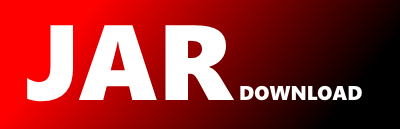
com.day.cq.analytics.testandtarget.PerformanceReportItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.analytics.testandtarget;
/**
* The {@code PerformanceReportItem} is an entry in a {@link PerformanceReport}
*/
public class PerformanceReportItem {
private String experienceName;
private int entryCount;
private int conversionCount;
private double conversionRate;
private double lift;
private double confidence;
private double engagement;
private double engagementTotal;
private int engagementCount;
private String revenuePerVisitor;
private String averageOrderValue;
private String totalSales;
/**
* Returns the name of the experience
*
* @return the name of the experience, possibly null
if this item represents the aggregated report data
*/
public String getExperienceName() {
return experienceName;
}
/**
* Sets the specified {@code experienceName}.
*
* @param experienceName string representation of the experience name
*/
public void setExperienceName(String experienceName) {
this.experienceName = experienceName;
}
/**
* Returns the number of entries for this item.
*
* @return the number of entries
*/
public int getEntryCount() {
return entryCount;
}
/**
* Sets the number of entries for this item.
*
* @param entryCount the number of entries
*/
public void setEntryCount(int entryCount) {
this.entryCount = entryCount;
}
/**
* Returns the number of conversions for this item.
*
* @return the number of conversions
*/
public int getConversionCount() {
return conversionCount;
}
/**
* Sets the number of conversions for this item.
*
* @param conversionCount the number of conversions
*/
public void setConversionCount(int conversionCount) {
this.conversionCount = conversionCount;
}
/**
* Getter and setter for the conversion rate field.
*
* @return the conversion rate
*/
public double getConversionRate() { return conversionRate; }
/**
* Sets the conversion rate.
*
* @param conversionRate conversion rate
*/
public void setConversionRate(double conversionRate) { this.conversionRate = conversionRate; }
/**
* Gets the lift value.
*
* @return the lift
*/
public double getLift() { return lift; }
/**
* Sets the lift value.
*
* @param lift the lift
*/
public void setLift(double lift) { this.lift = lift; }
/**
* Gets the confidence.
*
* @return the confidence
*/
public double getConfidence() { return confidence; }
/**
* Sets the confidence.
*
* @param confidence the confidence
*/
public void setConfidence(double confidence) {this.confidence = confidence; }
/**
* Gets the engagement.
*
* @return the engagement
*/
public double getEngagement() { return engagement; }
/**
* Sets the engagement.
*
* @param engagement the engagement
*/
public void setEngagement(double engagement) {this.engagement = engagement; }
/**
* Gets the engagement count.
*
* @return the engagement count
*/
public int getEngagementCount() { return engagementCount; }
/**
* Gets the engagement count.
*
* @param engagementCount the engagement count
*/
public void setEngagementCount(int engagementCount) {this.engagementCount = engagementCount; }
/**
* Gets the engagement total.
*
* @return the engagement total
*/
public double getEngagementTotal() { return engagementTotal; }
/**
* Sets the engagement total.
*
* @param engagementTotal the engagement total
*/
public void setEngagementTotal(double engagementTotal) { this.engagementTotal = engagementTotal; }
/**
* Gets the revenue per visitor.
*
* @return the revenue per visitor
*/
public String getRPV() { return revenuePerVisitor; }
/**
* Sets the revenue per visitor.
*
* @param revenuePerVisitor the revenue per visitor
*/
public void setRPV(String revenuePerVisitor) {this.revenuePerVisitor = revenuePerVisitor; }
/**
* Gets the average order value.
*
* @return the average order value
*/
public String getAOV() { return averageOrderValue; }
/**
* Sets the average order value.
*
* @param averageOrderValue the average order value
*/
public void setAOV(String averageOrderValue) {this.averageOrderValue = averageOrderValue; }
/**
* Gets the total sales.
*
* @return the total sales
*/
public String getTotalSales() { return totalSales; }
/**
* Sets the total sales.
*
* @param totalSales the total sales
*/
public void setTotalSales(String totalSales) {this.totalSales = totalSales; }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy