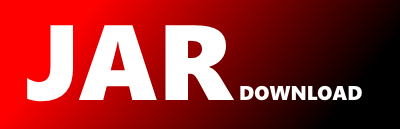
com.day.cq.commons.predicates.servlets.AbstractPredicateServlet Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2024 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.commons.predicates.servlets;
import com.day.cq.commons.predicates.PredicateProvider;
import com.day.cq.commons.predicates.ResourceFilter;
import com.day.cq.commons.predicates.TruePredicate;
import com.day.cq.commons.predicates.AnyPredicate;
import java.util.function.Predicate;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.SlingHttpServletResponse;
import org.apache.sling.api.servlets.SlingAllMethodsServlet;
import org.osgi.service.component.annotations.Reference;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.servlet.ServletException;
import java.io.IOException;
import java.util.Collection;
import java.util.HashSet;
/**
* Abstract servlet that retrieves the predicates from the request.
*/
public abstract class AbstractPredicateServlet extends SlingAllMethodsServlet {
/**
* default path parameter name
*/
public static final String PATH_PARAM = "path";
/**
* default name for the predicate parameter
*/
public static final String PREDICATE_PARAM = "predicate";
/**
* default name for the filter parameter
*/
public static final String FILTER_PARAM = "filter";
/**
* default log
*/
private final Logger log = LoggerFactory.getLogger(AbstractPredicateServlet.class);
@Reference
protected PredicateProvider provider;
/**
* {@inheritDoc}
*
* Calls {@link #doGet(SlingHttpServletRequest, SlingHttpServletResponse, Predicate)}.
*/
@Override
protected void doGet(SlingHttpServletRequest request,
SlingHttpServletResponse response)
throws ServletException, IOException {
doGet(request, response, getPredicate(request));
}
/**
* Handles the HTTP GET method
*
* @param request The HTTP request
* @param response The HTTP response
* @param predicate the predicate retrieved from the request
* @throws ServletException if a servlet error occurs
* @throws IOException if an I/O error occurs
*/
protected void doGet(SlingHttpServletRequest request,
SlingHttpServletResponse response,
Predicate predicate)
throws ServletException, IOException {
handleMethodNotImplemented(request, response);
}
/**
* Returns the index of the first selector that should be considered when
* retrieving the predicates for this servlet.
*
* @return Always {@link Integer#MAX_VALUE}
*/
protected int selectorPredicateStart() {
return Integer.MAX_VALUE;
}
/**
* Returns the predicate retrieved from the given request. the predicates
* are retrieved from the request selectors and the request parameter values
* of the {@value #PREDICATE_PARAM}. those predicates form an
* {@link AnyPredicate}. If no predicates are specified in the request a
* {@link TruePredicate} is returned.
*
* Note: the {@value #FILTER_PARAM} parameters are used to add {@link ResourceFilter}
* predicates. although this is discouraged because java class names should
* never be passed over the wire.
*
* @param request servlet request
* @return predicate
*
*/
public Predicate getPredicate(SlingHttpServletRequest request) {
// get predicates
Predicate predicate = TruePredicate.INSTANCE;
if (provider == null) {
log.warn("Predicate provider not bound.");
} else {
Collection predicates = new HashSet<>();
// add selector predicates
String[] selectors = request.getRequestPathInfo().getSelectors();
for (int i = selectorPredicateStart(); i < selectors.length; i++) {
Predicate p = provider.getPredicate(selectors[i]);
if (p == null) {
log.warn("Unable to retrieve predicate " + selectors[i]);
} else {
predicates.add(p);
}
}
// add request param predicates
if (request.getParameterMap().containsKey(PREDICATE_PARAM)) {
for (String pred : request.getParameterValues(PREDICATE_PARAM)) {
Predicate p = provider.getPredicate(pred);
if (p == null) {
log.warn("Unable to retrieve predicate " + pred);
} else {
predicates.add(p);
}
}
}
// add request param filters
if (request.getParameterMap().containsKey(FILTER_PARAM)) {
ClassLoader loader = this.getClass().getClassLoader();
for (String filter : request.getParameterValues(FILTER_PARAM)) {
try {
predicates.add(new ResourceFilter(loader.loadClass(filter)));
} catch (ClassNotFoundException e) {
log.warn("Requested filter class {} not found.", filter);
}
}
}
if (!predicates.isEmpty()) {
predicate = AnyPredicate.getInstance(predicates);
}
}
return predicate;
}
}