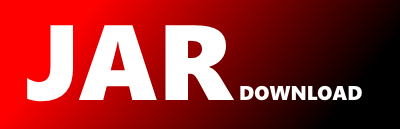
com.adobe.xmp.schema.model.PropertyDescription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
// =================================================================================================
// ADOBE SYSTEMS INCORPORATED
// Copyright 2011 Adobe Systems Incorporated
// All Rights Reserved
//
// NOTICE: Adobe permits you to use, modify, and distribute this file in accordance with the terms
// of the Adobe license agreement accompanying it.
// =================================================================================================
package com.adobe.xmp.schema.model;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.xml.namespace.QName;
/**
* Describes an XMP property that can live either in an SchemaDescription
* as top-level property or or in an StructType
as a struct field.
* When added to a schema description it has to be in the same namespace as the parent schema.
*
* @author Stefan Makswit
*/
public interface PropertyDescription extends Serializable
{
/**
* The namespace URI of the property.
* While all properties of a schema have to be in the same namespace,
* a struct can contain properties of different namespaces.
*
* @return Returns the namespace URI
*/
String getNamespaceURI();
/**
* @param namespaceURI the namespace to set
*/
void setNamespaceURI(String namespaceURI);
/**
* @return Returns the property name. It is a local name in the context of XML namespaces.
*/
String getName();
/**
* @return Returns the type of the property. Can be simple, array or struct.
*/
PropertyType getType();
/**
* Replaces an UnspecifiedType with a final PropertyType.
* This method is only needed by schema parsers.
*
* @param finalType the type that replaces the unspecified type.
*/
void replaceType(PropertyType finalType);
// =============
// Qualifier API
// =============
/**
* Add a qualifier to the property.
* A qualifier behaves exactly like a normal XMP property.
* A property must not have two qualifiers that have the same QName.
*
* @param qualifier the qualifier to add.
*/
void addQualifier(PropertyDescription qualifier);
/**
* Gets a qualifier by namespace and local name if existing.
*
* @param namespaceURI the qualifier's namespace
* @param localName the qualifier's local name
* @return Returns the qualifier or null
if not existing.
*/
PropertyDescription getQualifier(String namespaceURI, String localName);
/**
* @return Returns the collection of all qualifiers
*/
List getQualifiers();
/**
* Removes a qualifier by namespace and local name.
*
* @param namespaceURI the qualifier's namespace
* @param localName the qualifier's local name
*/
void removeQualifier(String namespaceURI, String localName);
/**
* @return Returns true if the property has at least on qualifier.
*/
boolean hasQualifiers();
// ==============
// Validation API
// ==============
/**
* The mandatory flag denotes that a property has to be specified in an XMP data object.
* This applies only when the schema is specified at all.
* It does not indicate that this property has to specified in each XMP data set.
*
* @return Returns true if the property is mandatory.
*/
boolean isMandatory();
/**
* @param mandatory the mandatory flag to set
*/
void setMandatory(boolean mandatory);
/**
* Denotes that this property shall not be used anymore,
* while it is still accepted. A validator could generate a warning
* when coming across a deprecated property.
*
* @return Returns the deprecated flag.
*/
boolean isDeprecated();
/**
* @param deprecated the deprecated flag to set
*/
void setDeprecated(boolean deprecated);
// ========
// UI Hints
// ========
/**
* @return Returns the default label for the property.
*/
String getLabel();
/**
* @param label Sets the default label
*/
void setLabel(String label);
/**
* @return Returns the default description for this property.
*/
String getDescription();
/**
* @param description Sets the default description
*/
void setDescription(String description);
/**
* Read-only is a hint for UI generators and indicate that the property should not be modified
* by the user, and is rather maintained by the application.
* This flag is similar to the internal (read-only) / external (read/write) term that we used
* in our spec.
* Note: this flag is under discussion.
*
* @return Returns true if the property is read-only, and false if the property may be edited.
*/
boolean isReadOnly();
/**
* @param readOnly Sets the readonly flag
*/
void setReadOnly(boolean readOnly);
/**
* @param schemaVisitor a visitor that implements the SchemaVisitor
-interface
*/
void accept(SchemaVisitor schemaVisitor) throws XMPSchemaException;
// =============
// Decorator API
// =============
/**
* Gets a user defined decorator map.
* To add decorators, get the map and add property-value pairs.
* It is implicitly created, when empty.
*
* @param namespaceURI namespace for the decorator tag
* @param localName name of the decorator tag
* @return Returns a decorator map. It may be empty, but is never null
.
*/
Map getDecorator(String namespaceURI, String localName);
/**
* Request if a decorator exists AND contains at least one property.
* Unlike the get-method, this method does not create
* an empty decorator when it has not existed before.
*
* @param namespaceURI namespace for the decorator tag
* @param localName name of the decorator tag
* @return Returns whether a decorator exists or not.
*/
boolean hasDecorator(String myNamespace, String myDecorator);
/**
* @return Returns true when the property contains at least one decorator.
*
*/
boolean hasDecorators();
/**
* Retrieves a list of all decorators, sorted by QName.
* To get the values the method {@link #getDecorator(String, String)}.
*
* @return Returns a list of QNames of all decorators
*/
Set getDecoratorSet();
/**
* Removes all decorators from one namespace.
* @param namespaceURI a decorator namespace URI
*/
void removeDecoratorNS(String namespaceURI);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy