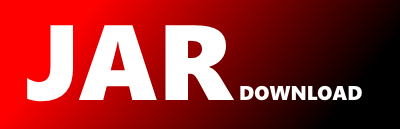
com.day.cq.analytics.sitecatalyst.SitecatalystWebservice Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.analytics.sitecatalyst;
import org.apache.sling.commons.json.JSONArray;
import org.apache.sling.commons.json.JSONObject;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.wcm.webservicesupport.Configuration;
@ProviderType
public interface SitecatalystWebservice {
/** Property name for company */
static final String PN_COMPANY = "company";
/** Property name for username */
static final String PN_USERNAME = "username";
/** Property name for secret */
static final String PN_SECRET = "secret";
/** Property name for server */
static final String PN_SERVER = "server";
/** Property name for password */
static final String PN_PASSWORD = "password";
/** Property name for report suite */
static final String PN_REPORTSUITE = "reportsuite";
/** Property name for report suites */
static final String PN_REPORTSUITES = "reportsuites";
/**
* Checks SiteCatalyst credentials by issuing the API call
* Company.GetReportSuites.
*
* @param company Company
* @param username Username
* @param password Password
* @throws SitecatalystException - if an error occurs, authentication or
* authorization fails.
*/
@Deprecated
void checkCredentials(String company, String username, String password) throws SitecatalystException;
/**
* Returns the login key (shared secret) for the provided company
,username
* and password
combination.
*
* @param company Company
* @param username Username
* @param password Password
* @return login key (shared secret) for the provided {@code company},{@code username}
* and {@code password} combination.
* @throws SitecatalystException {@link SitecatalystException}
*/
@Deprecated
String getLoginKey(String company, String username, String password) throws SitecatalystException;
/**
* Returns the login key (shared secret) for the provided
* company
,username
and password
* combination.
*
* @param server Server end point URL
* @param company Company
* @param username Username
* @param password Password
* @return login key (shared secret) for the provided {@code company},{@code username}
* and {@code password} combination.
* @throws SitecatalystException {@link SitecatalystException}
*/
@Deprecated
String getLoginKey(String server, String company, String username, String password) throws SitecatalystException;
/**
* Returns a JSON representation of report suites for a
* {@link Configuration}.
*
* @param configuration Web service support configuration
* @return JSON string
* @throws SitecatalystException {@link SitecatalystException}
*/
String getReportSuites(Configuration configuration) throws SitecatalystException;
/**
* Gets all available segments using the required Analytics parameters. This method can be used when the Analytics configuration is not
* available yet.
* @param server the Analytics data center to be used for the API call
* @param company the Analytics company
* @param username the Analytics user name
* @param secret the Analytics webservice API shared secret
* @return a stringified JSON list of all the available Analytics segments
* @throws SitecatalystException if something goes wrong while calling the Analytics API for getting the segments
*/
String getSegments(String server, String company, String username, String secret) throws SitecatalystException;
/**
* Returns the tracking server URI for a specified ReportSuiteID (
* rsid
).
*
* @param configuration Web service support configuration
* @param rsid Report suite ID
* @return the tracking server URI for specified {@code rsid}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getTrackingServer(Configuration configuration, String rsid) throws SitecatalystException;
/**
* Returns a JSON representation of conversion variables (eVar's) for a
* report suite rsid
.
*
* @param configuration Web service support configuration
* @param rsid Report suite ID
* @return JSON representation of conversion variables for {@code rsid}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getEvars(Configuration configuration, String rsid) throws SitecatalystException;
/**
* Returns a JSON representation of traffic variables (prop's) for a report
* suite rsid
.
*
* @param configuration Web service support configuration
* @param rsid Report suite ID
* @return TrafficVars for {@code rsid}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getTrafficVars(Configuration configuration, String rsid) throws SitecatalystException;
/**
* Queues a report of type
* reportType
with the reportDescription
.
*
* @param configuration Web service support configuration
* @param reportType The type of report to queue
* @param reportDescription The reportDescription JSON to use
* @return report of type {@code reportType}
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.6
* @deprecated Analytics API 1.4 no longer requires a report type as parameter for queueing reports.
* Use {@link SitecatalystWebservice#queueReport(Configuration, JSONObject)} instead.
*/
@Deprecated
String queueReport(Configuration configuration, String reportType, JSONObject reportDescription)
throws SitecatalystException;
/**
* Queues the execution of an Analytics report based on a given JSON reportDescription
.
*
* @param configuration Web service support configuration
* @param reportDescription a reportDescription JSON object that specifies the desired report contents
* @return a stringified JSON containing the ID of the queued report
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.13.0
*/
String queueReport(Configuration configuration, JSONObject reportDescription) throws SitecatalystException;
/**
* Queues a report of type pageView
for a report suite
* rsid
and the report date date
*
* @param configuration Web service support configuration
* @param rsid Report suite ID
* @param date Date
* @return report of type {@code pageView}
* @throws SitecatalystException {@link SitecatalystException}
*/
String queuePageViewReport(Configuration configuration, String rsid, String date) throws SitecatalystException;
/**
* Returns status of a report with report identifier reportID
.
*
* @param configuration Web service support configuration
* @param reportID report ID
* @return status of report with identifieer {@code reportID}
* @deprecated Use {@link #getReport(Configuration, String)} instead.
* @throws SitecatalystException {@link SitecatalystException}
*/
@Deprecated
String getReportStatus(Configuration configuration, String reportID) throws SitecatalystException;
/**
* Returns a report with report identifier reportID
.
*
* @param configuration Web service support configuration
* @param reportID Report ID
* @return report with report identifier {@code reportID}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getReport(Configuration configuration, String reportID) throws SitecatalystException;
/**
* Returns a report of type reportType
and report description
* reportDescription
.
*
* @param configuration Web service support configuration
* @param reportType the type of report to queue
* @param reportDescription the reportDescription json to use
* @return report of type {@code reportType} and report description
* {@code reportDescription}
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.6
* @deprecated The Analytics API no longer supports synchronous reports starting with the 1.4 version.
*/
@Deprecated
String getSynchronousReport(Configuration configuration, String reportType, JSONObject reportDescription)
throws SitecatalystException;
/**
* Retrieves a list of possible valid elements for a report.
* Some accounts may not have access to certain metrics.
* The metrics returned by GetMetrics reflect those restrictions.
*
* See more information on this API method at
* https://experiencecloud.adobe.com/developer/en_US/documentation/analytics-reporting-1-4/r-getmetrics
*
* @param configuration Web service support configuration
* @param rsID The reporting suite id
* @return response {@link String}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getMetrics(Configuration configuration, String rsID) throws SitecatalystException;
/**
* Returns a JSON representation of success events (event's) for the report
* suite rsid
.
*
* @param configuration Web service support configuration
* @param rsid Report suite ID
* @return JSON representation of success events for {@code rside}
* @throws SitecatalystException {@link SitecatalystException}
*/
String getSuccessEvents(Configuration configuration, String rsid) throws SitecatalystException;
/**
* Returns a JSON representation of pages matching the page name
* pagename
for the report suite rsid
.
*
* @param configuration Web service support configuration
* @param limit Max. number of pages
* @param pagename Name of the page
* @param rsid Report Suite ID
* @param start Start point for paging
* @return list of pages for {@code rsid}
* @throws SitecatalystException {@link SitecatalystException}
* @deprecated The Analytics API no longer supports retrieving a list of pages from specified report suite starting with the 1.4 version.
*/
@Deprecated
String getPages(Configuration configuration, Integer limit, String pagename, String rsid, Integer start)
throws SitecatalystException;
/**
* Retrieves a list of classifications (associated with the specified
* element) for each of the specified reportSuites
.
*
* @param configuration Web service support configuration
* @param classificationView The ID of the element whose classifications are
* being retrieved. The possible type IDs include the following elements.
*
* campaign
Classification applied to the Campaign report.
* days_between_buys
Classification applied to the Days Between Buys report.
* days_till_purchase
Classification applied to the Days Until Purchase report.
* domain
Classification applied to the Domain report.
* evar1-75
Classification applied to the specified eVar report (evar1, evar2, etc.)
* first_touch_marketing_channel
Classification applied to the First Touch Marketing Channel report.
* first_touch_marketing_channel_detail
Classification applied to the detailed First Touch Marketing Channel report.
* last_touch_marketing_channel
Classification applied to the Last Touch Marketing Channel report.
* last_touch_marketing_channel_detail
Classification applied to the detailed Last Touch Marketing Channel report.
* loyalty
Classification applied to the Loyalty report.
* media
Classification applied to the Media report.
* page
Classification applied to the Page report.
* page_type1-77
Classification applied to a specific Page Type report.
* page_type1
Site Sections
* page_type2
Servers
* page_type3-77
Custom Insight (Props) 1 - 75.
* mvvar45-47
Classification applied to a List Var report, mvvar45 - mvvar47 = listvar1 - listvar3.
* product
Classification applied to the Product report.
* sitetime
Classification applied to the Site Time report.
* state
Classification applied to the State report.
* survey
Classification applied to the Survey report.
* tnt
Classification applied to the Adobe Target report.
* visitdepth
Classification applied to the Visit Depth report.
* visitnum
Classification applied to the VisitNum report.
* zipcode
Classification applied to the Zip Code report.
*
* @param reportSuites A list of report suite IDs.
* @param relationId (Optional) Numeric ID of the variable for which you want to retrieve associated classifications or -1.
* @return A JSONArray of JSONObjects containing information about a report suite's classifications.The
* objects are of the following structure:
*
*
* {
* rsid: (int),
* site_title: (string),
* classifications: [{
* classifications: [{
* child_nodes: [{
* rel_id: (int),
* userid: (int),
* div_name: (string),
* div_num: (int),
* parent_div_num: (int),
* campaign_view_flag: (int),
* type: (int),
* order: (int),
* child_nodes: (array)
* }],
* div_name: (string),
* div_num: (int),
* rel_id: (int)
* }],
* in_queue: (int),
* status: (int)
* }]
* }
*
*
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.7
* @deprecated This method was used to support SAINT which is now deprecated.
*/
@Deprecated
JSONArray getClassifications(Configuration configuration, String classificationView, String[] reportSuites, int relationId) throws SitecatalystException;
/**
* Saves a classification for each of the specified
* reportSuites
.
*
* @param configuration Web service support configuration
* @param classificationView The ID of the element whose classifications are
* being retrieved. The possible type IDs include the following elements.
*
* campaign
Classification applied to the Campaign report.
* days_between_buys
Classification applied to the Days Between Buys report.
* days_till_purchase
Classification applied to the Days Until Purchase report.
* domain
Classification applied to the Domain report.
* evar1-75
Classification applied to the specified eVar report (evar1, evar2, etc.)
* first_touch_marketing_channel
Classification applied to the First Touch Marketing Channel report.
* first_touch_marketing_channel_detail
Classification applied to the detailed First Touch Marketing Channel report.
* last_touch_marketing_channel
Classification applied to the Last Touch Marketing Channel report.
* last_touch_marketing_channel_detail
Classification applied to the detailed Last Touch Marketing Channel report.
* loyalty
Classification applied to the Loyalty report.
* media
Classification applied to the Media report.
* page
Classification applied to the Page report.
* page_type1-77
Classification applied to a specific Page Type report.
* page_type1
Site Sections
* page_type2
Servers
* page_type3-77
Custom Insight (Props) 1 - 75.
* mvvar45-47
Classification applied to a List Var report, mvvar45 - mvvar47 = listvar1 - listvar3.
* product
Classification applied to the Product report.
* sitetime
Classification applied to the Site Time report.
* state
Classification applied to the State report.
* survey
Classification applied to the Survey report.
* tnt
Classification applied to the Adobe Target report.
* visitdepth
Classification applied to the Visit Depth report.
* visitnum
Classification applied to the VisitNum report.
* zipcode
Classification applied to the Zip Code report.
*
* @param campaignView Determines whether to treat this classification like
* a campaign.
* @param name Visible name of the classification.
* @param parentDivNum Contains the parent classification's
* div_num
if this is a sub-classification;
* otherwise it is 0.
* @param reportSuites A list of report suite IDs.
* @param update Enables the classification when set to true
,
* disabled it otherwise.
* @return Returns true
if the update operation is successful,
* false
otherwise
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.7
* @deprecated This method was used to support SAINT which is now deprecated.
*/
@Deprecated
boolean saveClassifications(Configuration configuration, String classificationView, boolean campaignView,
String name, int parentDivNum, String[] reportSuites, boolean update) throws SitecatalystException;
/**
* Deletes a classification from one or more reportSuites
.
*
* @param configuration Web service support configuration
* @param classificationView The ID of the element whose classifications are
* being retrieved. The possible type IDs include the following elements.
*
* campaign
Classification applied to the Campaign report.
* days_between_buys
Classification applied to the Days Between Buys report.
* days_till_purchase
Classification applied to the Days Until Purchase report.
* domain
Classification applied to the Domain report.
* evar1-75
Classification applied to the specified eVar report (evar1, evar2, etc.)
* first_touch_marketing_channel
Classification applied to the First Touch Marketing Channel report.
* first_touch_marketing_channel_detail
Classification applied to the detailed First Touch Marketing Channel report.
* last_touch_marketing_channel
Classification applied to the Last Touch Marketing Channel report.
* last_touch_marketing_channel_detail
Classification applied to the detailed Last Touch Marketing Channel report.
* loyalty
Classification applied to the Loyalty report.
* media
Classification applied to the Media report.
* page
Classification applied to the Page report.
* page_type1-77
Classification applied to a specific Page Type report.
* page_type1
Site Sections
* page_type2
Servers
* page_type3-77
Custom Insight (Props) 1 - 75.
* mvvar45-47
Classification applied to a List Var report, mvvar45 - mvvar47 = listvar1 - listvar3.
* product
Classification applied to the Product report.
* sitetime
Classification applied to the Site Time report.
* state
Classification applied to the State report.
* survey
Classification applied to the Survey report.
* tnt
Classification applied to the Adobe Target report.
* visitdepth
Classification applied to the Visit Depth report.
* visitnum
Classification applied to the VisitNum report.
* zipcode
Classification applied to the Zip Code report.
*
* @param reportSuites A list of report suite IDs.
* @param divNum The numeric index of the classification in its group, which
* can be obtained from
* {@link #getClassifications(Configuration, String, String[], int)}
* @param parentDivNum Contains the parent classification's div_num if this
* is a sub-classification; otherwise it is 0.
* @return Returns true
if the deletion operation is
* successful, false
otherwise.
* @throws SitecatalystException {@link SitecatalystException}
* @since 5.7
* @deprecated This method was used to support SAINT which is now deprecated.
*/
@Deprecated
boolean deleteClassifications(Configuration configuration, String classificationView, String[] reportSuites,
int divNum, int parentDivNum) throws SitecatalystException;
}