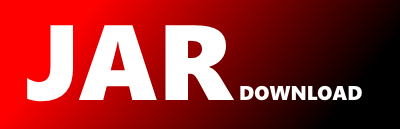
com.adobe.xfa.ut.trace.TraceTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2008 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut.trace;
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
/**
* @exclude from published api.
*/
public final class TraceTimer {
private final TraceHandler.TimingType meType;
private long mnStart;
private static final boolean gUseCpuTime;
private static final ThreadMXBean gBean;
static {
boolean useCpuTime = (System.getProperty("test.use.cputime") != null);
if (useCpuTime) {
gBean = ManagementFactory.getThreadMXBean();
if (! gBean.isCurrentThreadCpuTimeSupported())
useCpuTime = false;
}
else {
gBean = null;
}
gUseCpuTime = useCpuTime;
}
public TraceTimer(TraceHandler.TimingType eType) {
meType = eType;
if (TraceHandler.timingTraceEnabled())
doStartTimer();
}
// This should help us detect if we're starting the same timer twice
public void startTiming() {
if (TraceHandler.timingTraceEnabled())
doStartTimer();
}
/**
* Stop the timer and add the time to the totals being accumulated in TraceHandler.
* In this Java implementation, this method needs to be called explicitly since
* we can't rely on the destructor being called to stop the timer.
*/
public void stopTiming() {
if (TraceHandler.timingTraceEnabled())
doStopTimer();
}
private void doStartTimer() {
// This should help us detect if we're starting the same timer twice
assert mnStart == 0;
mnStart = getNanos();
}
private void doStopTimer() {
if (mnStart != 0) {
TraceHandler.addTimerNanos(meType, getNanos() - mnStart);
mnStart = 0;
}
}
private static long getNanos() {
return (gUseCpuTime) ? gBean.getCurrentThreadCpuTime() : System.nanoTime();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy