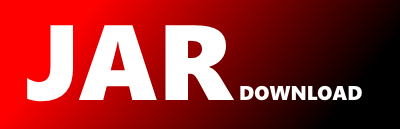
com.day.cq.dam.commons.util.AssetUpdate Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL __________________
*
* Copyright 2016 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Adobe Systems Incorporated and its suppliers, if any. The
* intellectual and technical concepts contained herein are proprietary to Adobe Systems Incorporated and its suppliers and are protected by
* trade secret or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden unless prior
* written permission is obtained from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.commons.util;
import java.io.Serializable;
import java.util.Date;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.api.Rendition;
import com.day.cq.workflow.WorkflowException;
import org.apache.felix.scr.annotations.Reference;
import org.apache.sling.event.jobs.Job;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* An update of (part of) an asset being performed.
*
* Represents an ongoing update of an asset (or an aspect of it, such as its metadata)
* to be used in monitoring workflow processes and allow supervision of execution times
* and successes.
*/
public interface AssetUpdate {
/**
* Get the asset this update is about.
* @return the asset being updated.
*/
Asset getAsset();
/**
* Get the asset, if it is acceptable. Otherwise return null.
* @param check the check to be performed
* @return the checked asset or null
* @throws WorkflowException on general failure
*/
Asset getAsset(Check check) throws WorkflowException;
/**
* Perform and the check, and if successful, run the instance
* @param check the check to be performed on the asset
* @param runner the code to be executed on a successful check
* @throws WorkflowException on general failure
*/
void checkAndRun(Check check, Runner runner) throws WorkflowException;
/**
* Variation of {@link #checkAndRun(Check, Runner)} that can return the identity
* of a started, decoupled sling job.
* @param check the check to be performed on the asset
* @param runner the code to be executed on a successful check
* @return the identifier of the started job or null
* @throws WorkflowException on general failure
*/
Serializable checkAndRunJob(Check check, JobRunner runner) throws WorkflowException;
boolean isIgnored();
/**
* Ignore this update in monitoring.
*/
void ignore();
/**
* Report the upate as finished.
*/
void done();
/**
* Report an error during update.
* @param ex the exception that as caught, or null.
*/
void error(Exception ex);
/**
* Announce that a job is run as part of this update.
* @param job the job that will run
*/
void addJob(Job job);
/**
* Simple check if a null asset (e.g. no longer found) still is acceptable.
*/
interface Check {
boolean isNullAcceptable() throws WorkflowException;
}
/**
* Check if the given asset is acceptable for the asset, e.g. has the correct mime type.
*/
interface AssetCheck extends Check {
boolean isAcceptable(Asset asset) throws WorkflowException;
}
/**
* Run the update on the given asset and update instance. Often used in chain
* with a {@link Check} instance.
*/
interface Runner {
void run(Asset asset, AssetUpdate update) throws WorkflowException, Exception;
}
/**
* Run the update on the given asset and update instance. Often used in chain
* with a {@link Check} instance. THis update may start a job whose id is returned.
*/
interface JobRunner {
Serializable run(Asset asset, AssetUpdate update) throws WorkflowException, Exception;
}
}