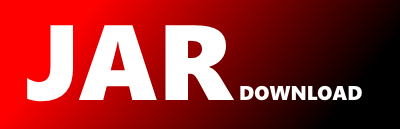
com.day.cq.dam.commons.util.AssetCache Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL __________________
*
* Copyright 2016 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Adobe Systems Incorporated and its suppliers, if any. The
* intellectual and technical concepts contained herein are proprietary to Adobe Systems Incorporated and its suppliers and are protected by
* trade secret or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden unless prior
* written permission is obtained from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.commons.util;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.api.Rendition;
import org.apache.commons.imaging.common.bytesource.ByteSource;
/**
* Provides convenience methods for handling {@link InputStream}s from and temporary
* files for DAM asset renditions. A proper instance of an {@link AssetCache} can
* be retrieved via {@link DamUtil#getAssetCache()}. Every obtained instance needs
* to be {@link #release()}d after use.
*
* The AssetCache might keep temporary files around for longer and reuse them in
* future {@link AssetCache} instances. This is transparent to the caller.
*
* @deprecated In AEM as a Cloud Service, asset file manipulation should no longer occur directly in the JVM.
* Instead, we recommend using the Asset Workflow Migration Tool to to migrate workflows to
* Processing profiles.
*/
@Deprecated
public interface AssetCache {
/**
* Get an InputStream to the content of the original Asset rendition. The stream
* is automatically closed on release of this cache instance.
*
* @param asset the asset to get the original rendition for
* @param needFile if a file based stream is preferred
* @return stream to original rendition content, closed on release
* @throws IOException if original rendition was not found
*/
InputStream getOriginalStream(Asset asset, boolean needFile) throws IOException;
/**
* Get an InputStream to the content of the Asset rendition with name. The stream
* is automatically closed on release of this cache instance.
*
* @param asset the asset to get the original rendition for
* @param name name of the rendition to get the content for
* @param needFile if a file based stream is preferred
* @return stream to original rendition content, closed on release
* @throws IOException if original rendition was not found
*/
InputStream getRenditionStream(Asset asset, String name, boolean needFile) throws IOException;
/**
* Get an InputStream to the content of the Asset rendition. The stream
* is automatically closed on release of this cache instance.
*
* @param rendition rendition to get the content for
* @param needFile if a file based stream is preferred
* @return stream to original rendition content, closed on release
* @throws IOException if original rendition was not found
*/
InputStream getStream(Rendition rendition, boolean needFile) throws IOException;
/**
* Get an {@link File} with the content of the Asset rendition. The file
* is temporary and will be deleted on cache release (not necessarily the release
* of this cache instance).
*
* @param rendition rendition to get the content for
* @return stream to original rendition content, closed on release
* @throws IOException if original rendition was not found
*/
File getFile(Rendition rendition) throws IOException;
/**
* Get a {@link ByteSource} for the renction's content. The source is
* automatically cleaned up on release of this cache instance.
*
* @param rendition rendition to get the content for
* @param needFile if a file based stream is preferred
* @return stream to original rendition content, closed on release
* @throws IOException if original rendition was not found
*/
ByteSource getByteSource(Rendition rendition, boolean needFile) throws IOException;
/**
* Notify the cache that an asset has been modified/deleted and that cached data is
* no longer valid
* @param asset asset that was modified/deleted.
*/
void invalidateCache(Asset asset);
/**
* Notify the cache that an asset rendition has been modified/deleted and that cached data is
* no longer valid
* @param rendition rendition that was modified/deleted.
*/
void invalidateCache(Rendition rendition);
/**
* Release this cache instance and cleanup all handed out streams and data. Needs
* to be invoked at the end of cache use.
*/
void release();
}