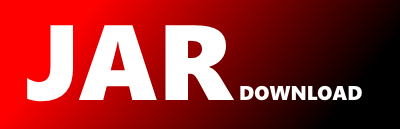
com.day.cq.dam.commons.util.UIHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.commons.util;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.jcr.Node;
import javax.jcr.PathNotFoundException;
import javax.jcr.Property;
import javax.jcr.RepositoryException;
import javax.jcr.ValueFormatException;
import javax.jcr.security.AccessControlManager;
import javax.jcr.security.Privilege;
import org.apache.commons.lang.ArrayUtils;
import org.apache.commons.lang.StringUtils;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.resource.collection.ResourceCollection;
import org.osgi.framework.BundleContext;
import org.osgi.framework.FrameworkUtil;
import org.osgi.framework.ServiceReference;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.adobe.granite.toggle.api.ToggleRouter;
import com.day.cq.commons.LabeledResource;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.api.DamConstants;
import com.day.cq.dam.api.Rendition;
import com.day.cq.i18n.I18n;
import com.day.cq.wcm.api.NameConstants;
import com.day.cq.wcm.api.Page;
import com.day.text.Text;
/**
* This class provides various utility methods pertaining to DAM required
* in presentation/user interface.
*/
public class UIHelper {
private static final Logger log = LoggerFactory.getLogger(UIHelper.class);
private static final String CONTENT_FRAGMENT = "contentFragment";
private static final String ASSET_REPORT_ITEM = "dam/gui/coral/components/admin/reports/viewreport/items/assetreportitem";
/**
* Returns the title of a resource.
* returns dc:title
metadata property is return if resource represents a DAM asset
* returns Page title if the resource represents a cq:Page
* returns title if it is a {@link LabeledResource}
* in other cases, it returns name of the resource or jcr:title property if exists
*
* @param res a resource
* @return title of the resource
*/
public static String getTitle(Resource res) {
String title = null;
try {
Node resNode = res.adaptTo(Node.class);
if (null != resNode) {
Node contentNode = null;
if (resNode.hasNode(JcrConstants.JCR_CONTENT)) {
contentNode = resNode.getNode(JcrConstants.JCR_CONTENT);
}
if (resNode.isNodeType(DamConstants.NT_DAM_ASSET)) {
if ( null != contentNode && contentNode.hasProperty(CONTENT_FRAGMENT) && contentNode.getProperty(CONTENT_FRAGMENT).getBoolean()) {
if (contentNode.hasProperty(JcrConstants.JCR_TITLE)) {
title = contentNode.getProperty(JcrConstants.JCR_TITLE).getString();
} else {
title = resNode.getName();
}
} else {
Node metadata = resNode.getNode(JcrConstants.JCR_CONTENT + "/metadata");
if (metadata != null && metadata.hasProperty(DamConstants.DC_TITLE)) {
Property property = metadata.getProperty(DamConstants.DC_TITLE);
if(property.isMultiple()){
title = property.getValues()[0].getString();
} else {
title = property.getValue().getString();
}
}
}
} else if (resNode.isNodeType(NameConstants.NT_PAGE)) {
Page page = res.adaptTo(Page.class);
title = page.getPageTitle();
if (StringUtils.isBlank(title)) {
if (null != contentNode && contentNode.hasProperty(JcrConstants.JCR_TITLE)) {
title = contentNode.getProperty(JcrConstants.JCR_TITLE).getString();
}
}
}
}
if (null == title) {
LabeledResource lr = res.adaptTo(LabeledResource.class);
if (lr != null) {
title = lr.getTitle();
} else {
if (null != resNode && resNode.hasProperty(JcrConstants.JCR_TITLE)) {
title = resNode.getProperty(JcrConstants.JCR_TITLE).getString();
}
}
}
} catch (Exception ex) {
log.error("error in get title ", ex);
}
if (title != null && !title.equals("")) {
return title;
}
return Text.getName(res.getPath());
}
/**
* Returns best fit rendition, whose width is nearer to given width.
* First it tries to look for thumbnail name starting with "cq5dam.thumbnail.width".
* If such a perfect match is not found, then it tries to look for nearest match by looking at rendition
* width. If a null asset is provided, an IllegalArgumentException will be thrown.
*
* @param asset dam asset
* @param width width of thumbnail in pixels
* @return Rendition best fit rendition
*/
public static Rendition getBestfitRendition(Asset asset, int width) {
if (asset != null) {
List renditions = asset.getRenditions();
return DamUtil.getBestFitRendition(width, renditions);
} else {
throw new IllegalArgumentException("The asset provided is null.");
}
}
/**
* Returns alternate text that is to be used in place of the image when
* displaying the resource's thumbnail
*
* @param resource The resource whose alt text need to be found.
* @return The alternate text that is to be used in place of the image/thumbnail.
*/
public static String getAltText(final Resource resource) {
String altText = "";
if (null != resource) {
ResourceResolver resourceResolver = resource.getResourceResolver();
ValueMap vm = resource.adaptTo(ValueMap.class);
if (DamConstants.NT_DAM_ASSET.equals(vm.get(JcrConstants.JCR_PRIMARYTYPE, String.class))) {
altText = getAssetAltText(vm);
// we put the metadata warning here in order to let UI view could generate such warning
// otherwise, there isn't index for query this problem. and recursively scan the JCR isn't efficient
warningAssetMetadata(resource);
} else if (ASSET_REPORT_ITEM.equals(resource.getResourceType())) {
Resource res = resourceResolver.getResource(resource.getPath());
//if the resource exists i.e. has not been deleted/moved since the report was created
if (null != res) {
altText = getAssetAltText(res.adaptTo(ValueMap.class));
}
} else if (vm.get(JcrConstants.JCR_CONTENT + "/" + CONTENT_FRAGMENT, false)) {
altText = getCfmOrCollectionAltText(vm, JcrConstants.JCR_CONTENT + "/");
} else if (resource.adaptTo(ResourceCollection.class) != null) {
altText = getCfmOrCollectionAltText(vm, "");
}
}
return (StringUtils.isBlank(altText) && null != resource) ? resource.getName() : altText;
}
private static void warningAssetMetadata(Resource resource) {
String prefixMetadataPath = JcrConstants.JCR_CONTENT + "/" + DamConstants.METADATA_FOLDER + "/";
if (resource.getChild(prefixMetadataPath + "xmpMM:History") != null
|| resource.getChild(prefixMetadataPath + "xmpMM:Pantry") != null) {
log.warn("Found asset which metadata still have xmpMM:Pantry and xmpMM:History, asset=\"{}\"", resource.getPath());
}
}
/**
* Returns alternate text that should be shown while displaying the CFM/Collection's thumbnail
*/
private static String getCfmOrCollectionAltText(ValueMap vm, String pathConstant) {
String altText = "";
String description = vm.get(pathConstant +JcrConstants.JCR_DESCRIPTION, String.class);
String title = vm.get(pathConstant + JcrConstants.JCR_TITLE, String.class);
if (!StringUtils.isBlank(description)) {
altText = description;
} else if (!StringUtils.isBlank(title)) {
altText = title;
}
return altText;
}
/**
* Returns alternate text that should be shown while displaying the asset's thumbnail
*/
private static String getAssetAltText(ValueMap vm) {
String altText = "";
String description = vm.get(JcrConstants.JCR_CONTENT + "/" + DamConstants.METADATA_FOLDER + "/" + DamConstants.DC_DESCRIPTION, String.class);
String title = vm.get(JcrConstants.JCR_CONTENT + "/" + DamConstants.METADATA_FOLDER + "/" + DamConstants.DC_TITLE, String.class);
String name = vm.get(JcrConstants.JCR_CONTENT + "/" + DamConstants.PN_NAME , String.class);
if (!StringUtils.isBlank(description)) {
altText = description;
} else if (!StringUtils.isBlank(title)) {
altText = title;
} else if (!StringUtils.isBlank(name)) {
altText = name;
}
return altText;
}
/**
* Returns the cache killer number that can be appended the resource request.
*
* @param node a node
* @return a number which represent last modified timestamp of node/resource
*/
public static long getCacheKiller(Node node) {
long ck = 0;
try {
if (node.isNodeType(DamConstants.NT_DAM_ASSET) || node.isNodeType(JcrConstants.NT_FILE)) {
// ck for asset/rendition is the jcr:content/jcr:lastModified
if (node.hasProperty("jcr:content/jcr:lastModified")) {
ck = node.getProperty("jcr:content/jcr:lastModified").getLong();
}
} else if (node.isNodeType(JcrConstants.NT_FOLDER)) {
// ck for directory is
// jcr:content/folderthumbnail/jcr:lastModified
if (node.hasProperty("jcr:content/folderThumbnail/jcr:content/jcr:lastModified")) {
ck = node.getProperty("jcr:content/folderThumbnail/jcr:content/jcr:lastModified").getLong();
}
} else if (node.hasProperty("jcr:lastModified")) {
ck = node.getProperty("jcr:lastModified").getLong();
}
} catch (Exception e) {
log.error("error creating cache killer", e);
}
// remove milliseconds in order to match the mod date provided by json servlets
return ck / 1000 * 1000;
}
/**
* returns the resource represented by the suffix.
* if the request path is assets.html/content/dam, it will return suffix represnted by /content/dam
* in case of invalid paths, it defaults to /content/dam
*
* @param request sling request
* @return suffix resource
*/
public static Resource getCurrentSuffixResource(SlingHttpServletRequest request) {
String contentPath = request.getRequestPathInfo().getSuffix();
Resource res = request.getResourceResolver().getResource(contentPath);
if (contentPath == null || !contentPath.contains(DamConstants.MOUNTPOINT_ASSETS) || res == null) {
return request.getResourceResolver().getResource(DamConstants.MOUNTPOINT_ASSETS);
}
return res;
}
/**
* Adds units to given size in bytes and create label to be displayed in UI
*
* @param size size in bytes
* @return string label for file/asset size.
*/
public static String getSizeLabel(double size) {
//this method will represent the size in KB, MB, GB and TB appropriately
String units[] = {"B", "KB", "MB", "GB", "TB"};
int i;
for (i = 0; size >= 1024; i++) {
size = size / 1024;
}
return Math.round(size * Math.pow(10, 1)) / Math.pow(10, 1) + " " + units[i];
}
/**
* Adds units to given size in bytes and create label to be displayed in UI
* and Internationalize the result.
*
* @param size size in bytes
* @return string label for file/asset size.
*/
public static String getSizeLabel(double size, SlingHttpServletRequest slingRequest) {
final Locale locale = slingRequest.getResourceBundle(null).getLocale();
final I18n i18n = new I18n(slingRequest);
// this method will represent the size in KB, MB, GB and TB
// appropriately
int i;
for (i = 0; size >= 1024; i++) {
size = size / 1024;
}
final double roundedUpSize = Math.round(size * Math.pow(10, 1)) / Math.pow(10, 1);
String formattedSize;
if (null != locale) {
/* this can happen for an i18n pseudo-build [0]
[0] https://wiki.corp.adobe.com/display/granite/I18n+Checklist#I18nChecklist-PseudoBuild */
final NumberFormat nf = NumberFormat.getInstance(locale);
formattedSize = nf.format(roundedUpSize);
} else {
formattedSize = String.valueOf(roundedUpSize);
}
switch (i) {
case 0:
return i18n.get("{0} B", "Byte", formattedSize);
case 1:
return i18n.get("{0} KB", "KiloByte", formattedSize);
case 2:
return i18n.get("{0} MB", "MegaByte", formattedSize);
case 3:
return i18n.get("{0} GB", "GigaByte", formattedSize);
case 4:
return i18n.get("{0} TB", "TeraByte", formattedSize);
default:
return "";
}
}
/**
* Display resolution with correct i18n number formatting
*
* @param width width
* @param height height
* @param slingRequest request
* @return string label for resolution in i18n'ed format of: width x height
*/
public static String getResolutionLabel(long width, long height, SlingHttpServletRequest slingRequest) {
final Locale locale = slingRequest.getResourceBundle(null).getLocale();
final I18n i18n = new I18n(slingRequest);
String formattedWidth, formattedHeight;
if (null != locale) {
/* this can happen for an i18n pseudo-build [0]
[0] https://wiki.corp.adobe.com/display/granite/I18n+Checklist#I18nChecklist-PseudoBuild */
final NumberFormat nf = NumberFormat.getInstance(locale);
formattedWidth = nf.format(width);
formattedHeight = nf.format(height);
} else {
formattedWidth = String.valueOf(width);
formattedHeight = String.valueOf(height);
}
return i18n.get("{0} x {1}", "width x height, image resolution", formattedWidth, formattedHeight);
}
/**
* returns whether the given resource has the permission to perform the
* privileged action
*
* @param acm AccessControlManager to determine the permissions
* @param res resource
* @param privilege action, wanted to perform on the res
* @return true if user has the permission, otherwise false
* @throws RepositoryException
*/
public static boolean hasPermission(AccessControlManager acm, Resource res, String privilege) throws RepositoryException {
Privilege p = acm.privilegeFromName(privilege);
return acm.hasPrivileges(res.getPath(), new Privilege[]{p});
}
/**
* returns the privileges the session has for the given resource
*
* @param acm AccessControlManager to determine the permissions
* @param res resource
* @return returns the privileges for the resource
* @throws RepositoryException
* @throws PathNotFoundException
*/
public static Privilege[] getAllPermission(AccessControlManager acm, Resource res) throws PathNotFoundException, RepositoryException {
Set allPrivileges = new HashSet();
final Privilege jcrAllPriv = acm.privilegeFromName(Privilege.JCR_ALL);
for (Privilege p : acm.getPrivileges(res.getPath())) {
if (p.isAggregate() && !jcrAllPriv.equals(p)) {
Collections.addAll(allPrivileges, p.getAggregatePrivileges());
}
/* for backwards compatibility of this method,
treat JCR_ALL as a 'single' privilege */
allPrivileges.add(p);
}
return allPrivileges.toArray(new Privilege[0]);
}
/**
* returns whether Interactive Edit(crop/rotate) operations are supported on
* the given mimetype
*
* @param mimetype
* @return
*/
public static boolean isEditSupportedFormat(String mimetype) {
String supportedTypes[] = {"image/jpg", "image/jpeg", "image/png", "image/gif", "image/bmp"};
for (String type : supportedTypes) {
if (type.equalsIgnoreCase(mimetype)) {
return true;
}
}
return false;
}
/**
* Returns best fit rendition, whose size is nearer to given size.
*
* @param asset dam asset
* @param size size in KB
* @return Rendition best fit rendition
*/
public static Rendition getBestFitRendtionBasedOnSize(final Asset asset, final long size) {
return getBestFitRendtionBasedOnSize(asset, size, false);
}
/**
* Returns best fit rendition, whose size is nearer to given size.
*
* @param asset dam asset
* @param size size in KB
* @param preferOriginal return original if size of best fit rendition is greater
* @return Rendition best fit rendition
*/
public static Rendition getBestFitRendtionBasedOnSize(final Asset asset, final long size,
boolean preferOriginal) {
try {
long sizeinBytes = size * 1024;
List renditions = asset.getRenditions();
UIHelper.SizeBasedRenditionComparator comp = new UIHelper.SizeBasedRenditionComparator();
Collections.sort(renditions, comp);
Iterator itr = renditions.iterator();
Rendition bestFit = null;
while (itr.hasNext()) {
Rendition rend = itr.next();
if (canRenderOnWeb(rend.getMimeType())) {
if (rend.getSize() <= sizeinBytes) {
bestFit = rend;
}
}
}
// if all renditions are larger then given size, find rendition
// closest to size;
if (bestFit == null) {
itr = renditions.iterator();
while (itr.hasNext()) {
Rendition rend = itr.next();
if (canRenderOnWeb(rend.getMimeType())) {
bestFit = rend;
break;
}
}
} else if (preferOriginal) {
// prefer original when size of original is smaller than bestFit
// rendition & can be rendered on WEB
// Not applicable when all renditions are greater than size
// given
if (canRenderOnWeb(asset.getOriginal().getMimeType())
&& bestFit.getSize() > asset.getOriginal().getSize()) {
return asset.getOriginal();
}
}
return bestFit;
} catch (Exception e) {
log.error("Error occured while getting best fit rendition ", e);
return null;
}
}
/**
* Returns true if the given mime type can be rendered in HTML by browser.
*
* @param mimeType
* @return
*/
public static boolean canRenderOnWeb(final String mimeType) {
if(mimeType == null)
return false;
String lowerCaseMimeType = mimeType.toLowerCase();
return (lowerCaseMimeType.contains("jpeg")
|| lowerCaseMimeType.contains("jpg")
|| lowerCaseMimeType.contains("gif")
|| lowerCaseMimeType.contains("png")
|| lowerCaseMimeType.contains("bmp")
|| lowerCaseMimeType.contains("webp"));
}
/**
* Returns the width of rendition if it is the original rendition,
* and has dimension metadata, or if it follows the naming convention
* cq5dam.[thumbnail|web].width.height.ext,else 0
*
* @param r Rendition whose width is required
* @return width if it follows the naming convention, else 0
*/
public static int getWidth(final Rendition r) {
return getDimension(r, DamConstants.TIFF_IMAGEWIDTH);
}
/**
* Returns the height of rendition if it is the original rendition,
* and has dimension metadata, or if it follows the naming convention
* cq5dam.[thumbnail|web].width.height.ext,else 0
*
* @param r Rendition whose width is required
* @return width if it follows the naming convention, else 0
*/
public static int getHeight(final Rendition r) {
return getDimension(r, DamConstants.TIFF_IMAGELENGTH);
}
// assuming convention cq5dam.[thumbnail|web].width.height.ext Note: This would be better with named regex starting in java 1.7
private static final Pattern renditionPattern = Pattern.compile("cq5dam\\.(.*)?\\.(\\d+)\\.(\\d+)\\.(.*)");
private static final int PATTERN_WIDTH_INDEX = 2;
private static final int PATTERN_HEIGHT_INDEX = 3;
//package scope for unit tests.
private static int getDimension(final Rendition r, final String dimensionProperty) {
if(r == null) {
log.debug("Null rendition at", new Exception("Null rendition"));
return 0;
}
if(dimensionProperty == null || (!dimensionProperty.equals(DamConstants.TIFF_IMAGELENGTH) && !dimensionProperty.equals(DamConstants.TIFF_IMAGEWIDTH))) {
log.warn("Incorrect dimension property for {}", r.getPath(), new Exception("Invalid property name " + dimensionProperty)); //This indicates a programatic error in this class.
return 0;
}
String name = r.getName();
if(name == null) {
log.warn("Null name returned at {}", r.getPath());
return 0;
}
// assuming convention cq5dam.[thumbnail|web].width.height.ext
try {
if(name.equals(DamConstants.ORIGINAL_FILE)) {
Asset asset = r.adaptTo(Asset.class);
if(asset == null) {
log.debug("Rendition at {} is not adaptable to an asset.", r.getPath());
return 0;
}
String val = asset.getMetadataValue(dimensionProperty);
if(val == null || val.length() == 0) {
//This can happen for vector types (PDF, SVG, AI, EPS, etc..) and for assets that have not had their async workflows processed.
log.debug("Unable to find metadata property {} for {}", dimensionProperty, asset.getPath());
return 0;
}
try {
return Integer.parseInt(val);
}
catch(NumberFormatException nfe) {
log.warn("Metadata property {} was {} and not a number at {}", new Object[]{dimensionProperty, val, asset.getPath()});
return 0;
}
}
Matcher matcher = renditionPattern.matcher(name);
if(matcher.matches()) {
final int matcherIndex;
if(DamConstants.TIFF_IMAGELENGTH.equals(dimensionProperty)) {
matcherIndex = PATTERN_HEIGHT_INDEX;
}
else {
matcherIndex = PATTERN_WIDTH_INDEX;
}
int renditionHeight = Integer.parseInt(matcher.group(matcherIndex));
return renditionHeight;
}
else {
log.debug("Unknown naming format for name {} at {}", name, r.getPath());
return 0;
}
} catch (Exception e) {
log.warn("Unexpected exception finding dimension for asset at {} " + r.getPath(), e);
return 0;
}
}
/**
* Please use lookupMimeType(String, Resource, boolean)
*/
@Deprecated
public static String lookupMimeType(String mimeType, Node node, boolean uppercase) {
throw new UnsupportedOperationException("This API has been deprecated. Use lookupMimeType(String, Resource, uppercase) instead.");
}
/**
* does a lookup for the given mimetype in the given resource.
*
* @param mimeType
* @param resource
* @param uppercase
* @return title/description of the matched resource
*/
public static String lookupMimeType(String mimeType, Resource resource,
boolean uppercase) {
try {
if (resource == null || mimeType == null) {
return null;
}
for (Iterator it = resource.listChildren(); it.hasNext();) {
Resource child = it.next();
ValueMap childVM = child.adaptTo(ValueMap.class);
String mimetypes = childVM.get("mimetypes", String.class);
String mimetypesList[] = mimetypes.split(",");
if (ArrayUtils.contains(mimetypesList, mimeType.toUpperCase())) {
if (uppercase) {
return childVM.get(JcrConstants.JCR_DESCRIPTION,
String.class);
} else {
return childVM
.get(JcrConstants.JCR_TITLE, String.class);
}
}
}
} catch (Exception e) {
log.error(e.getMessage());
}
return null;
}
/**
* Returns true, if an asset is checked out by drive. False otherwise.
*
* @param asset Asset whose status needs to be determined
* @return True if asset is checked out by drive, false otherwise
*/
public static boolean isCheckedOutByDrive(final Asset asset) {
try {
Node assetNode = asset.adaptTo(Node.class);
Node jcrContent = assetNode.getNode("jcr:content");
if (jcrContent != null) {
if (jcrContent.hasProperty("cq:drivelock")) {
return true;
}
}
} catch (PathNotFoundException e) {
log.warn("Asset does not exists ", e);
} catch (RepositoryException e) {
log.warn("Respoitory execption", e);
}
return false;
}
public static String getCheckedOutby(final Asset asset) {
if (isCheckedOutByDrive(asset)) {
try {
Node assetNode = asset.adaptTo(Node.class);
Node jcrContent = assetNode.getNode("jcr:content");
return jcrContent.getProperty("cq:drivelock").getString();
} catch (PathNotFoundException e) {
log.warn("Asset does not exists ", e);
} catch (ValueFormatException e) {
log.warn("Value format exception ", e);
} catch (RepositoryException e) {
log.warn("Respoitory execption", e);
}
}
return "";
}
private static class SizeBasedRenditionComparator implements Comparator {
public int compare(Rendition r1, Rendition r2) {
if (r1.getSize() < r2.getSize()) {
return -1;
} else if (r1.getSize() == r2.getSize()) {
return 0;
} else {
return 1;
}
}
}
/**
* Structs for specifying action rel filters
* Want to create bitmaps for fast comparison. But will be for a later todo.
*/
public static class ActionRelsResourceProperties {
public boolean isAssetExpired;
public boolean isSubAssetExpired;
public boolean isContentFragment;
public boolean isArchive;
public boolean isSnippetTemplate;
public boolean isDownloadable;
public boolean isStockAsset;
public boolean isStockAssetLicensed;
public boolean isStockAccessible;
public boolean canFindSimilar;
ActionRelsResourceProperties() {
isAssetExpired = false;
isSubAssetExpired = false;
isContentFragment = false;
isArchive = false;
isSnippetTemplate = false;
isDownloadable = false;
isStockAsset = false;
isStockAssetLicensed = false;
isStockAccessible = false;
canFindSimilar = false;
}
public static ActionRelsResourceProperties create(
boolean isAssetExpired,
boolean isSubAssetExpired,
boolean isContentFragment,
boolean isArchive,
boolean isSnippetTemplate,
boolean isDownloadable,
boolean isStockAsset,
boolean isStockAssetLicensed,
boolean isStockAccessible,
boolean canFindSimilar
) {
ActionRelsResourceProperties obj = new ActionRelsResourceProperties();
obj.isAssetExpired = isAssetExpired;
obj.isSubAssetExpired = isSubAssetExpired;
obj.isContentFragment = isContentFragment;
obj.isArchive = isArchive;
obj.isSnippetTemplate = isSnippetTemplate;
obj.isDownloadable = isDownloadable;
obj.isStockAsset = isStockAsset;
obj.isStockAssetLicensed = isStockAssetLicensed;
obj.isStockAccessible = isStockAccessible;
obj.canFindSimilar = canFindSimilar;
return obj;
}
}
public static class ActionRelsUserProperties {
boolean hasJcrRead;
boolean hasJcrWrite;
boolean hasAddChild;
boolean canEdit;
boolean canAnnotate;
boolean isAdmin;
ActionRelsUserProperties() {
hasJcrRead = false;
hasJcrWrite = false;
hasAddChild = false;
canEdit = false;
canAnnotate = false;
isAdmin = false;
}
public static ActionRelsUserProperties create(
boolean hasJcrRead,
boolean hasJcrWrite,
boolean hasAddChild,
boolean canEdit,
boolean canAnnotate,
boolean isAdmin
) {
ActionRelsUserProperties obj = new ActionRelsUserProperties();
obj.hasJcrRead = hasJcrRead;
obj.hasJcrWrite = hasJcrWrite;
obj.hasAddChild = hasAddChild;
obj.canEdit = canEdit;
obj.canAnnotate = canAnnotate;
obj.isAdmin = isAdmin;
return obj;
}
}
public static class ActionRelsRequestProperties {
boolean isOmniSearchRequest;
boolean isLiveCopy;
ActionRelsRequestProperties() {
isOmniSearchRequest = false;
isLiveCopy = false;
}
public static ActionRelsRequestProperties create(
boolean isOmniSearchRequest,
boolean isLiveCopy) {
ActionRelsRequestProperties req = new ActionRelsRequestProperties();
req.isOmniSearchRequest = isOmniSearchRequest;
req.isLiveCopy = isLiveCopy;
return req;
}
}
/**
* Returns list of actions rels string for assets
* @param resource - properties of the resource in question
* @param user - properties of the current principal
* @param request - properties of the request itself
*/
public static List getAssetActionRels(
final ActionRelsResourceProperties resource,
final ActionRelsUserProperties user,
final ActionRelsRequestProperties request) {
List actionRels = null;
if ((resource != null) && (user != null) && (request != null)) {
actionRels = getAssetActionRels(
user.hasJcrRead,
user.hasJcrWrite,
user.hasAddChild,
user.canEdit,
user.canAnnotate,
user.isAdmin,
resource.isAssetExpired,
resource.isSubAssetExpired,
resource.isContentFragment,
resource.isArchive,
resource.isSnippetTemplate,
resource.isDownloadable,
request.isOmniSearchRequest,
resource.isStockAsset,
resource.isStockAssetLicensed,
resource.isStockAccessible,
request.isLiveCopy
);
if (actionRels == null) {
actionRels = new ArrayList();
}
if ((actionRels != null) && resource.canFindSimilar) {
actionRels.add("cq-damadmin-admin-actions-findsimilar-activator");
}
}
return actionRels;
}
/**
* Returns list of actions rels string for assets
*
* @param hasJcrRead hasJcrRead flag
* @param hasJcrWrite hasJcrWrite flag
* @param hasAddChild hasAddChild flag
* @param canEdit canEdit flag
* @param canAnnotate canAnnotate flag
* @param isAdmin isAdmin flag
* @param isAssetExpired isAssetExpired flag
* @param isSubAssetExpired isSubAssetExpired flag
* @param isContentFragment isContentFragment flag
* @param isArchive isArchive flag
* @param isSnippetTemplate isSnippetTemplate flag
* @param isDownloadable isDownloadable flag
* @param isOmniSearchRequest isOmniSearchRequest flag
* @param isStockAsset isStockAsset flag
* @param isStockAssetLicensed isStockAssetLicensed flag
* @param isStockAccessible isStockAccessible flag
* @param isLiveCopy isLiveCopy flag
* @return List of actions rels strings
*/
public static List getAssetActionRels(boolean hasJcrRead, boolean hasJcrWrite, boolean hasAddChild,
boolean canEdit, boolean canAnnotate, boolean isAdmin, boolean isAssetExpired, boolean isSubAssetExpired,
boolean isContentFragment, boolean isArchive, boolean isSnippetTemplate, boolean isDownloadable,
boolean isOmniSearchRequest, boolean isStockAsset, boolean isStockAssetLicensed,
boolean isStockAccessible, boolean isLiveCopy) {
BundleContext bundleContext = FrameworkUtil.getBundle(UIHelper.class).getBundleContext();
ServiceReference toggleRouterRef = bundleContext.getServiceReference(ToggleRouter.class);
List actionRels = new ArrayList();
boolean isToggleEnabled = false;
if (toggleRouterRef != null) {
ToggleRouter toggleRouter = bundleContext.getService(toggleRouterRef);
if(toggleRouter != null && toggleRouter.isEnabled("FT_SITES-116")) {
isToggleEnabled = true;
}
}
actionRels.add("cq-damadmin-admin-actions-createworkflow");
if (isContentFragment && isOmniSearchRequest) {
actionRels.add("aem-assets-admin-actions-edit-fragment-activator");
if(isToggleEnabled) {
actionRels.add("cq-damadmin-admin-actions-createlaunch");
}
}
if (hasJcrRead) {
if (!isContentFragment) {
actionRels.add("foundation-damadmin-properties-activator");
} else {
actionRels.add("foundation-damadmin-fragmentprops-activator");
}
actionRels.add("cq-damadmin-admin-actions-add-to-collection-activator");
actionRels.add("cq-damadmin-admin-actions-desktop-activator");
actionRels.add("cq-damadmin-admin-actions-opendesktop");
actionRels.add("cq-damadmin-admin-actions-editdesktop");
actionRels.add("cq-damadmin-admin-actions-revealdesktop");
actionRels.add("aem-assets-admin-actions-moderatetags-activator");
}
if (hasJcrWrite) {
actionRels.add("cq-damadmin-admin-actions-open-activator");
actionRels.add("aem-assets-admin-actions-shoppableedit-activator");
actionRels.add("cq-damadmin-admin-actions-createversion");
if (!isContentFragment) {
actionRels.add("cq-damadmin-admin-actions-createlivecopy");
if (!isLiveCopy) {
actionRels.add("cq-siteadmin-admin-actions-relate-activator");
actionRels.add("cq-siteadmin-admin-actions-unrelate-activator");
actionRels.add("dam-asset-reprocessassets-action-activator");
}
} else {
if(isToggleEnabled) {
actionRels.add("cq-damadmin-admin-actions-createlaunch");
}
}
}
if (hasAddChild) {
actionRels.add("cq-damadmin-admin-actions-open-activator");
actionRels.add("icon-note");
}
// Content Fragment Editor can be viewed in "read-only mode" if user cannot edit.
if ((canEdit || isContentFragment) && (isAdmin || !isAssetExpired)) {
if (!isContentFragment || !isOmniSearchRequest) {
actionRels.add("aem-assets-admin-actions-edit-activator");
}
actionRels.add("dam-assetedit-action-select");
}
if (canAnnotate && !isLiveCopy) {
actionRels.add("aem-assets-admin-actions-annotate-activator");
actionRels.add("cq-project-admin-actions-annotate-activator");
}
if ((isAdmin || (!isAssetExpired && !isSubAssetExpired)) && isDownloadable) {
if (!isContentFragment) {
actionRels.add("cq-damadmin-admin-actions-download-activator");
} else {
actionRels.add("cq-damadmin-admin-actions-downloadcf-activator");
}
}
if (isArchive) {
actionRels.add("cq-damadmin-admin-actions-extract-activator");
}
if (hasJcrWrite && isSnippetTemplate) {
actionRels.add("cq-damadmin-admin-actions-createsnippet-activator");
}
if (isStockAccessible && isStockAsset) {
actionRels.add("cq-damadmin-admin-actions-stock-viewsimilar-activator");
actionRels.add("cq-damadmin-admin-actions-stock-viewexternal-activator");
if (!isStockAssetLicensed) {
actionRels.add("cq-damadmin-admin-actions-stock-license-activator");
} else {
actionRels.add("cq-damadmin-admin-actions-stock-licenseagain-activator");
}
}
if(isOmniSearchRequest){
actionRels.add("cq-damadmin-admin-actions-goto-activator");
}
return actionRels;
}
/**
* Returns list of actions rels string for directories
*
* @deprecated Replaced by {@link UIHelper#getDirectoryActionRels(boolean, boolean, boolean, boolean, boolean,
* boolean, boolean, boolean, boolean, boolean, boolean, boolean, boolean)}
*
* @param hasJcrRead hasJcrRead flag
* @param hasModifyAccessControl hasModifyAccessControl flag
* @param hasJcrWrite hasJcrWrite flag
* @param hasReplicate hasReplicate flag
* @param isMACShared isMACShared flag
* @param isCCShared isCCShared flag
* @param isRootMACShared isRootMACShared flag
* @param isMPShared isMPShared flag
* @param isRootMPShared isRootMPShared flag
* @param isLiveCopy isLiveCopy flag
* @return List of actions rels strings
*/
@Deprecated
public static List getDirectoryActionRels(boolean hasJcrRead, boolean hasModifyAccessControl, boolean hasJcrWrite,
boolean hasReplicate, boolean isMACShared, boolean isCCShared,
boolean isRootMACShared, boolean isMPShared, boolean isRootMPShared, boolean isLiveCopy) {
List actionRels = new ArrayList();
actionRels.add("cq-damadmin-admin-actions-createworkflow");
if (hasJcrRead) {
actionRels.add("icon-share");
actionRels.add("cq-damadmin-admin-actions-download-activator");
actionRels.add("cq-damadmin-admin-actions-add-to-collection-activator");
actionRels.add("cq-damadmin-admin-actions-revealdesktop");
actionRels.add("aem-assets-admin-actions-moderatetags-activator");
actionRels.add("cq-damadmin-admin-actions-foldershare");
}
if (hasModifyAccessControl) {
actionRels.add("cq-damadmin-admin-actions-adhocassetshare-activator");
actionRels.add("cq-damadmin-admin-actions-publicLinkShare-activator");
}
if (hasJcrWrite) {
actionRels.add("cq-damadmin-admin-actions-fileupload-at-activator");
actionRels.add("cq-damadmin-admin-actions-createfolder-at-activator");
actionRels.add("cq-damadmin-admin-actions-imageset-at-activator");
actionRels.add("cq-damadmin-admin-actions-spinset-at-activator");
actionRels.add("cq-damadmin-admin-actions-mixedmedia-at-activator");
actionRels.add("cq-damadmin-admin-actions-createcarousel-at-activator");
actionRels.add("cq-damadmin-admin-actions-createfragment-at-activator");
actionRels.add("cq-damadmin-admin-actions-createlivecopy");
actionRels.add("dam-asset-exportmetadata-action-activator");
actionRels.add("dam-asset-reprocessassets-action-activator");
if (!isLiveCopy) {
actionRels.add("dam-asset-createtask-action-activator");
}
}
if (hasJcrWrite && hasReplicate) {
actionRels.add("cq-damadmin-admin-actions-macshare-activator");
actionRels.add("cq-damadmin-admin-actions-mpshare-activator");
}
if (isMACShared || isCCShared || isRootMACShared || isMPShared || isRootMPShared) {
actionRels.remove("cq-damadmin-admin-actions-move-activator");
actionRels.remove("cq-damadmin-admin-actions-delete-activator");
}
return actionRels;
}
/**
* Returns list of actions rels string for directories
*
* @param hasJcrRead hasJcrRead flag
* @param hasModifyAccessControl hasModifyAccessControl flag
* @param hasJcrWrite hasJcrWrite flag
* @param hasReplicate hasReplicate flag
* @param isMACShared isMACShared flag
* @param isCCShared isCCShared flag
* @param isRootMACShared isRootMACShared flag
* @param isMPShared isMPShared flag
* @param isRootMPShared isRootMPShared flag
* @param isLiveCopy isLiveCopy flag
* @param hasAddChild Indicates if jcr:addChildNodes permission is granted
* @param hasRemoveNode Indicates if jcr:removeNode permission is granted
* @param hasModifyProperties Indicates if jcr:modifyProperties permission is granted
* @return List of actions rels strings
*/
public static List getDirectoryActionRels(boolean hasJcrRead, boolean hasModifyAccessControl, boolean hasJcrWrite,
boolean hasReplicate, boolean isMACShared, boolean isCCShared,
boolean isRootMACShared, boolean isMPShared, boolean isRootMPShared,
boolean isLiveCopy, boolean hasAddChild, boolean hasRemoveNode,
boolean hasModifyProperties) {
List actionRels = new ArrayList();
actionRels.add("cq-damadmin-admin-actions-createworkflow");
if (hasJcrRead) {
actionRels.add("icon-share");
actionRels.add("cq-damadmin-admin-actions-download-activator");
actionRels.add("cq-damadmin-admin-actions-add-to-collection-activator");
actionRels.add("cq-damadmin-admin-actions-revealdesktop");
actionRels.add("aem-assets-admin-actions-moderatetags-activator");
actionRels.add("cq-damadmin-admin-actions-foldershare");
}
if (hasModifyAccessControl) {
actionRels.add("cq-damadmin-admin-actions-adhocassetshare-activator");
actionRels.add("cq-damadmin-admin-actions-publicLinkShare-activator");
}
if (hasAddChild && hasModifyProperties) {
actionRels.add("cq-damadmin-admin-actions-fileupload-at-activator");
actionRels.add("cq-damadmin-admin-actions-createfolder-at-activator");
actionRels.add("cq-damadmin-admin-actions-createfragment-at-activator");
actionRels.add("cq-damadmin-admin-actions-createlivecopy");
actionRels.add("cq-damadmin-admin-actions-imageset-at-activator");
actionRels.add("cq-damadmin-admin-actions-spinset-at-activator");
actionRels.add("cq-damadmin-admin-actions-mixedmedia-at-activator");
actionRels.add("cq-damadmin-admin-actions-createcarousel-at-activator");
actionRels.add("dam-asset-exportmetadata-action-activator");
actionRels.add("dam-asset-reprocessassets-action-activator");
if (!isLiveCopy) {
actionRels.add("dam-asset-createtask-action-activator");
}
}
if (hasJcrWrite && hasReplicate) {
actionRels.add("cq-damadmin-admin-actions-macshare-activator");
actionRels.add("cq-damadmin-admin-actions-mpshare-activator");
}
if (isMACShared || isCCShared || isRootMACShared || isMPShared || isRootMPShared) {
actionRels.remove("cq-damadmin-admin-actions-move-activator");
actionRels.remove("cq-damadmin-admin-actions-delete-activator");
}
return actionRels;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy