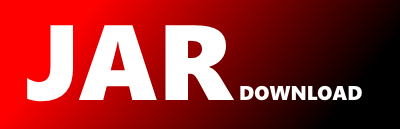
com.day.cq.dam.scene7.api.Scene7FileMetadataService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.scene7.api;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.scene7.api.model.Scene7Asset;
/**
* The {@code Scene7FileMetadataService} is responsible for the management of Scene7 specific information for CQ DAM assets.
*/
@ProviderType
public interface Scene7FileMetadataService {
/**
* Sets properties on the {@code metadata} node of a CQ DAM asset. The session must be manually saved.
*
* @param asset
* the CQ DAM asset
* @param propertyName
* the property's name
* @param propertyValue
* the property's value
* @throws Exception
* if the property cannot be added / modified
*/
public abstract void setAssetMetadataProperty(Asset asset, String propertyName, Object propertyValue) throws Exception;
/**
* Sets multiple properties at the same time on a CQ DAM asset to store its relevant Scene7 properties. The session must be manually
* saved.
*
* @param asset
* the CQ DAM asset on which to add metadata information
* @param scene7Asset
* the {@code Scene7Asset} from which to extract the metadata information
* @param s7Config
* the Scene7 configuration
* @param status
* the status of the sync operation (e.g. the status of an upload job)
* @throws Exception
* if the property cannot be added / modified
*/
public abstract void setAssetMetadataOnSync(Asset asset, Scene7Asset scene7Asset, S7Config s7Config, String status) throws Exception;
/**
* Removes a metadata property from a CQ DAM asset. The session must be manually saved.
*
* @param asset
* the CQ DAM asset
* @param propertyName
* the property's name
* @throws Exception
* if the property cannot be removed
*/
public abstract void removeAssetMetadataProperty(Asset asset, String propertyName) throws Exception;
/**
* Sets properties on the {@code jcr:content} node of a CQ DAM asset. The
* session must be manually saved.
*
* @param asset
* the CQ DAM asset
* @param propertyName
* the property's name
* @param propertyValue
* the property's value
* @throws Exception
* if the property cannot be added / modified
*/
public abstract void setAssetJcrContentProperty(Asset asset,
String propertyName, Object propertyValue) throws Exception;
/**
* Sets properties on the {@code Resource} JCR node to store its relevant
* Scene7 properties. This method is compatible with Scene7 assets (e.g.
* images, videos) and company settings (e.g. image presets, viewer
* presets). The session must be manually saved.
*
* @param resource
* Resource object on which to add Scene7 metadata
* @param scene7Handle
* Scene7 asset handle or asset ID
* @param s7Config
* the Scene7 configuration
* @param status
* the status of the sync operation (e.g. the status of an upload
* job)
* @param deleteOnFail
* whether to delete Scene7 remote assets/settings when the
* properties save action fails,
* @throws Exception
*/
void setResourceMetadataOnSync(Resource resource, String scene7Handle,
S7Config s7Config, String status, Boolean deleteOnFail)
throws Exception;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy