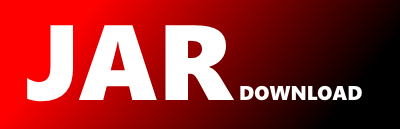
com.day.cq.dam.handler.ffmpeg.LocatorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.dam.handler.ffmpeg;
import java.io.File;
import java.util.ArrayList;
import java.util.Dictionary;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.SystemUtils;
import org.apache.felix.scr.annotations.Component;
import org.apache.felix.scr.annotations.Properties;
import org.apache.felix.scr.annotations.Property;
import org.apache.felix.scr.annotations.Service;
import org.apache.sling.commons.osgi.OsgiUtil;
import org.osgi.service.component.ComponentContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Finds an executable on a configurable search path.
*
*/
@Component(metatype = true, label = "Day CQ Executable Locator", description = "Locates command line executables")
@Service
@Properties({ @Property(name="service.description", value="CQ Executable Locator") })
public class LocatorImpl implements ExecutableLocator {
private static final Logger log = LoggerFactory.getLogger(LocatorImpl.class);
@Property(value={"/opt/local/bin", "/usr/local/bin", "PATH"})
public static final String PROP_SEARCH_PATH = "executable.searchpath";
private static final String PATH_ENV = "PATH";
private static final String[] FALLBACK_SEARCH_PATH = { PATH_ENV };
private List searchPath;
private File last;
/**
* Default constructor for SCR.
*/
public LocatorImpl() {
}
/**
* Constructor for non-OSGi component usage.
* @param searchPath list of search paths
*/
public LocatorImpl(String[] searchPath) {
setSearchPath(searchPath);
}
protected void activate(final ComponentContext componentContext) {
final Dictionary, ?> properties = componentContext.getProperties();
String[] sp = OsgiUtil.toStringArray(properties.get(PROP_SEARCH_PATH));
if (sp == null || sp.length == 0) {
setSearchPath(FALLBACK_SEARCH_PATH);
} else {
setSearchPath(sp);
}
}
protected void setSearchPath(String[] sp) {
searchPath = new ArrayList();
for (int i = 0; i < sp.length; i++) {
if (PATH_ENV.equals(sp[i])) {
String[] paths = getPathEnv();
for (int j = 0; j < paths.length; j++) {
searchPath.add(paths[j]);
}
} else {
searchPath.add(sp[i]);
}
}
log.info("Search path: {}", StringUtils.join(searchPath.toArray(), ", "));
}
public synchronized String getPath(String cmd) {
if (SystemUtils.IS_OS_WINDOWS && cmd != null && !cmd.endsWith(".exe")) {
cmd = cmd + ".exe";
}
if (last != null && last.getName().equals(cmd) && last.exists()) {
log.debug("Found executable '{}' (cached): {}", cmd, last.getAbsolutePath());
return last.getAbsolutePath();
}
if (searchPath != null) {
for (String path : searchPath) {
File file = new File(path, cmd);
if (file.exists()) {
last = file;
log.debug("Found executable '{}': {}", cmd, file.getAbsolutePath());
return file.getAbsolutePath();
}
}
}
log.warn("Could not find executable '{}'!", cmd);
// not found
return null;
}
public static String[] getPathEnv() {
String path = System.getenv("PATH");
if (path == null) {
path = System.getenv("Path");
}
if (path == null) {
return new String[0];
}
return StringUtils.split(path, File.pathSeparatorChar);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy