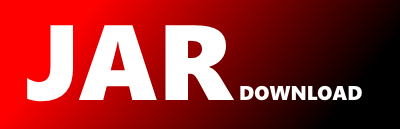
com.day.cq.commons.predicates.AnyPredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.commons.predicates;
import java.io.Serializable;
import java.util.Collection;
import java.util.Iterator;
import java.util.function.Predicate;
public class AnyPredicate implements Predicate, Serializable {
private final Predicate[] predicates;
public static Predicate getInstance(Predicate[] predicates) {
validate(predicates);
if (predicates.length == 0) {
return FalsePredicate.INSTANCE;
} else {
return predicates.length == 1 ? predicates[0] : new AnyPredicate(predicates);
}
}
public static Predicate getInstance(Collection predicates) {
Predicate[] preds = validate(predicates);
if (preds.length == 0) {
return FalsePredicate.INSTANCE;
} else {
return preds.length == 1 ? preds[0] : new AnyPredicate(preds);
}
}
public AnyPredicate(Predicate[] predicates) {
this.predicates = predicates;
}
public boolean test(Object object) {
for (Predicate iPredicate : this.predicates) {
if (iPredicate.test(object)) {
return true;
}
}
return false;
}
public Predicate[] getPredicates() {
return this.predicates;
}
private static void validate(Predicate[] predicates) {
if (predicates == null) {
throw new IllegalArgumentException("The predicate array must not be null");
} else {
for(int i = 0; i < predicates.length; ++i) {
if (predicates[i] == null) {
throw new IllegalArgumentException("The predicate array must not contain a null predicate, index " + i + " was null");
}
}
}
}
private static Predicate[] validate(Collection predicates) {
if (predicates == null) {
throw new IllegalArgumentException("The predicate collection must not be null");
} else {
Predicate[] preds = new Predicate[predicates.size()];
int i = 0;
for(Iterator it = predicates.iterator(); it.hasNext(); ++i) {
preds[i] = (Predicate)it.next();
if (preds[i] == null) {
throw new IllegalArgumentException("The predicate collection must not contain a null predicate, index " + i + " was null");
}
}
return preds;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy