/XFA time string.
* @param locale a locale string. When empty, it will default
* to the current locale.
*/
public ISOTime(String time, String locale /* = "" */) {
super(locale);
setTimeSymbols(mLocale.getIsoName());
//
// Remove any ISO date component from time string.
//
String trimTime = time;
int tee = time.indexOf('T');
if (tee >= 0)
trimTime = time.substring(tee + 1);
//
// Remove any leading and trailing white spaces from time string.
//
trimTime = trimTime.trim();
setTimeSymbols(LcLocale.DEFAULT_LOCALE);
if (parse(trimTime)) {
//
// Adjust for local time if no timezone was parsed.
//
if (! mTimeZoneSeen) {
Calendar now = Calendar.getInstance();
mTimeZone = - now.get(Calendar.ZONE_OFFSET);
if (TimeZone.getDefault().inDaylightTime(now.getTime()))
mTimeZone -= now.get(Calendar.DST_OFFSET);
}
mMillis = epoch(mHourOfDay, mMinuteOfHour, mSecondOfMinute,
mThousandthOfSecond, mTimeZone);
mAdjustment = 0;
mValid = true;
}
else {
mMillis = 0;
mValid = false;
}
setTimeSymbols(mLocale.getIsoName());
}
/*
* Parse the given time string.
*
* @param src the time string to parse. Valid ISO times are in one
* of the following formats:
*
* - HH[MM[SS[.FFF][z]]]
*
- HH[MM[SS[.FFF][+HH[MM]]]]
*
- HH[MM[SS[.FFF][-HH[MM]]]]
*
- HH[:MM[:SS[.FFF][z]]]
*
- HH[:MM[:SS[.FFF][+HH[:MM]]]]
*
- HH[:MM[:SS[.FFF][-HH[:MM]]]]
*
* @return boolean true if successfully parsed, and false otherwise.
*/
boolean parse(String src) {
boolean needColons = (src.indexOf(':') >= 0);
int srcPos = 0;
int srcLen = src.length();
int parseRes;
//
// Reset parsed time sub-elements.
//
mHourOfDay = mMinuteOfHour = mSecondOfMinute = mThousandthOfSecond = -1;
mHourOfMeriDiem = mMeriDiem = mTimeZone = -1;
mTimeZoneSeen = false;
//
// Parse hours.
//
if (srcPos + 2 > srcLen)
return false;
parseRes = subParse(src, srcPos, 'H', 2);
if (parseRes < 0)
return false;
srcPos = parseRes;
//
// Return if we're done.
//
if (srcPos == srcLen) {
mMinuteOfHour = 0;
mSecondOfMinute = 0;
mThousandthOfSecond = 0;
return true;
}
if (needColons) {
if (! DateTimeUtil.matchChr(src, srcPos, ':', false))
return false;
srcPos += 1;
}
//
// Parse any optional minutes.
//
if (srcPos + 2 > srcLen)
return false;
parseRes = subParse(src, srcPos, 'M', 2);
if (parseRes < 0)
return false;
srcPos = parseRes;
//
// Return if we're done.
//
if (srcPos == srcLen) {
mSecondOfMinute = 0;
mThousandthOfSecond = 0;
return true;
}
if (needColons) {
if (! DateTimeUtil.matchChr(src, srcPos, ':', false))
return false;
srcPos += 1;
}
//
// Parse any optional seconds.
//
if (srcPos + 2 > srcLen)
return false;
parseRes = subParse(src, srcPos, 'S', 2);
if (parseRes < 0)
return false;
srcPos = parseRes;
//
// Return if we're done.
//
if (srcPos == srcLen) {
mThousandthOfSecond = 0;
return true;
}
//
// Parse any optional milliseconds.
//
if (DateTimeUtil.matchChr(src, srcPos, '.', false)) {
srcPos += 1;
if (srcPos + 3 > srcLen)
return false;
parseRes = subParse(src, srcPos, 'F', 3);
if (parseRes < 0)
return false;
srcPos = parseRes;
//
// Return if we're done.
//
if (srcPos == srcLen)
return true;
}
//
// Parse any optional timezones.
//
parseRes = subParse(src, srcPos, 'z', (needColons) ? 2 : 1);
if (parseRes < 0)
return false;
srcPos = parseRes;
//
// Ensure there's no more source to parse.
//
if (srcPos != srcLen)
return false;
if (mThousandthOfSecond == -1)
mThousandthOfSecond = 0;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy