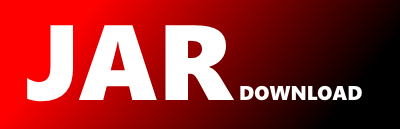
com.adobe.xfa.ut.lcdata.LcBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut.lcdata;
/**
* A utility class to access the properties of Locale resource bundles.
*
* @exclude from published api.
*/
public final class LcBundle {
/*
* Disallow instances of this class.
*/
private LcBundle() {
// empty
}
/**
* The base name of property resource bundles.
*/
public static final String BUNDLE_BASE = "com/adobe/xfa/ut/lcdata/Locale";
private static final String[] LONG_MONTH_PROPERTIES = {
"gregorian.month.full.jan",
"gregorian.month.full.feb",
"gregorian.month.full.mar",
"gregorian.month.full.apr",
"gregorian.month.full.may",
"gregorian.month.full.jun",
"gregorian.month.full.jul",
"gregorian.month.full.aug",
"gregorian.month.full.sep",
"gregorian.month.full.oct",
"gregorian.month.full.nov",
"gregorian.month.full.dec"
};
public static String getLongMonthProperty(int month) {
return LONG_MONTH_PROPERTIES[month];
}
private static final String[] SHORT_MONTH_PROPERTIES = {
"gregorian.month.abbr.jan",
"gregorian.month.abbr.feb",
"gregorian.month.abbr.mar",
"gregorian.month.abbr.apr",
"gregorian.month.abbr.may",
"gregorian.month.abbr.jun",
"gregorian.month.abbr.jul",
"gregorian.month.abbr.aug",
"gregorian.month.abbr.sep",
"gregorian.month.abbr.oct",
"gregorian.month.abbr.nov",
"gregorian.month.abbr.dec"
};
public static String getShortMonthProperty(int month) {
return SHORT_MONTH_PROPERTIES[month];
}
private static final String[] LONG_WEEKDAY_PROPERTIES = {
"gregorian.weekday.full.sun",
"gregorian.weekday.full.mon",
"gregorian.weekday.full.tue",
"gregorian.weekday.full.wed",
"gregorian.weekday.full.thu",
"gregorian.weekday.full.fri",
"gregorian.weekday.full.sat"
};
public static String getLongDayProperty(int weekday) {
return LONG_WEEKDAY_PROPERTIES[weekday];
}
private static final String[] SHORT_WEEKDAY_PROPERTIES = {
"gregorian.weekday.abbr.sun",
"gregorian.weekday.abbr.mon",
"gregorian.weekday.abbr.tue",
"gregorian.weekday.abbr.wed",
"gregorian.weekday.abbr.thu",
"gregorian.weekday.abbr.fri",
"gregorian.weekday.abbr.sat"
};
public static String getShortDayProperty(int weekday) {
return SHORT_WEEKDAY_PROPERTIES[weekday];
}
private static final String[] MERIDIEM_PROPERTIES = {
"gregorian.meridiem.am",
"gregorian.meridiem.pm"
};
public static String getMeridiemProperty(int aspect) {
return MERIDIEM_PROPERTIES[aspect];
}
private static final String[] ERA_PROPERTIES = {
"gregorian.era.bc",
"gregorian.era.ad"
};
public static String getEraProperty(int era) {
return ERA_PROPERTIES[era];
}
private static final String[] DATE_PATTERN_PROPERTIES = {
"gregorian.date.pattern.full",
"gregorian.date.pattern.long",
"gregorian.date.pattern.medium",
"gregorian.date.pattern.short"
};
public static String getDateFormatProperty(int style) {
return DATE_PATTERN_PROPERTIES[style];
}
private static final String[] TIME_PATTERN_PROPERTIES = {
"gregorian.time.pattern.full",
"gregorian.time.pattern.long",
"gregorian.time.pattern.medium",
"gregorian.time.pattern.short"
};
public static String getTimeFormatProperty(int style) {
return TIME_PATTERN_PROPERTIES[style];
}
public static String getDateTimeFormatProperty() {
return "gregorian.datetime.pattern";
}
public static String getDateTimeSymbolsProperty() {
return "gregorian.datetime.symbols";
}
private static final String[] NUMBER_PATTERN_PROPERTIES = {
"number.pattern.numeric",
"number.pattern.currency",
"number.pattern.percent"
};
public static String getNumberFormatProperty(int style) {
return NUMBER_PATTERN_PROPERTIES[style];
}
private static final String[] NUMERIC_SYMBOL_PROPERTIES = {
"numeric.symbol.decimal",
"numeric.symbol.grouping",
"numeric.symbol.percent",
"numeric.symbol.minus",
"numeric.symbol.zero"
};
public static String getNumericSymbolProperty(int symbol) {
return NUMERIC_SYMBOL_PROPERTIES[symbol];
}
private static final String[] CURRENCY_SYMBOL_PROPERTIES = {
"currency.symbol.sym",
"currency.symbol.name",
"currency.symbol.decimal"
};
public static String getCurrencySymbolProperty(int symbol) {
return CURRENCY_SYMBOL_PROPERTIES[symbol];
}
public static String getTypefacesProperty() {
return "font.typefaces";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy