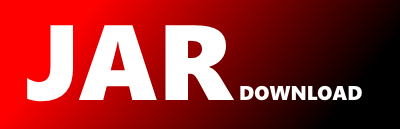
com.adobe.xmp.schema.rng.model.ParamInfoImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: ParamInfo.java
* ************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.xmp.schema.rng.model;
import com.adobe.xmp.schema.rng.parser.constants.RNGSymbol;
/**
* This class defines a single constraint on a property value
*
* @author pwollek
*/
public class ParamInfoImpl implements ParamInfo
{
private final RNGSymbol mType;
private final Object mValue;
private String mValueType;
private final String mLabel;
/**
*
* Constructs a new ParamInfoImpl.
*
* @param type
* Type
* @param value
* Value defined from RNG schema
* @param label
* Label used
*/
public ParamInfoImpl(String type, Object value, String valueType, String label)
{
mType = RNGSymbol.toSymbol(type);
mValue = value;
mValueType = valueType;
mLabel = label;
}
/**
*
* Constructs a new ParamInfoImpl.
*
* @param type
* Type
* @param value
* Value defined from RNG schema
*/
public ParamInfoImpl(String type, Object value)
{
this(type, value, null, "");
}
/**
* @return the mType
*/
public RNGSymbol getType()
{
return mType;
}
/**
* @return the mValue
*/
public Object getValue()
{
return mValue;
}
/**
* @return Returns the literal type of the parameter, e.g.
* 1
*/
public String getValueType()
{
return mValueType;
}
/**
* @return Return the XMP value type or null
if there is no match.
*/
public String getXMPValueType()
{
return DatatypeInfo.kXMLDatatypes.get(mValueType);
}
/**
* @return the mValue
*/
public String getStringValue()
{
return (mValue instanceof String) ? (String) mValue : null;
}
public boolean equals(ParamInfo param)
{
boolean ret = false;
if (param instanceof ParamInfoImpl)
{
ParamInfoImpl info = (ParamInfoImpl) param;
ret = (mType == info.mType && mValue.equals(info.mValue));
}
return ret;
}
/**
* @return the mLabel
*/
public String getLabel()
{
return mLabel;
}
@Override
public String toString()
{
StringBuilder str = new StringBuilder();
str.append("\nParamInfo : (" + mType + ", ");
str.append(mValue + ", ");
str.append(mValueType + ", ");
str.append(mLabel + " )");
return str.toString();
}
/**
* Constant ParamInfoImpl for
*/
public static final ParamInfoImpl PARAM_ALL_TEXT = new ParamInfoImpl("text", null);
/**
* Constant ParamInfoImpl for
*/
public static final ParamInfoImpl PARAM_ALL_INTEGER = new ParamInfoImpl("INTEGER", null);
/**
* Constant ParamInfoImpl for
*/
public static final ParamInfoImpl PARAM_ALL_DOUBLE = new ParamInfoImpl("DOUBLE", null);
/**
* Constant ParamInfoImpl for
*/
public static final ParamInfoImpl PARAM_ALL_BOOLEAN = new ParamInfoImpl("BOOLEAN", null);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy