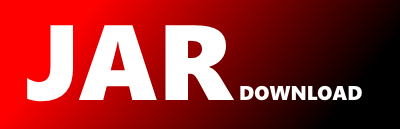
com.day.cq.dam.scene7.api.Scene7DAMService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.scene7.api;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.dam.api.Asset;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.io.IOException;
/**
* The {@code Scene7DAMAssetService} is responsible for obtaining S7 related information about already imported DAM
* assets.
*/
@ProviderType
public interface Scene7DAMService {
/**
* For a DAM imported Scene7 asset, this method returns the file reference of the asset from Scene7.
* This is actually the URL used to retrieve the Scene7 asset from the Scene7 public servers.
* In case a DAM asset cannot be identified as an imported Scene7 asset, this method will return null.
* @param asset the DAM asset
* @return the URL to the Scene7 asset; null if the asset is not imported from Scene7
*/
String getS7FileReference(Asset asset);
/**
* For a DAM imported Scene7 asset, this method returns the file reference of the asset from Scene7.
* This is actually the URL used to retrieve the Scene7 asset from the Scene7 public servers.
* In case a DAM asset cannot be identified as an imported Scene7 asset, this method will return null.
* @param asset the DAM asset
* @param configPath Optional path to indicate what cloud configuration to use. If null, an attempt will be made to
* extract the cloud config from the asset.
* @return the URL to the Scene7 asset; null if the asset is not imported from Scene7
*/
String getS7FileReference(Asset asset, String configPath);
/**
* For a DAM imported Scene7 asset, this method returns the file reference
* of the asset from Scene7. This is actually the URL used to retrieve the
* Scene7 asset from the Scene7 public or preview servers. In case a DAM
* asset cannot be identified as an imported Scene7 asset, this method will
* return null.
*
* @param asset
* the DAM asset
* @param configPath
* Optional path to indicate what cloud configuration to use. If
* null, an attempt will be made to extract the cloud config from
* the asset.
* @param isPreview
* the Scene7 public or preview server URL
* @return the URL to the Scene7 asset; null if the asset is not imported
* from Scene7
*/
String getS7FileReference(Asset asset, String configPath, boolean isPreview);
/**
* Update an embedded Scene7 asset URL reference in WCM component
*
* @param resource
* Resource object of the WCM component
* @param fileReference
* Scene7 image or video asset source URL
* @return whether the update operation is successful
*/
boolean setS7FileReference(Resource resource, String fileReference);
/**
* Update an embedded Dynamic Media Scene7 asset URL reference in WCM
* component
*
* @param resource
* Resource object of the WCM component
* @param fileReference
* Scene7 image or video asset source URL
* @return whether the update operation is successful
*/
boolean setS7DMFileReference(Resource resource, String fileReference);
/**
* Sets properties on the {@code Resource} JCR node to store its relevant
* Scene7 properties. This method is compatible with Scene7 assets (e.g.
* images, videos) and company settings (e.g. image presets, viewer
* presets). The session must be manually saved.
*
* @param resource
* Resource object on which to add Scene7 metadata
* @param scene7Handle
* Scene7 asset handle or asset ID
* @param s7Config
* the Scene7 configuration
* @param status
* the status of the sync operation (e.g. the status of an upload
* job)
* @param deleteOnFail
* whether to delete Scene7 remote assets/settings when the
* properties save action fails,
* @throws Exception .
* @deprecated Use {@link Scene7FileMetadataService#setResourceMetadataOnSync} instead
*/
@Deprecated
void setResourceMetadataOnSync(Resource resource, String scene7Handle,
S7Config s7Config, String status, Boolean deleteOnFail)
throws Exception;
/**
* Update the static renditions using DM Preview server url using thumbnail asset path or the Video itself.
* If no thumbnail asset is provided, video asset is/image url will be used to update static thumbnails.
* @param resource Video Resource which needs to be updated
* @param thumbAssetPath Thumbnail asset to be used for updating thumbnail.
*@throws Exception when unable to generate static renditions.
*
*/
void updateDMVideoStaticRendition(@Nonnull Resource resource, @Nullable String thumbAssetPath) throws Exception;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy