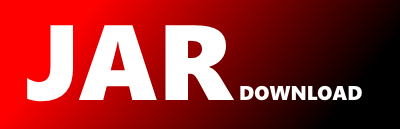
com.day.cq.dam.commons.preset.S7Preset Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2016 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.commons.preset;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.tenant.Tenant;
import javax.jcr.Node;
import javax.jcr.PathNotFoundException;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
public class S7Preset {
private static final String DEFAULT_PRESET_ROOT_PATH = "/libs/settings/dam/dm/";
private static final String TENANTS_BASE_PATH = "tenants/";
private static final String PRESET_BASE_PATH = "/presets/";
private static final String JCR_PRESET_ROOT_PATH = "/etc/dam/dynamicmediaconfig";
private static final String JCR_PRESET_ROOT_PROP = "dynamicMediaPresetRoot";
private PresetType presetType = null;
private String tenantId = "";
private String presetRootPath = "";
/**
*
* @param presetTypeName preset type name defined in PresetType
* @param resourceResolver resource resolver to get tenant information. If null is passed, it is used for out of the box preset path.
*/
public S7Preset(String presetTypeName, ResourceResolver resourceResolver) {
this.presetType = PresetType.getPresetTypeByName(presetTypeName);
this.presetRootPath = DEFAULT_PRESET_ROOT_PATH;
if (resourceResolver != null) {
Tenant tenant = resourceResolver.adaptTo(Tenant.class);
if (tenant != null) {
tenantId = tenant.getId();
this.presetRootPath = getPresetRootPath(resourceResolver);
}
}
}
/**
* Get preset root path
* @param resourceResolver
* @return Preset root path if it is set; otherwise, it returns default root path.
*/
private String getPresetRootPath(ResourceResolver resourceResolver){
String presetRootPath = DEFAULT_PRESET_ROOT_PATH;
try {
Session session = resourceResolver.adaptTo(Session.class);
Node jcrRootPathNode = session.getNode(JCR_PRESET_ROOT_PATH);
if (jcrRootPathNode != null && jcrRootPathNode.hasProperty(JCR_PRESET_ROOT_PROP)) {
presetRootPath = jcrRootPathNode.getProperty(JCR_PRESET_ROOT_PROP).getString();
if (!presetRootPath.endsWith("/")) {
presetRootPath += "/";
}
}
}
catch(PathNotFoundException pnfe) {
}
catch(RepositoryException re){
}
return presetRootPath;
}
/**
* Get preset path based on preset type and tenancy setup
* @return preset root path
*/
public String getPresetPath(){
StringBuilder path = new StringBuilder(this.presetRootPath);
if (!tenantId.isEmpty()) {
path.append(TENANTS_BASE_PATH);
path.append(this.tenantId);
path.append(PRESET_BASE_PATH);
path.append(this.presetType.getName());
}
else {
path.append(this.presetType.getDefaultPresetPath());
}
return path.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy