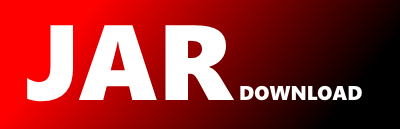
com.day.cq.analytics.sitecatalyst.util.RelativeDateFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.analytics.sitecatalyst.util;
import java.text.DateFormatSymbols;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Locale;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
@SuppressWarnings("serial")
public class RelativeDateFormat extends SimpleDateFormat {
protected Date base;
protected static final String RELATIVE_DATE_REGEX = "([+-]\\d+)([dDmMyY])";
protected Pattern pattern;
public RelativeDateFormat() {
super();
this.pattern = Pattern.compile(RELATIVE_DATE_REGEX);
base = new Date();
}
public RelativeDateFormat(String pattern) {
super(pattern);
this.pattern = Pattern.compile(RELATIVE_DATE_REGEX);
base = new Date();
}
public RelativeDateFormat(String pattern, DateFormatSymbols formatSymbols) {
super(pattern, formatSymbols);
this.pattern = Pattern.compile(RELATIVE_DATE_REGEX);
base = new Date();
}
public RelativeDateFormat(String pattern, Locale locale) {
super(pattern, locale);
this.pattern = Pattern.compile(RELATIVE_DATE_REGEX);
base = new Date();
}
public Date parseRelative(String text) throws ParseException {
Matcher matcher = pattern.matcher(text);
if(matcher.matches()) {
// integer parsing does not work with leading +
int amount = Integer.parseInt(matcher.group(1).replace("+", ""));
String unit = matcher.group(2).toLowerCase();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(this.base);
int field = 0;
if(unit.equals("d"))
field = GregorianCalendar.DAY_OF_MONTH;
else if(unit.equals("m"))
field = GregorianCalendar.MONTH;
else if(unit.equals("y"))
field = GregorianCalendar.YEAR;
cal.add(field, amount);
return cal.getTime();
}else return this.parse(text);
}
public void setBaseDate(Date base) {
if(base != null)
this.base = base;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy