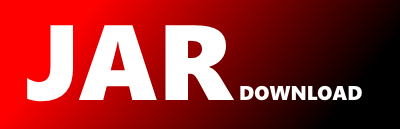
com.day.cq.analytics.testandtarget.TestandtargetService Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.analytics.testandtarget;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.analytics.testandtarget.impl.TestandtargetCampaign;
import com.day.cq.analytics.testandtarget.util.CampaignType;
import com.day.cq.wcm.webservicesupport.Configuration;
/**
* The {@code TestandtargetService} is the entry point for all the operations which interact with the
* Adobe Target API
*
* This service is able to perform operations on both Adobe Target entry points - XML and REST. While
* care has been taken to make the API backend transparent, to the caller, there are some methods where there are
* small differences, usually due the XML API providing less information than the REST API. These limitations
* are clearly documented in the method-level javadocs.
*
* As such, clients of this service are encouraged to use the REST API rather than XML API. This setting is
* a property on the cloud service configuration and is properly saved by the cloud configuration UI.
*
* @see TestandtargetCampaignMediator
* @deprecated This service will be removed in future versions. No replacement will be provided.
*/
@ProviderType
@Deprecated
public interface TestandtargetService {
/** folder list operation */
String OPERATION_FOLDER_LIST = "folderList";
/** campaign list operation */
String OPERATION_CAMPAIGN_LIST = "campaignList";
/** HTML offer save operation */
String OPERATION_SAVE_HTML_OFFER = "saveHtmlOfferContent";
/** HTML offer get operation */
String OPERATION_GET_HTML_OFFER = "getHtmlOfferContent";
/** HTML offer list operation */
String OPERATION_HTML_OFFER_LIST = "offerList";
/** Widget offer deletion operation */
String OPERATION_DELETE = "deleteWidgetOffer";
/** Widget offer save operation */
String OPERATION_SAVE = "saveWidgetOffer";
/** save operation */
String OPERATION_SET_CAMPAIGN_STATE = "setCampaignState";
/** Campaign create operation */
String OPERATION_SAVE_CAMPAIGN = "saveCampaign";
String OPERATION_REPORT = "report";
/** Property name E-Mail */
String PN_EMAIL = "email";
/** Property name password */
String PN_PASSWORD = "password";
String CAMPAIGN_STATE_APPROVED = "Approved";
String CAMPAIGN_STATE_DEACTIVATED = "Deactivated";
/** Property name client code */
String PN_CLIENTCODE = "clientcode";
/** Property name tenant id */
String PN_TENANTID = "tenantId";
/** Property name authentication */
String PN_AUTHENTICATION = "authentication";
/**
* Returns a tree of folders available in Adobe Target.
* Folders are not supported when using REST API.
*
* @param configuration Service {@link Configuration}
* @return The tree of folders available in Adobe Target. This method returns <code>null</code>
* when using Adobe Target REST API
* @throws TestandtargetException {@link TestandtargetException} on all errors
*/
Folder listFolders(Configuration configuration) throws TestandtargetException;
/**
* Creates a widget offer on Adobe Target.
*
* @param configuration
* Service {@link Configuration}
* @param name
* Name of the widget offer
* @param url
* The encoded URL of the third-party server that hosts the
* dynamically generated response
* @param id
* The third-party ID of the widget offer
* @return A string representation of the third-party id
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
String publishOffer(Configuration configuration, String name, String url, String id) throws TestandtargetException;
/**
* Deletes a widget offer on Adobe Target.
*
* @param configuration
* Service {@link Configuration}
* @param name
* Name of the widget offer
* @param url
* The encoded URL of the third-party server that hosts the
* dynamically generated response
* @param id
* The third-party ID of the widget offer
* @return A string representation of the third-party id
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
String unpublishOffer(Configuration configuration, String name, String url, String id) throws TestandtargetException;
/**
* Creates a widget offer.
*
* @param configuration Service {@link Configuration}
* @param name Name of the widget offer
* @param url The encoded URL of the third-party server that hosts the
* dynamically generated response
* @param id The third-party ID of the widget offer
* @return A string representation of the third-party id
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
String createWidgetOffer(Configuration configuration, String name, String url, String id) throws TestandtargetException;
/**
* Creates an HTML offer.
*
* @param configuration Service {@link Configuration}
* @param offerName Name of the offer
* @param folderId Folder id
* @param content Offer content as plain HTML.
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
void createHTMLOffer(Configuration configuration, String offerName, String folderId, String content) throws TestandtargetException;
/**
* Creates a HTML offer
* @param configuration the cloud-service configuration
* @param request a {@link SaveOfferRequest} object containing the operation's details, such as the offer data.
* This object may be incomplete, i.e. just contain the essential data for creating an offer - name and content
* @return
* the offer id, as set by Adobe Target. If the XML API is used this method return -1
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
long createHTMLOffer(Configuration configuration, SaveOfferRequest request) throws TestandtargetException;
/**
* Retrieves a HTML offer from Adobe Target
*
* @param configuration Service {@link Configuration}
* @param offerName Name of the offer
* @return An HTMLOffer
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
HTMLOffer getHTMLOffer(Configuration configuration, String offerName) throws TestandtargetException;
/**
* Retrieves an offer from Adobe Target
* @param configuration the cloud services configuration for the Adobe Target account
* @param request a {@link ViewOfferRequest} object containing the request data
* @return a {@link ViewOfferResponse} object containing the offer's data
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
ViewOfferResponse getReusableOffer(Configuration configuration, ViewOfferRequest request) throws TestandtargetException;
/**
* Returns a JSON representation of offers.
*
* @param configuration Service {@link Configuration}
* @param folderId Folder id
* @return An {@link Collection} of {@link Offer} objects
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
Collection listOffers(Configuration configuration, String folderId) throws TestandtargetException;
/**
* Returns a list of offers currently registered in Target
*
* @param configuration a reference to the service {@link Configuration}
* @param request a {@link ListOffersRequest} object containing the request details
* @return a collection of {@link ViewOfferResponse} objects.
* @throws TestandtargetException {@link TestandtargetException} on all errors
*/
Collection listOffers(Configuration configuration, ListOffersRequest request) throws TestandtargetException;
/**
* Returns a {@link Map} with campaigns. The Map key contains the campaign
* ID and the Map value the campaign name.
*
* @param configuration
* Service {@link Configuration}
* @param before
* A date value. Includes campaigns that were active at least
* once before the specified date. By default, the before
* parameter is 2100-01-01T00:00.
* @param after
* A date value. Includes campaigns that were active at least
* once after the specified date. By default, the after parameter
* is 1969-00-00T00:00.
* @param environment
* A URL-encoded host group name, as defined in the T&T Tool. By
* default, the environment value is Production.
* @param name
* The campaign name, or portion of the campaign name, that you
* want to match.
* @param state
* Comma-separated list of states to match. Supported values
* include: saved, activated, library. By default, the filtered
* results include all states.
* @param label
* Comma-separated list of labels to match. By default, the
* filtered results include all labels.
* @return Map of campaigns
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
Map listCampaigns(Configuration configuration, Date before, Date after, String environment, String name, String state, String label) throws TestandtargetException;
/**
* Updates the state of the campaign in Adobe Target.
*
* @param configuration
* Service {@link Configuration}
* @param campaignState
* The campaign state , one of {@link #CAMPAIGN_STATE_APPROVED} or {@link #CAMPAIGN_STATE_DEACTIVATED}
* @param testAndTargetCampaignId
* The campaign identifier. You must provide either testAndTargetCampaignId or thirdPartyCampaignId
* @param thirdPartyCampaignId
* The third-party campaign identifier. You must provide either testAndTargetCampaignId or thirdPartyCampaignId
* @return true if the operation succeeded
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
boolean setCampaignState(Configuration configuration, String campaignState, String testAndTargetCampaignId, String thirdPartyCampaignId) throws TestandtargetException;
void checkCredentials(String clientcode, String email, String password) throws TestandtargetException;
/**
* Retrieves the performance report data for a specific campaign
*
*
* All parameters are mandatory, except for {@code campaignId} and {@code thirdPartyId} , out of which exactly one
* must be specified.
*
*
* @param configuration the cloud services configuration associated with the Adobe Target account
* @param reportType a {@link ReportType} object representing the type of report
* @param campaignId the id of the campaign, as defined in Adobe Target
* @param thirdPartyCampaignId the third party id of the campaign
* @param start the start date of the campaign (used as report filter)
* @param end the end date of the campaign (used as a report filter)
* @param resolution the campaign's resolution (hour, day etc.)
* @return performance report data for a specific campaign
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
* @see Adobe Target
* performance report API
*
*/
Reports getPerformanceReport(Configuration configuration, ReportType reportType, String thirdPartyCampaignId,
String campaignId, Date start, Date end, Resolution resolution) throws TestandtargetException;
/**
* Retrieves the performance report data for a specific campaign
*
*
* The data returned by this method always returns visits for the {@link PerformanceReportItem#getEntryCount()}
* method
*
*
*
* Note that it is recommended that the {@code request} is configured with a campaign id rather than a third party
* id. The reason is that when using the REST API an additional call is performed to map the third party id to a
* campaign id.
*
*
* @param configuration the cloud services configuration associated with the Adobe Target account
* @param request the request object holding all data needed to retrieve the performance data
* @return the performance report data, never null
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
PerformanceReport getPerformanceReport(Configuration configuration, PerformanceReportRequest request) throws TestandtargetException;
/**
* Retrieves a list of segments for the specified {@code configuration}
*
*
* Optional filtering parameters may be set in the {@code request} parameter.
*
*
* @param configuration Service {@link Configuration}
* @param request optional request parameters. May be null.
* @return a list of segments, possibly empty, never null
* @throws TestandtargetException {@link TestandtargetException} on all errors
*/
List listSegments(Configuration configuration, ListSegmentsRequest request) throws TestandtargetException;
/**
* Detects the usage of an mbox in multiple activities
* @param configuration a reference to the Cloud Service configuration
* @param mboxName the name of the mbox
* @param mboxUrl the URL of the mbox. "http://localhost" is accepted
* @return a list of campaign names which reference the specified mbox. The return value can be empty, which signals that there are no collisions. It can also be {@code null}, which signals that there is no information about collisions
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
@Deprecated
List getMboxActivityCollisions(Configuration configuration, String mboxName, String mboxUrl) throws TestandtargetException;
/**
* Get the mboxes list for a given configuration
* @param configuration a reference to the Cloud Service configuration
* @return a list of mbox names. The list can be empty if no mboxes are available for the received configuration
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
List listMboxNames(Configuration configuration) throws TestandtargetException;
/**
* Retrieves a campaign from Target using the thirdPartyId property
* @param configuration the {@link Configuration} associated with the Target account
* @param thirdPartyId the thirdPartyId of the campaign
* @param campaignType the type of the campaign - landingPage
or ab
* @return a {@link Campaign} object or null
if the campaign cannot be found in Target
* @throws TestandtargetException {@link TestandtargetException} on all errors
*
*/
Campaign getCampaignByThirdPartyId(Configuration configuration, String thirdPartyId, CampaignType campaignType ) throws TestandtargetException;
/**
* Retrieves a campaign from Target using the id property
* @param configuration the {@link Configuration} associated with the Target account
* @param campaignId the id of the campaign, as received from Target during a previous update
* @param campaignType the type of the campaign - landingPage
or ab
* @return a {@link Campaign} or null
if the campaign cannot be found in Target
* @throws TestandtargetException {@link TestandtargetException} on all errors
*/
@Deprecated
Campaign getCampaignById(Configuration configuration, long campaignId, CampaignType campaignType ) throws TestandtargetException;
}