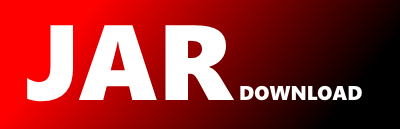
com.adobe.xfa.wsdl.WSDLDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2008 Adobe Systems Incorporated
* All Rights Reserved
*
* Notice: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.wsdl;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Document;
import com.adobe.xfa.Element;
import com.adobe.xfa.Node;
import com.adobe.xfa.protocol.ProtocolUtils;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.ResId;
import com.adobe.xfa.ut.StringUtils;
/**
* WSDLDocument
is the top node in the overall
* WSDL DOM.
* @exclude from published api.
*/
public class WSDLDocument extends WSDLNode {
private static class ImportedDocInfo {
public String docName;
public String importNS;
public Document oDoc;
public WSDLNode oDefinitions;
}
static final class PrefixParseInfo {
PrefixParseInfo (String localName, String targetNS) {
mLocalName = localName;
mTargetNS = targetNS;
}
final String mLocalName;
final String mTargetNS;
}
private WSDLNode moDefinitions;
private String mTargetNS;
private String mOpenFileName;
private final List mImportFileNameStack = new ArrayList();
private final List mImportedDomDocuments = new ArrayList();
private final List mTargetNSStack = new ArrayList();
public WSDLDocument () {
super (null, null, WSDLNode.WSDL_DOCUMENT);
}
/**
* Loads a file/datasource into a document from a file, and creates an
* abstract schema hierarchy for it.
*
* @param sFilename - the name of the file that contains the data to load.
* @param sLoadOptions - (optional) loading options.
* @return The created abstract schema model
* @exception AS_INVALIDDTDEXCEPTION - thrown if an invalid DTD definition
* is encountered
* @exception AS_CREATESCHEMAEXCEPTION - thrown if failed to load and create
* an XML Schema definition
* @exception AS_INVALIDELEMENTNAMEEXCEPTION - thrown if schema defines an invalid
* name for an element declaration
*/
public static WSDLDocument loadFromFile (String sFilename, String sLoadOptions) {
InputStream oStreamFile = openFile (sFilename);
WSDLDocument poWSDLDoc = null;
try {
poWSDLDoc = loadFromStream (oStreamFile, sFilename, sLoadOptions);
} catch (ExFull oEx) {
// only throw in test mode - need to either provide better error
// reporting here or update callers (Designer) to handle a throw.
if (sLoadOptions.contains("mode='test'")) {
throw oEx;
}
return null;
}
return poWSDLDoc;
}
/**
* Loads a file/datasource into a model from a stream, and creates an
* abstract schema hierarchy for it.
*
* @param oStream - The stream that contains the data to load.
* @param sFilename - Input file name.
* @param sLoadOptions - (optional) loading options.
* @return The created abstract schema model
* @exception AS_INVALIDDTDEXCEPTION - thrown if an invalid DTD definition
* is encountered
* @exception AS_CREATESCHEMAEXCEPTION - thrown if failed to load and create
* an XML Schema definition
* @exception AS_INVALIDELEMENTNAMEEXCEPTION - thrown if schema defines an invalid
* name for an element declaration
*/
public static WSDLDocument loadFromStream (InputStream oStream, String sFilename, String sLoadOptions) {
// when an exception is thrown during parsing
// the document will be destroyed
WSDLDocument poDoc = new WSDLDocument();
Document oDomDoc = loadDocument (oStream, sFilename, sLoadOptions);
poDoc.mOpenFileName = sFilename;
poDoc.loadChildren (oDomDoc);
if (poDoc.moDefinitions == null) {
return null;
}
poDoc.consolidateBindings();
return poDoc;
}
/**
* Get the definitions node
* @return the WSDLNode
that is the definitions node of type WSDL_DEFINITIONS
*/
public WSDLNode getDefinitionsNode () {
return moDefinitions;
}
/**
* Get the target namespace specifed by the WSDL
* @return the target namespace URI
*/
public String getTargetNS () {
return mTargetNS;
}
/**
* Get the uri where this WSDL originally resides
* @return the uri where this WSDL originally resides
*/
public String getOpenFileName () {
return mOpenFileName;
}
/**
* Given the full qname, check if there is a prefix. If there is, check if that
* prefix matches the Document targetNamespace or the namespace on one of the imported
* documents. If it does match return true, otherwise false. Also return the parsed
* local name and the targetNamespace it matched
* @param inFullName - the full qname in form "nsprefix:localname"
* @param inReferencingNode - node referencing "inFullName"
* @return Structure describing the parse.
*/
PrefixParseInfo checkAndParsePrefix (String inFullName, WSDLNode inReferencingNode) {
int i;
String outLocalName = null;
String outTargetNS = null;
int nPos = inFullName.indexOf (':');
if (nPos >= 0) {
String prefix = inFullName.substring (0, nPos);
String localName = inFullName.substring (nPos+1, inFullName.length());
outLocalName = localName;
String docTargetNS = getTargetNS();
// find out what namespace the prefix evaluates to
// (making sure we're checking the namespace prefix values
// for the appropriate definitions node if we're searching from
// an imported doc)
String prefixExpanded = null;
// check if we're searching from an imported doc
String referencingTargetNS = null;
if (inReferencingNode != null) {
referencingTargetNS = inReferencingNode.getTargetNamespace();
}
int importCount = mImportedDomDocuments.size();
for (i = 0; i < importCount; i++) {
ImportedDocInfo importedDoc = mImportedDomDocuments.get (i);
if (StringUtils.equalsWithNull (referencingTargetNS, importedDoc.importNS)) {
WSDLNode oDefinitions = importedDoc.oDefinitions;
if (oDefinitions != null) {
prefixExpanded = oDefinitions.getNSURI (prefix);
}
break;
}
}
if (StringUtils.isEmpty (prefixExpanded) && (moDefinitions != null)) {
// if not expanded yet, try the main doc
prefixExpanded = moDefinitions.getNSURI (prefix);
}
outTargetNS = prefixExpanded;
if (StringUtils.equalsWithNull (prefixExpanded, docTargetNS)) {
// this is the Document's targetNamespace
return new PrefixParseInfo (outLocalName, outTargetNS);
}
for (i = 0; i < importCount; i++) {
// check the other targetNamespaces from the imported docs
if (StringUtils.equalsWithNull (prefixExpanded, mImportedDomDocuments.get(i).importNS)) {
return new PrefixParseInfo (outLocalName, outTargetNS);
}
}
return null;
}
// no prefix, just return the doc's prefix
return new PrefixParseInfo (inFullName, getTargetNS());
}
public String getCurrentFileName () {
String sFileName = mOpenFileName;
int stacksize = mImportFileNameStack.size();
if (stacksize > 0) {
sFileName = mImportFileNameStack.get (stacksize - 1);
}
return sFileName;
}
private void loadChildren (Node oStartNode) {
// first node should be the node
Node oDomChild = oStartNode.getFirstXMLChild();
while (oDomChild != null) {
// create the children and add them:
if (oDomChild instanceof Element) {
Element e = (Element) oDomChild;
String aLocalName = e.getLocalName();
if (aLocalName == WSDL.DEFINITIONS) {
WSDLNode oDefinitions = createStandardNode (e, WSDLNode.WSDL_DEFINITIONS);
// go through the attributes to get the namespace URI definitions
Element definitionsDomNode = oDefinitions.getDomNode();
if (definitionsDomNode != null) {
int attrCount = definitionsDomNode.getNumAttrs();
for (int i = 0; i < attrCount; i++) {
Attribute oDomAttr = definitionsDomNode.getAttr (i);
String aNodeName = oDomAttr.getLocalName();
if (aNodeName == WSDL.TARGET_NAMESPACE) {
// set up all the namespaces
mTargetNS = oDomAttr.getAttrValue();
mTargetNSStack.add (mTargetNS);
oDefinitions.setTargetNamespace (mTargetNS);
break;
}
}
}
CreateDefinitionsEnumerator enumerator = new CreateDefinitionsEnumerator (e, oDefinitions);
enumerator.enumerate();
moDefinitions = oDefinitions;
// add this new node to this Document's ChildNodes array
appendWSDLChild (oDefinitions);
int targetNSSize = mTargetNSStack.size();
if (targetNSSize > 0) {
mTargetNSStack.remove(targetNSSize - 1);
}
}
}
oDomChild = oDomChild.getNextXMLSibling();
}
}
private void consolidateBindings () {
// here we want to link up all the operation nodes with their corresponding binding nodes
WSDLNode current = getDefinitionsNode().getFirstWSDLNode (WSDL_BINDING);
while (current != null) {
WSDLNode oBinding = current;
current = current.getNextWSDLNode (WSDL_BINDING); // because continue is used in loop
// first figure out what portType this binding relates to
String bindingPortType = oBinding.getWSDLAttribute (WSDLA_TYPE);
WSDLNode portType = null;
if (!StringUtils.isEmpty(bindingPortType)) {
PrefixParseInfo prefixParseInfo = checkAndParsePrefix (bindingPortType, oBinding);
if (prefixParseInfo == null) {
// the bindingPortType did not have the correct prefix corresponding to the document TargetNS
// or any of the imported docs
continue;
}
// now get the appropriate portType
portType = getDefinitionsNode().getWSDLChildNode (WSDL_PORTTYPE, prefixParseInfo.mLocalName, prefixParseInfo.mTargetNS);
}
if (portType == null) {
// if we didn't find one we can't continue here
continue;
}
// get the array of binding operaiton Nodes
WSDLNode operationSource = oBinding.getFirstWSDLNode (WSDL_BINDING_OPERATION);
while (operationSource != null) {
WSDLNode next = operationSource.getNextWSDLNode (WSDL_BINDING_OPERATION);
// get the operation node corresponding to this binding operation Node
WSDLNode childOp = portType.getWSDLChildNode (WSDLNode.WSDL_OPERATION, operationSource.getWSDLName());
if (childOp instanceof WSDLOperation) {
WSDLOperation operationNode = (WSDLOperation) childOp;
if (operationSource instanceof WSDLBindingOperation) {
WSDLBindingOperation bindingOperation = (WSDLBindingOperation) operationSource;
// add the current bindingoperation to the operation's bindingoperationnode array!
operationNode.addBindingOperation (operationSource);
// now set up the info in the bindingoperations node
bindingOperation.setOperation (operationNode);
}
}
operationSource = next;
}
}
}
private static Document loadDocument (InputStream stream, String fileName, String loadOptions) {
AppModel appModel = new AppModel (null);
Document result = appModel.getDocument();
Element bogusRoot = null;
try {
bogusRoot = result.loadIntoDocument (stream);
}
catch (ExFull oEx) {
// don't throw if the error is an XML parse error
int resId = oEx.firstResId();
if (resId != ResId.EXPAT_ERROR) {
throw (oEx); // XML parse error
}
return null;
}
finally {
try { stream.close(); }
catch (IOException e) { }
}
result.appendChild(bogusRoot.getFirstXMLChildElement());
return result;
}
private static InputStream openFile (String fileName) {
InputStream inputStream = ProtocolUtils.openUrl (fileName);
if (inputStream == null) {
ExFull ex = new ExFull (ResId.FILE_ERR_MUST_EXIST, fileName);
throw ex;
}
return inputStream;
}
private String resolveLocation (String sLocation) {
int position = 0;
if (sLocation.startsWith("/")) {
// absolute "relative" path - append to schema and authority from base path;
// See IETF's RFC 2396. Watson 1282381
String directory = getCurrentFileName();
position = directory.indexOf ("://");
if (position >= 0) {
URL url = null;
try {
url = new URL (directory);
} catch (IOException e) {
}
if (url != null) {
String sServer = url.getHost();
String sPath = url.getPath();
position = -1;
if (sServer.length() > 0) {
position = sPath.indexOf ('/'); // must contain at least one '/' in path according to C++ code
}
if (position >= 0) {
String sAuthority = sPath.substring (0, position);
return sServer + sAuthority + sLocation;
}
}
}
}
position = sLocation.indexOf ("://");
if (position < 0) {
// this is a relative path since there is no "://" in the location
String directory = getCurrentFileName();
char cDirSep = '/'; // normally
if ((directory.indexOf('/') < 0) && (directory.indexOf('\\') >= 0)) {
cDirSep = '\\'; // base file is local windows filepath
}
int offset = 0;
for (;;) {
position = directory.indexOf (cDirSep, offset+1);
if (position < 0) {
break;
}
offset = position;
}
directory = directory.substring (0, offset + 1);
return directory + sLocation;
}
return sLocation;
}
private WSDLNode createTypesNode (Element oStartNode) {
return createStandardNode (oStartNode, WSDLNode.WSDL_TYPES);
}
private WSDLNode createMessageNode (Element oDomNode) {
WSDLMessage poMessage = new WSDLMessage (this, oDomNode);
setStandardNodeProps (oDomNode, poMessage);
CreatePartEnumerator enumerator = new CreatePartEnumerator (oDomNode, poMessage);
enumerator.enumerate();
return poMessage;
}
private WSDLNode createPortTypeNode (Element oStartNode) {
WSDLNode portTypeNode = createStandardNode (oStartNode, WSDLNode.WSDL_PORTTYPE);
CreateOperationEnumerator enumerator = new CreateOperationEnumerator (oStartNode, portTypeNode);
enumerator.enumerate();
return portTypeNode;
}
private WSDLNode createBindingNode (Element oStartNode) {
WSDLNode bindingNode = createStandardNode (oStartNode, WSDLNode.WSDL_BINDING);
CreateOperationEnumerator enumerator = new CreateOperationEnumerator (oStartNode, bindingNode);
enumerator.enumerate();
return bindingNode;
}
private WSDLNode createServiceNode (Element oStartNode) {
WSDLNode serviceNode = createStandardNode (oStartNode, WSDLNode.WSDL_SERVICE);
CreatePortEnumerator enumerator = new CreatePortEnumerator (oStartNode, serviceNode);
enumerator.enumerate();
return serviceNode;
}
private WSDLNode createDocumentationNode (Element oDomNode) {
return null;
}
private void createImportNode (Element oImportNode, WSDLNode oParentWSDLNode) {
if (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_DEFINITIONS) {
String sLocation = null;
String sNamespace = null;
// get the 'location' attribute
int attrIndex = oImportNode.findAttr (null, WSDL.LOCATION);
if (attrIndex >= 0) {
sLocation = oImportNode.getAttrVal (attrIndex);
}
// get the namespace attribute
attrIndex = oImportNode.findAttr (null, WSDL.NAMESPACE);
if (attrIndex >= 0) {
sNamespace = oImportNode.getAttrVal (attrIndex);
}
if (! StringUtils.isEmpty (sLocation)) {
// first we should check if this is a relative path, if so we need to
// make it a full one.
sLocation = resolveLocation (sLocation);
int importCount = mImportedDomDocuments.size();
for (int i = 0; i < importCount; i++) {
// check if we've already imported this location.
ImportedDocInfo oDocInfo = mImportedDomDocuments.get (i);
if (StringUtils.equalsWithNull (oDocInfo.docName, sLocation)
&& StringUtils.equalsWithNull (oDocInfo.importNS, sNamespace)) {
// we already imported this doc under this namespace, so just return here
return;
}
}
// we haven't imported this one yet, go ahead and import it.
InputStream oStreamFile = openFile (sLocation);
Document oDomDoc = loadDocument (oStreamFile, sLocation, "");
if (oDomDoc != null) {
ImportedDocInfo oDocInfo = new ImportedDocInfo();
oDocInfo.docName = sLocation;
oDocInfo.importNS = sNamespace;
oDocInfo.oDoc = oDomDoc;
mImportedDomDocuments.add (oDocInfo);
// push the namespace onto the stack
mTargetNSStack.add (sNamespace);
// push the filename onto the stack
mImportFileNameStack.add (sLocation);
// create the children and add them:
Node oDomChild = oDomDoc.getFirstXMLChild();
if (oDomChild instanceof AppModel) {
oDomChild = oDomChild.getFirstXMLChild();
}
while (oDomChild != null) {
if (oDomChild instanceof Element) {
Element e = (Element) oDomChild;
if (e.getLocalName() == WSDL.DEFINITIONS) {
// create an orphaned definitions wsdl node to gather any namespace prefixes defined on it
WSDLNode oImportDefinitions = createStandardNode (e, WSDLNode.WSDL_DEFINITIONS);
mImportedDomDocuments.get(mImportedDomDocuments.size()-1).oDefinitions = oImportDefinitions;
CreateDefinitionsEnumerator enumerator = new CreateDefinitionsEnumerator (e, oParentWSDLNode);
enumerator.enumerate();
}
}
oDomChild = oDomChild.getNextXMLSibling();
}
mTargetNSStack.remove (mTargetNSStack.size()-1);
mImportFileNameStack.remove (mImportFileNameStack.size()-1);
}
}
}
}
// helpers
private WSDLNode createStandardNode (Element oDomNode, int eType) {
WSDLNode node = new WSDLNode (this, oDomNode, eType);
setStandardNodeProps (oDomNode, node);
return node;
}
private void setStandardNodeProps (Element oDomNode, WSDLNode poNodeImp) {
// get name
String nodeName = null;
int attrIndex = oDomNode.findAttr (null, WSDL.NAME);
if (attrIndex >= 0) {
nodeName = oDomNode.getAttrVal (attrIndex);
}
poNodeImp.setLocalName (oDomNode.getLocalName()); // setLocalName, setNamespaceURI, and setPrefix may eventually take a Atom
poNodeImp.setNamespaceURI (oDomNode.getNS());
poNodeImp.setWSDLPrefix (oDomNode.getPrefix());
poNodeImp.setWSDLName (nodeName);
if (mTargetNSStack.size() > 0) {
poNodeImp.setTargetNamespace (mTargetNSStack.get (mTargetNSStack.size() - 1));
}
}
private WSDLNode createExtenNode (Element oDomNode, int eType) {
WSDLExten oExten = new WSDLExten (this, oDomNode);
setStandardNodeProps (oDomNode, oExten);
oExten.setExtenType (eType);
return oExten;
}
private abstract class NodeEnumerator {
private Element mDOMNode;
private WSDLNode mParentWSDLNode;
NodeEnumerator (Element domNode, WSDLNode parentWSDLNode) {
mDOMNode = domNode;
mParentWSDLNode = parentWSDLNode;
}
void enumerate () {
Node oDomChild = mDOMNode.getFirstXMLChild();
while (oDomChild != null) {
if (oDomChild instanceof Element) {
WSDLNode oWSDLNode = null;
oWSDLNode = processNode ((Element) oDomChild);
if (oWSDLNode != null) {
// add this new node to the parent's ChildNodes array
mParentWSDLNode.appendWSDLChild (oWSDLNode);
}
}
oDomChild = oDomChild.getNextXMLSibling();
}
}
Element getDomNode() {
return mDOMNode;
}
WSDLNode getParentWSDLNode() {
return mParentWSDLNode;
}
abstract WSDLNode processNode (Element domChild);
}
private class CreateDefinitionsEnumerator extends NodeEnumerator {
CreateDefinitionsEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element domChild) {
String aLocalName = domChild.getLocalName();
WSDLNode oWSDLNode = null;
if (aLocalName == WSDL.TYPES) {
oWSDLNode = createTypesNode (domChild);
} else if (aLocalName == WSDL.MESSAGE) {
oWSDLNode = createMessageNode (domChild);
} else if (aLocalName == WSDL.PORT_TYPE) {
oWSDLNode = createPortTypeNode (domChild);
} else if (aLocalName == WSDL.BINDING) {
oWSDLNode = createBindingNode (domChild);
} else if (aLocalName == WSDL.SERVICE) {
oWSDLNode = createServiceNode (domChild);
} else if (aLocalName == WSDL.DOCUMENTATION) {
oWSDLNode = createDocumentationNode (domChild);
} else if (aLocalName == WSDL.IMPORT) {
// the import node has to place children nodes directly into the parent node.
createImportNode (domChild, getParentWSDLNode());
} else {
// The definition node has an unknown element. Just ignore it.
}
return oWSDLNode;
}
}
// part of message
private class CreatePartEnumerator extends NodeEnumerator {
CreatePartEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element domChild) {
String aLocalName = domChild.getLocalName();
WSDLNode oWSDLNode = null;
if (aLocalName == WSDL.PART) {
oWSDLNode = new WSDLPart (WSDLDocument.this, domChild);
setStandardNodeProps (domChild, oWSDLNode);
}
return oWSDLNode;
}
}
// part of PortType and Binding
private class CreateOperationEnumerator extends NodeEnumerator {
CreateOperationEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element oDomNode) {
String aLocalName = oDomNode.getLocalName();
WSDLNode oParentWSDLNode = getParentWSDLNode();
WSDLNode oNode = null;
if (aLocalName == WSDL.OPERATION) {
if (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_PORTTYPE) {
WSDLOperation oOperation = new WSDLOperation (WSDLDocument.this, oDomNode);
setStandardNodeProps (oDomNode, oOperation);
Node oChild = oDomNode.getFirstXMLChild();
int eOpType = WSDLOperation.OP_TYPE_UNKNOWN;
while (oChild != null) {
if (oChild instanceof Element) {
Element oElementChild = (Element) oChild;
String aChildLocalName = oElementChild.getLocalName();
if (aChildLocalName == WSDL.INPUT) {
if (eOpType == WSDLOperation.OP_TYPE_NOTIFICATION) {
eOpType = WSDLOperation.OP_TYPE_SOLICIT_RESPONSE;
break;
}
eOpType = WSDLOperation.OP_TYPE_ONE_WAY;
} else if (aChildLocalName == WSDL.OUTPUT) {
if (eOpType == WSDLOperation.OP_TYPE_ONE_WAY) {
eOpType = WSDLOperation.OP_TYPE_REQUEST_RESPONSE;
break;
}
eOpType = WSDLOperation.OP_TYPE_NOTIFICATION;
}
}
oChild = oChild.getNextXMLSibling();
}
oOperation.setOperationType (eOpType);
oNode = oOperation;
}
else if (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_BINDING) {
WSDLBindingOperation poBindingOperation = new WSDLBindingOperation (WSDLDocument.this, oDomNode);
setStandardNodeProps (oDomNode, poBindingOperation);
oNode = poBindingOperation;
}
CreateInputOutputEnumerator enumerator = new CreateInputOutputEnumerator (oDomNode, oNode);
enumerator.enumerate();
if (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_PORTTYPE) {
// set the names of the input and output nodes if none is provided
if (oNode instanceof WSDLOperation) {
WSDLOperation oOperation = (WSDLOperation) oNode;
WSDLNode oInput = oOperation.getWSDLChildNode (WSDLNode.WSDL_INPUT, "");
if ((oInput != null) && StringUtils.isEmpty (oInput.getWSDLName())) {
oInput.setWSDLName (oOperation.getInputName());
}
WSDLNode oOutput = oOperation.getWSDLChildNode (WSDLNode.WSDL_OUTPUT, "");
if ((oOutput != null) && StringUtils.isEmpty (oOutput.getWSDLName())) {
oOutput.setWSDLName (oOperation.getOutputName());
}
}
}
} else if ((oDomNode.getNS() == WSDL.SOAP) && (aLocalName == WSDL.BINDING)) {
if (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_BINDING) {
// this is a "soap:binding" element.
// verify that the parent is a binding element
oNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_BINDING);
}
}
return oNode;
}
}
private class CreatePortEnumerator extends NodeEnumerator {
CreatePortEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element domChild) {
String aLocalName = domChild.getLocalName();
WSDLNode oWSDLNode = null;
if (aLocalName == WSDL.PORT) {
oWSDLNode = createStandardNode (domChild, WSDLNode.WSDL_PORT);
}
CreatePortSubEnumerator enumerator = new CreatePortSubEnumerator (domChild, oWSDLNode);
enumerator.enumerate();
return oWSDLNode;
}
}
private class CreatePortSubEnumerator extends NodeEnumerator {
CreatePortSubEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element domChild) {
String aLocalName = domChild.getLocalName();
WSDLNode oWSDLNode = null;
if ((domChild.getNS() == WSDL.SOAP) && (aLocalName == WSDL.ADDRESS)) {
// this is a "soap:address" element.
oWSDLNode = createExtenNode (domChild, WSDLExten.EX_TYPE_SOAP_ADDRESS);
}
return oWSDLNode;
}
}
// part of operation
private class CreateInputOutputEnumerator extends NodeEnumerator {
CreateInputOutputEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element oDomNode) {
String aLocalName = oDomNode.getLocalName();
WSDLNode oParentWSDLNode = getParentWSDLNode();
WSDLNode oWSDLNode = null;
boolean bEnumerateSubChildren = false;
if (aLocalName == WSDL.INPUT) {
oWSDLNode = createStandardNode (oDomNode, WSDLNode.WSDL_INPUT);
bEnumerateSubChildren = true;
} else if (aLocalName == WSDL.OUTPUT) {
oWSDLNode = createStandardNode (oDomNode, WSDLNode.WSDL_OUTPUT);
bEnumerateSubChildren = true;
} else if (aLocalName == WSDL.FAULT) {
oWSDLNode = createStandardNode (oDomNode, WSDLNode.WSDL_FAULT);
bEnumerateSubChildren = true;
} else if ((oDomNode.getNS() == WSDL.SOAP) && (aLocalName == WSDL.OPERATION) && (oParentWSDLNode.getNodeType() == WSDLNode.WSDL_BINDING_OPERATION)) {
// this is a "soap:operation" element.
oWSDLNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_OPERATION);
}
if ((oParentWSDLNode.getNodeType() == WSDLNode.WSDL_BINDING_OPERATION) && bEnumerateSubChildren) {
CreateInputOutputSubEnumerator enumerator = new CreateInputOutputSubEnumerator (oDomNode, oWSDLNode);
enumerator.enumerate();
}
return oWSDLNode;
}
}
private class CreateInputOutputSubEnumerator extends NodeEnumerator {
CreateInputOutputSubEnumerator (Element domNode, WSDLNode parentWSDLNode) {
super (domNode, parentWSDLNode);
}
WSDLNode processNode (Element oDomNode) {
String aLocalName = oDomNode.getLocalName();
WSDLNode oWSDLNode = null;
if (oDomNode.getNS() == WSDL.SOAP) {
if (aLocalName == WSDL.BODY) {
// this is a "soap:body" element.
oWSDLNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_BODY);
} else if (aLocalName == WSDL.HEADER) {
// this is a "soap:header" element.
oWSDLNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_HEADER);
} else if (aLocalName == WSDL.HEADERDEFAULT) {
// this is a "soap:header" element.
oWSDLNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_HEADER_DEFAULT);
} else if ((aLocalName == WSDL.FAULT) && (getParentWSDLNode().getNodeType() == WSDLNode.WSDL_FAULT)) {
// this is a "soap:fault" element.
oWSDLNode = createExtenNode (oDomNode, WSDLExten.EX_TYPE_SOAP_FAULT);
}
}
return oWSDLNode;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy