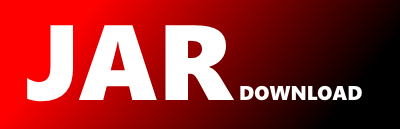
com.day.cq.dam.scene7.api.Scene7Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.dam.scene7.api;
import java.io.IOException;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.day.cq.dam.scene7.api.constants.ManifestType;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.jetbrains.annotations.Nullable;
import org.joda.time.Instant;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.dam.api.Asset;
import com.day.cq.dam.scene7.api.model.MetadataCondition;
import com.day.cq.dam.scene7.api.model.Scene7Asset;
import com.day.cq.dam.scene7.api.model.Scene7AssetSet;
import com.day.cq.dam.scene7.api.model.Scene7BatchMetadataResult;
import com.day.cq.dam.scene7.api.model.Scene7CompanyMembership;
import com.day.cq.dam.scene7.api.model.Scene7ConfigSetting;
import com.day.cq.dam.scene7.api.model.Scene7Folder;
import com.day.cq.dam.scene7.api.model.Scene7ImageFieldUpdate;
import com.day.cq.dam.scene7.api.model.Scene7ImageFormat;
import com.day.cq.dam.scene7.api.model.Scene7ImageMapDefinition;
import com.day.cq.dam.scene7.api.model.Scene7MetadataUpdate;
import com.day.cq.dam.scene7.api.model.Scene7NormalizedCropRect;
import com.day.cq.dam.scene7.api.model.Scene7PropertySet;
import com.day.cq.dam.scene7.api.model.Scene7User;
import com.day.cq.dam.scene7.api.model.Scene7ViewerConfig;
import com.day.cq.dam.scene7.api.model.UploadJobDetail;
import com.scene7.ipsapi.CdnCacheInvalidationReturn;
import com.scene7.ipsapi.ImageSetMemberUpdateArray;
import com.scene7.ipsapi.ImageSetMemberArray;
import com.scene7.ipsapi.UrlArray;
import com.scene7.ipsapi.Company;
/**
* The Scene7Service
provides methods for interacting with the official Scene7 API.
*
*/
@ProviderType
@NotNullApi
public interface Scene7Service {
// Assoc types
/**
* Scene7 specification used in getAssociatedAssets - Array of set and template assets containing the specified asset.
*/
public static final int CONTAINER = 1;
/**
* Scene7 specification used in getAssociatedAssets - Array of assets contained by the specified set or template asset.
*/
public static final int MEMBER = 2;
/**
* Scene7 specification used in getAssociatedAssets - Array of assets that own the specified asset.
*/
public static final int OWNER = 3;
/**
* Scene7 specification used in getAssociatedAssets - Array of assets that are derivatives of the specified asset.
*/
public static final int DERIVED = 4;
/**
* Scene7 specification used in getAssociatedAssets - Array of assets that were used to generate the specified asset.
*/
public static final int GENERATOR = 5;
/**
* Scene7 specification used in getAssociatedAssets - Array of assets that were generated from the specified asset.
*/
public static final int GENERATED = 6;
/**
* Returns the domain name of the S7 publish server
*
* @param s7Config
* @return A string, {@code null} if not present
* @throws AssertionError in case of IPS failures or undefined data format
*/
@Nullable
String getPublishServer(S7Config s7Config);
/**
* Returns the application property handle.
*
* @param s7Config
* the Scene7 configuration
* @return the application property handle, {@code null} if not present
* @throws AssertionError in case of IPS failures or undefined data format
*/
@Nullable
String getApplicationPropertyHandle(S7Config s7Config);
/**
* Returns the video encoder preset type handle.
*
* @param s7Config
* the Scene7 configuration
* @return the video encoder preset type handle, {@code null} if not present
* @throws AssertionError in case of IPS failures or undefined data format
*/
@Nullable
String getVideoEncoderPresetTypeHandle(S7Config s7Config);
/**
* Returns the handle for IPS PropertySet type by its name.
*
* @param s7Config the Scene7 configuration
* @return the PropertySet type's handle, or {@code null} if not present
* @throws AssertionError in case of IPS or other failure
*/
@Nullable
String getPropertySetTypeHandle(S7Config s7Config, String typeName);
/**
* Returns the video encoder preset type handle.
*
* @param typeHandle
* - the type handle
* @param s7Config
* the Scene7 configuration
* @return the list of property sets
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getPropertySets(String typeHandle, S7Config s7Config);
/**
* Create PropertySet of given type, and store given Properties to it.
*
* @param s7Config the Scene7 configuration
* @param typeHandle PropertySet type handle
* @param props array of Properties (Key-Value pairs) to put into new PropertySet
* @return the handle of newly created PropertySet
* @throws AssertionError in case of IPS or other failure
*/
String createPropertySet(S7Config s7Config, String typeHandle, Map props);
/**
* Update the PropertySet with the new set of Properties, replacing existing ones.
*
* @param s7Config the Scene7 configuration
* @param setHandle PropertySet handle
* @param props array of Properties (Key-Value pairs) to put into PropertySet
* @throws AssertionError in case of IPS or other failure
*/
void updatePropertySet(S7Config s7Config, String setHandle, Map props);
/**
* Update single property in the PropertySet.
*
* @param setHandle
* the set handle for the company settings
* @param propKey
* property key name
* @param propValue
* property key value
* @param s7Config
* the Scene7 configuration
* @throws AssertionError in case of IPS or other failure
*/
void updatePropertySet(String setHandle, String propKey, String propValue, S7Config s7Config);
/**
* Returns a unique user handle from Scene7.
*
* @param resourceResolver
* resource resolver used to access Scene7 configuration
* @param email
* E-Mail address
* @param password
* Password
* @param region
* Region of the Scene7 service
* @return A unique user handle
* @throws AssertionError in case of IPS failures or undefined data format
*/
String getUserHandle(ResourceResolver resourceResolver, String email,
String password, String region);
/**
* Returns a JSON representation of the company membership information
* provided by Scene7. The information includes a company handle, a company
* name and an asset root path.
*
* For example:
*
* {
* handle: 'jlkj98',
* name: 'Acme Ltd.',
* rootPath: '/MyAssets'
* }
*
*
*
* @param resourceResolver
* resource resolver used to access Scene7 configuration
* @param userHandle
* Unique user handle
* @param email
* E-Mail address
* @param password
* Password
* @param region
* Region of the Scene7 service
* @return A JSON string
* @throws AssertionError in case of IPS failures or undefined data format
*/
String getCompanyMembership(ResourceResolver resourceResolver,
String userHandle, String email, String password, String region);
/**
* Returns a Scene7Folder
holding a tree structure
*
* @param folderPath
* - the top folder of the request - does not need to be the root.
* @param depth
* - depth of the returned folder tree.
* @param responseFields
* - fields to be filled in for each node in the tree
* @param excludeFields
* - fields to be excluded in each node of the tree
* @param s7Config
* @return A Scene7Folder
object containing the requested folder structure
* @throws AssertionError in case of IPS failures or undefined data format
*/
Scene7Folder getFolderTree(String folderPath, int depth, String[] responseFields, String[] excludeFields, S7Config s7Config);
/**
* Returns s7 folder handle for the associated cq folder
*
* @param cqFolderPath
* - the path the target cq folder targeted in the s7 folder organization
* @param s7Config
* @return folder handle or null if the folder does not exist in s7.
* @throws AssertionError in case of IPS failures or undefined data format
*/
String getFolderHandle(String cqFolderPath, S7Config s7Config);
/**
* Returns a String success, note that the underlying S7 API call does not provide a data response.
*
* @param folderHandle
* - folder to be deleted
* @param s7Config
* - s7 config based on cloud service config info.
* @return String success
* @throws AssertionError in case of IPS failures
*/
String deleteFolder(String folderHandle, S7Config s7Config);
/**
* Returns a String success, note that the underlying S7 API call does not provide a data response.
*
* @param assetHandle
* - asset to be deleted
* @param s7Config
* - s7 config based on cloud service config info.
* @return String success
* @throws AssertionError in case of IPS failures or undefined data format
*/
String deleteAsset(String assetHandle, S7Config s7Config);
/**
* Delete multiple assets
* Returns a String success, note that the underlying S7 API call does not provide a data response.
*
* @param assetHandleArr
* - asset to be deleted
* @param s7Config
* - s7 config based on cloud service config info.
* @return String success
* @throws AssertionError in case of IPS failures or undefined data format
*/
String deleteAssets(String[] assetHandleArr, S7Config s7Config);
/**
* Returns a DOM list with search results (from metadata)
*
* @param folder
* - all matched assets will be in this folder
* @param includeSubfolders
* - whether to recursively search for assets
* @param assetTypes
* - all matched assets will be one of these types
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param published
* - whether the matched assets must be marked for publish (only marked - not necessarily actually published)
* @param conditions
* - metadata conditions for search
* @param s7Config
* @return A list of assets; the list can be empty if no assets have been found.
* @throws AssertionError in case of IPS failures
*/
List searchAssetsByMetadata(String folder, Boolean includeSubfolders, String[] assetTypes, String[] assetSubTypes,
Boolean published, MetadataCondition[] conditions, S7Config s7Config);
/**
* Submit a job to S7 for reprocessing video thumbnail to a specified video frame time
* @param s7Config the Scene7 configuration
* @param jobName the name of the job
* @param assetHandle the asset handle
* @param thumbnailTime the frame time
* @return the scene7 jobHandle, return empty string if failed to get it
*/
String submitReprocessAssetsThumbnailJob(S7Config s7Config, String jobName, String assetHandle, long thumbnailTime);
/**
* Returns a DOM list with search results (from metadata), support specify
*
* @param folder
* - all matched assets will be in this folder
* @param includeSubfolders
* - whether to recursively search for assets
* @param assetTypes
* - all matched assets will be one of these types
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param published
* - whether the matched assets must be marked for publish (only marked - not necessarily actually published)
* @param conditions
* - metadata conditions for search
* @param recordsPerPage
* maximum number of results to return
* @param resultsPage
* specifies the page of results to return, based on {@code recordsPerPage} page size
* @param s7Config
* @return A list of assets; the list can be empty if no assets have been found.
* @throws AssertionError in case of IPS failures
*/
List searchAssetsByMetadata(String folder, Boolean includeSubfolders, String[] assetTypes,
String[] assetSubTypes, Boolean published, MetadataCondition[] conditions, int recordsPerPage,
int resultsPage, S7Config s7Config);
/**
* Returns a Scene7Asset
list, based on a list of asset handles
*
* @param assetHandles
* - the assets to retrieve
* @param responseFields
* - fields to be filled in for each node in the tree
* @param excludeFields
* - fields to be excluded in each node of the tree
* @param s7Config
* @return A Scene7Asset
list
* @throws AssertionError in case of IPS failures
*/
List getAssets(String[] assetHandles, String[] responseFields, String[] excludeFields, S7Config s7Config);
/**
* Returns a single Scene7Asset
object. If there are multiple
* matching assets found, return the first asset.
*
* @param assetHandle
* - the asset to retrieve
* @param responseFields
* - fields to be filled in for each node in the tree
* @param excludeFields
* - fields to be excluded in each node of the tree
* @param s7Config
* - Scene7 cloud configuration object
* @return A Scene7Asset
object, or null if not found or failed
* @throws AssertionError in case of IPS failures
*/
@Nullable
Scene7Asset getAsset(String assetHandle, String[] responseFields,
String[] excludeFields, S7Config s7Config);
/**
* Returns an Arraylist of job details objects
*
* @param jobHandle
* - the handle for the job to get details.
* @param s7Config
* @return An AssetList of UploadJobDetail
* @throws AssertionError in case of IPS failures or undefined data format
*/
ArrayList getMultiFileJobLogDetails(String jobHandle, S7Config s7Config);
/**
* @param jobHandle
* of the job
* @param s7Config
* @return true
if active job with this jobHandle or orginalName is active, false
otherwise
* @throws AssertionError in case of IPS failures or undefined data format
*/
boolean isJobActiveByJobHandle(String jobHandle, S7Config s7Config);
/**
* @param originalName
* of the job
* @param s7Config
* @return true
if active job with this jobHandle or orginalName is active, false
otherwise
* @throws AssertionError in case of IPS failures or undefined data format
*/
boolean isJobActiveByOriginalName(String originalName, S7Config s7Config);
/**
* Returns a list of Scene7 asset handles(Strings) for the given job handle
*
* @param jobHandle
* - the handle for the job to get details.
* @param s7Config
* @return A list containing asset handles strings
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getJobLogDetails(String jobHandle, S7Config s7Config);
/**
* Returns a list of UploadJobDetail objects for the given job name
*
* @param jobName
* - the handle for the job to get details.
* @param s7Config
* @return A list containing UploadJobDetail
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getJobLogDetailsByJobName(String jobName, S7Config s7Config);
/**
* Get scene7 job status with given jobName, the jobName should be uniq, if there are many job have same name, it only get the first one
* @param s7Config the Scene7 configuration
* @param jobName the name of the job
* @return a string indicate the result, can be "NotFound", "Done" or a string returned by the S7 API, like "Running"
*/
String getJobStatus(S7Config s7Config, String jobName);
/**
* Returns a list of Scene7 image preset names for the given {@code S7Config}
*
* @param s7Config
* @return
* a list containing the names of the image presets defined in SPS
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getImagePresets(S7Config s7Config);
/**
* Retrieves the server used for serving and manipulating flash templates, depending on the Scene7 configuration.
*
* @param s7Config
* the Scene7 configuration
* @return a String containing the server's URL
*/
@Deprecated
String getFlashTemplatesServer(S7Config s7Config);
/**
* Retrieves a list of {@code Scene7Asset}s from the Scene7 server.
*
* @param folder
* the folder from which the assets are retrieved
* @param includeSubfolders
* whether to recursively search for assets in sub-folders of the folder where the search is performed
* @param published
* whether the matched assets must be marked for publish (only marked - not necessarily actually published)
* @param assetTypes
* all matched assets will be one of these types
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param responseFields
* the response fields that should be used to populate the {@code Scene7Asset}'s attributes
* @param excludeFields
* fields to be excluded from the response
* @param s7Config
* the Scene7 configuration
* @return a List of assets; can be empty if no assets have been found
* @throws AssertionError in case of IPS failures
*/
List searchAssets(String folder, Boolean includeSubfolders, Boolean published, String[] assetTypes,
String[] assetSubTypes, String[] responseFields, String[] excludeFields, S7Config s7Config);
/**
* Retrieves a list of {@code Scene7Asset}s from the Scene7 server.
*
* @param folder
* the folder from which the assets are retrieved
* @param includeSubfolders
* whether to recursively search for assets in sub-folders of the folder where the search is performed
* @param published
* whether the matched assets must be marked for publish (only marked - not necessarily actually published)
* @param assetTypes
* all matched assets will be one of these types
* @param assetSubTypes
* array of sub Asset Types to include in search
* @param responseFields
* the response fields that should be used to populate the {@code Scene7Asset}'s attributes
* @param excludeFields
* fields to be excluded from the response
* @param recordsPerPage
* maximum number of results to return
* @param resultsPage
* specifies the page of results to return, based on {@code recordsPerPage} page size
* @param s7Config
* the Scene7 configuration
* @return a List of assets; can be empty if no assets have been found
* @throws AssertionError in case of IPS failures
*/
List searchAssets(String folder, Boolean includeSubfolders, Boolean published, String[] assetTypes,
String[] assetSubTypes, String[] responseFields, String[] excludeFields, final int recordsPerPage,
final int resultsPage, S7Config s7Config);
/**
* Retrieve a list of {@code Scene7Asset}s from the Scene7 server by
* filename.
*
* @param folder
* @param includeSubfolders
* @param published
* @param filename
* @param s7Config
* @return
* @throws AssertionError in case of IPS failures
*/
List searchAssetsByFilename(String folder,
Boolean includeSubfolders, Boolean published, String filename,
S7Config s7Config);
/**
* Retrieve a list of {@code Scene7Asset}s from the Scene7 server by Scene7
* asset name.
*
* @param folder
* remote search folder path
* @param includeSubfolders
* to include children folders
* @param published
* to include published or non-published assets
* @param name
* Scene7 name of target asset
* @param s7Config
* Scene7 cloud configuration
*
* @throws AssertionError
* in case of IPS failures
*/
List searchAssetsByName(String folder,
Boolean includeSubfolders, Boolean published, String name,
S7Config s7Config);
/**
* Retrieve a list of {@code Scene7Asset}s from the Scene7 server by Scene7
* asset name with partial name search option
*
* @param folder
* remote search folder path
* @param includeSubfolders
* to include children folders
* @param published
* to include published or non-published assets
* @param name
* Scene7 name of target asset
* @param conditionMode
* partial name search condition mode. Currently supported modes
* are "Equals" and "StartsWith"
* @param s7Config
* Scene7 cloud configuration
* @return a list of {@code Scene7Asset}s
*/
List searchAssetsByPartialName(String folder,
Boolean includeSubfolders, Boolean published, String name,
String conditionMode, S7Config s7Config);
/**
* Retrieves the generated assets associated with the given input asset
*
* @param asset
* - the asset to get the sub-assets and generator from
* @param s7Config
* - the Scene7 configuration
* @return the original Scene7Asset
object updated with the
* sub-assets and the originator asset
* @throws AssertionError in case of IPS failures
*/
@Nullable
Scene7Asset getGeneratedAssets(Scene7Asset asset, S7Config s7Config);
/**
* Retrieves the sub-assets and the origiator asset associated with the
* given asset
*
* @param asset
* - the asset to get the sub-assets and generator from
* @param s7Config
* - the Scene7 configuration
* @return the original Scene7Asset
object updated with the
* sub-assets and the originator asset
* @throws AssertionError in case of IPS failures
*/
@Nullable
Scene7Asset getAssociatedAssets(Scene7Asset asset, S7Config s7Config);
/**
* Retrieves the originator asset associated with the given asset
*
* @param asset
* - the asset to get the originator asset
* @param s7Config
* - the Scene7 configuration
* @return the original Scene7Asset
object updated with the
* originator asset
* @throws AssertionError in case of IPS failures
*/
@Nullable
Scene7Asset getMasterAsset(Scene7Asset asset, S7Config s7Config);
/**
* Retrieves the server handling FXG rendering. Deprecated and only return null now.
*
* @param s7Config the Scene7 configuration
* @return a String containing the FXG server URL
*/
@Deprecated
String getFxgServer(S7Config s7Config);
/**
* Set the publish state of a given Scene7 asset
*
* @param resource
* activated resource
* @param markForPublish
* value of markForPublish flag
* @param s7Config
* the Scene7 configuration
* @return String success / failure
* @throws AssertionError in case of IPS failures or undefined data format
*/
String setAssetPublishState(Resource resource, boolean markForPublish,
S7Config s7Config);
/**
* Set the publish state of a given Scene7 asset
*
* @param assetHandle
* unique asset identifier
* @param markForPublish
* value of markForPublish flag
* @param s7Config
* the Scene7 configuration
* @return String success / failure
* @throws AssertionError in case of IPS failures
*/
String setAssetPublishState(String assetHandle, boolean markForPublish,
S7Config s7Config);
/**
* Set the publish state of a given Scene7 assets
*
* @param assetHandles
* asset identifiers to set publish state
* @param markForPublish
* value of markForPublish flag
* @param s7Config
* the Scene7 configuration
* @return String success / failure
* @throws AssertionError in case of IPS failures
*/
String setAssetsPublishState(Set assetHandles, boolean markForPublish, S7Config s7Config);
/**
* Create a folder on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param cqFolderPath
* the path the target cq folder targeted in the s7 folder
* organization
* @return folder handle
* @throws AssertionError in case of IPS failures or undefined data format
*/
String createFolder(S7Config s7Config, String cqFolderPath);
/**
* Create an ImageSet asset on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param folder
* relative target folder based on S7 Config root folder. If not
* exists, will attempt to create it
* @param name
* name for new ImageSet
* @param type
* type of new ImageSet (ImageSet, SwatchSet, RenderSet)
* @param resource
* (optional) if exists, jcr resource to receive metadata from new object
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set
* @return asset handle of newly created ImageSet, or null on error
* @throws AssertionError
* in case of IPS failures or undefined data format
*/
@Nullable
String createImageSet(
S7Config s7Config,
String folder,
String name,
String type,
@Nullable Resource resource,
@Nullable String thumbAssetHandle);
/**
* Create an ImageSet asset on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param imageSetFolder
* the path the target imageset cq folder targeted in the s7
* folder organization
* @param imageSetName
* name of the image set
* @param thumbAssetHandle
* (optional) user defined thumbnail asset handle
* @return asset handle of new image set
* @deprecated Use {@link #createImageSet(S7Config, String, String, String, Resource, String)}
*/
@Deprecated
String createImageSet(
S7Config s7Config,
@Nullable String imageSetFolder,
String imageSetName,
@Nullable String thumbAssetHandle);
/**
* Update an ImageSet on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle of the ImageSet to update
* @param members
* (optional) list of ImageSet members (Null = not updated)
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for set (Null = not updated)
* @throws AssertionError in case of IPS failures
*/
void updateImageSet(S7Config s7Config, String assetHandle,
@Nullable ImageSetMemberUpdateArray members, @Nullable String thumbAssetHandle);
/**
* Update an ImageSet asset on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param imageSetAssetHandle
* image set Scene7 asset handle
* @param setMemberHandles
* asset handles of image set members
* @param thumbAssetHandle
* (optional) user defined thumbnail asset handle
* @deprecated Use
* {@link #updateImageSet(S7Config, String, ImageSetMemberUpdateArray, String)}
*/
@Deprecated
void updateImageSet(S7Config s7Config,
String imageSetAssetHandle,
List setMemberHandles,
@Nullable String thumbAssetHandle);
/**
* Get members of legacy ImageSet
*
* @param s7Config the Scene7 configuration
* @param assetHandle asset handle of the ImageSet
* @return not-null ordered list of members embedded into {@link ImageSetMemberArray} instance
*/
ImageSetMemberArray getImageSetMembers(S7Config s7Config, String assetHandle);
/**
* Create an AssetSet asset on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param folder
* relative target folder based on S7 Config root folder. If not
* exists, will attempt to create it
* @param name
* name for new AssetSet
* @param type
* type of new AssetSet
* @param resource
* (optional) if exists, jcr resource to receive metadata from new object
* @param setDefinition
* (optional) set's definition string
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for
* set
* @return asset handle of newly created AssetSet, or null on error
* @throws AssertionError
* in case of IPS failures or undefined data format
*/
@Nullable
String createAssetSet(
S7Config s7Config,
String folder,
String name,
String type,
@Nullable Resource resource,
@Nullable Scene7AssetSet setDefinition,
@Nullable String thumbAssetHandle);
/**
* Update an AssetSet on Scene7 server
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle of the AssetSet to update
* @param setDefinition
* (optional) set's definition string (Null = not updated)
* @param thumbAssetHandle
* (optional) asset handle of user-defined thumbnail image for set (Null = not updated)
* @throws AssertionError in case of IPS failures
*/
void updateAssetSet(S7Config s7Config, String assetHandle,
@Nullable Scene7AssetSet setDefinition, @Nullable String thumbAssetHandle);
/**
* Create a preset view to determine what users can see.
*
* The preset view is applied when assets are published.
*
* @param s7Config
* the Scene7 configuration
* @param folderHandle
* folder handle of the folder that contains the assets
* @param name
* name for the viewer
* @param type
* type of any available viewer in IPS
* @param resource
* (optional) if exists, jcr resource to receive metadata from new object
* @param configSettings
* (optional) list containing {@link Scene7ConfigSetting} values to apply
* @return viewer preset handle or {@code null} if unsuccessful
* @throws AssertionError in case of IPS failures or undefined data format
*/
String createViewerPreset(
S7Config s7Config,
String folderHandle,
String name,
String type,
@Nullable Resource resource,
@Nullable List configSettings);
/**
* Create a preset view to determine what users can see.
*
* The preset view is applied when assets are published.
*
* @param s7Config
* the Scene7 configuration
* @param folderHandle
* folder handle of the folder that contains the assets
* @param name
* name for the viewer
* @param type
* type of any available viewer in IPS
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @return viewer preset handle or {@code null} if unsuccessful
* @deprecated Use {@link #createViewerPreset(S7Config, String, String,
* String, Resource, List)}
*/
@Deprecated
String createViewerPreset(S7Config s7Config,
String folderHandle, String name,
String type,
@Nullable List configSettings);
/**
* Get the viewer configuration for the referenced asset
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset of interest
*
* @return {@link Scene7ViewerConfig} with the information or {@code null}
* if unsuccessful
* @throws AssertionError in case of IPS failures or undefined data format
*/
Scene7ViewerConfig getViewerConfigSettings(
S7Config s7Config,
String assetHandle);
/**
* Attach viewer configuration settings to an asset
*
* Can be viewer preset or the source asset for the viewer
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset to modify
* @param name
* name of the asset
* @param type
* type of any available viewer in IPS
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @throws AssertionError in case of IPS failures
*/
void setViewerConfigSettings(
S7Config s7Config,
String assetHandle,
String name,
String type,
List configSettings);
/**
* Updates SWF viewer configuration settings.
*
* @param s7Config
* the Scene7 configuration
* @param assetHandle
* asset handle for the asset to modify
* @param configSettings
* list containing {@link Scene7ConfigSetting} values to apply
* @throws AssertionError in case of IPS failures
*/
void updateViewerConfigSettings(
S7Config s7Config,
String assetHandle,
List configSettings);
/**
* Creates a user account and adds the account to one of more companies
*
* @param s7Config
* The Scene7 configuration
* @param firstName
* The user's first name
* @param lastName
* The user's last name
* @param email
* The user's email address
* @param defaultRole
* The role for the user in the companies they belong, the
* IpsAdmin role overrides other per-company settings
* @param password
* The user's password
* @param passwordExpires
* The instant of expiration of the password
* @param isValid
* Determine the user's validity
* @param membershipArray
* List of {@link Scene7CompanyMembership} entries enumerating the
* membership of the user.
* @return The handle of the newly created user
* @throws AssertionError in case of IPS failures or undefined data format
*/
String addUser(
S7Config s7Config,
String firstName,
String lastName,
String email,
String defaultRole,
String password,
@Nullable Instant passwordExpires,
boolean isValid,
List membershipArray);
/**
* Return all users
*
* @param s7Config
* The Scene7 configuration
* @param includeInvalid
* Indicate if invalid users should be included
* @return List containing {@link Scene7User} requested
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getAllUsers(
S7Config s7Config,
boolean includeInvalid);
/**
* Return a optionally sorted, filtered and paged list user results
*
* @param s7Config
* The Scene7 config
* @param includeInactive
* Include or exclude inactive members
* @param includeInvalid
* Include or exclude invalid members
* @param companyHandleArray
* Filter results be company
* @param groupHandleArray
* Filter results by group
* @param userRoleArray
* Filter results by user role
* @param charFilterField
* Filter results by field's string prefix
* @param charFilter
* Filter results by a specific character
* @param sortBy
* Choice of user sort field
* @param recordsPerPage
* Number of records per page to return
* @param resultsPage
* The desired result page
* @return List containing {@link Scene7User} requested
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getUsers(
S7Config s7Config,
@Nullable Boolean includeInactive,
@Nullable Boolean includeInvalid,
@Nullable List companyHandleArray,
@Nullable List groupHandleArray,
@Nullable List userRoleArray,
@Nullable String charFilterField,
@Nullable String charFilter,
@Nullable String sortBy,
@Nullable Integer recordsPerPage,
@Nullable Integer resultsPage);
/**
* Set user attributes
*
* @param s7Config
* The Scene7 config
* @param userHandle
* User handle
* @param firstName
* First name
* @param lastName
* Last name
* @param email
* Email address
* @param defaultRole
* The role for the user in the companies they belong, the
* IpsAdmin role overrides other per-company settings
* @param passwordExpires
* The password's expiration
* @param isValid
* Determine if valid IPS user
* @param membershipArray
* List of {@link Scene7CompanyMembership} entries enumerating the
* membership of the user
* @throws AssertionError in case of IPS failures
*/
void setUserInfo(
S7Config s7Config,
@Nullable String userHandle,
String firstName,
String lastName,
String email,
String defaultRole,
@Nullable Instant passwordExpires,
boolean isValid,
List membershipArray);
/**
* Deletes an image format.
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Handle to the image format. (This is returned from
* {@link #saveImageFormat(S7Config, String, String, String, Resource)}
* @param path
* If available, path to JCR resource backing the image format
*
* @return String success
* @throws AssertionError in case of IPS failures
*/
String deleteImageFormat(
S7Config s7Config,
String imageFormatHandle,
@Nullable String path);
/**
* Deletes an image format.
* @deprecated in favour of {@link #deleteImageFormat(S7Config, String, String)}
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Handle to the image format. (This is returned from
* {@link #saveImageFormat(S7Config, String, String, String, Resource)}
*
* @return String success
* @throws AssertionError in case of IPS failures
*/
@Deprecated
String deleteImageFormat(
S7Config s7Config,
String imageFormatHandle);
/**
* Returns image formats, such as PDF, EPS, SWF, and others.
*
* @param s7Config
* The Scene7 config
* @return A list of {@link Scene7ImageFormat} objects
* @throws AssertionError in case of IPS failures or undefined data format
*/
List getImageFormats(S7Config s7Config);
/**
* Create an image format.
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Image format handle you want to save
* @param name
* Image format name
* @param urlModifier
* IPS protocol query string
* @param resource
* (optional) if exists, jcr resource to receive metadata from new object
*
* @return The imageFormatHandle
* @throws AssertionError
* in case of IPS failures or undefined data format
*/
String saveImageFormat(
S7Config s7Config,
@Nullable String imageFormatHandle,
String name,
String urlModifier,
@Nullable Resource resource);
/**
* Create an image format.
*
* @param s7Config
* The Scene7 config
* @param imageFormatHandle
* Image format handle you want to save
* @param name
* Image format name
* @param urlModifier
* IPS protocol query string
*
* @return The imageFormatHandle
* @deprecated Use
* {@link #saveImageFormat(S7Config, String, String, String, Resource)}
*/
@Deprecated
String saveImageFormat(
S7Config s7Config,
@Nullable String imageFormatHandle,
String name,
String urlModifier);
/**
* Deletes an image map
*
* @param s7Config
* The Scene7 config
* @param imageMapHandle
* Handle for the image map to delete
* @throws AssertionError in case of IPS failures
*/
void deleteImageMap(
S7Config s7Config,
String imageMapHandle);
/**
* Create a new image map or edit an existing map
*
* @param s7Config
* The Scene7 config
* @param assetHandle
* Handle to the asset the map belongs to
* @param imageMapHandle
* Handle to the image map.
* @param name
* Name of the image map
* @param shapeType
* Choice of region shape
* @param region
* A comma delimited list of points that define the region
* @param action
* The href value associated with the image map
* @param position
* The order in the list of image maps
* @param enabled
* Whether this map is enabled
* @return The handle to the new or edited image map
* @throws AssertionError in case of IPS failures or undefined data format
*/
String saveImageMap(
S7Config s7Config,
String assetHandle,
@Nullable String imageMapHandle,
String name,
String shapeType,
String region,
String action,
int position,
boolean enabled);
/**
* Sets the image map for an asset.
*
* You must have already created the image maps. Image maps are applied in
* order of retrieval from the array. This means the second image map
* overlays the first, the third overlays the second, and so on.
*
* @param s7Config
* The Scene7 config
* @param assetHandle
* Handle to the asset
* @param imageMap
* list of predefined {@link Scene7ImageMapDefinition} objects
* @return list of image map handles applied to the asset
* @throws AssertionError in case of IPS failures or undefined data format
*/
List setImageMaps(
S7Config s7Config,
String assetHandle,
List imageMap);
/**
* Sets asset metadata using batch mode.
* @param s7Config
* The Scene7 config
* @param updates
* List of {@link Scene7ImageFieldUpdate} to be applied
* @return {@link Scene7BatchMetadataResult} containing details
* on any warnings or errors
* @throws AssertionError in case of IPS failures or undefined data format
*/
Scene7BatchMetadataResult batchSetAssetMetadata(
S7Config s7Config, List updates);
/**
* Sets asset metadata using batch mode.
*
* @param s7Config
* The Scene7 config
* @param updateArray
* List of {@link Scene7ImageFieldUpdate} to be applied
* @return {@link Scene7BatchMetadataResult} containing details
* on any warnings or errors
* @throws AssertionError in case of IPS failures or undefined data format
*/
Scene7BatchMetadataResult batchSetImageFields(
S7Config s7Config, List updateArray);
/**
* Retrieves Scene7 remote asset preview /is/image/ URL (context path with
* image server uri). If resource is null, then returns DMS7 default
* S7Config preview URL
*
* @param resource
* Scene7 sync-ed asset in DAM
* @param configResolver
* resource resolver with access to S7Config object
* @return String Scene7 asset preview URL
*/
@Nullable
String getPreviewServerUrl(Resource resource,
ResourceResolver configResolver);
/**
* Retrieves Scene7 remote asset publish /is/image/ URL (server domain and
* image server uri). If resource is null, then returns DMS7 default
* S7Config publish URL
*
* @param resource
* Scene7 sync-ed asset in DAM
* @param configResolver
* resource resolver with access to S7Config object
* @return String Scene7 asset publish URL
*/
@Nullable
String getPublishServerUrl(Resource resource,
ResourceResolver configResolver);
/**
* Retrieves Scene7 remote video preview /is/content/ URL (context path with
* image server uri). If resource is null, then returns DMS7 default
* S7Config preview URL
*
* @param resource
* Scene7 sync-ed asset in DAM
* @param configResolver
* resource resolver with access to S7Config object
* @return String Scene7 asset preview URL
*/
@Nullable
String getPreviewVideoServerUrl(Resource resource,
ResourceResolver configResolver);
/**
* Retrieves Scene7 remote video publish /is/content/ URL (server domain and
* image server uri). If resource is null, then returns DMS7 default
* S7Config preview URL
*
* @param resource
* Scene7 sync-ed asset in DAM
* @param configResolver
* resource resolver with access to S7Config object
* @return String Scene7 asset publish URL
*/
@Nullable
String getPublishVideoServerUrl(Resource resource,
ResourceResolver configResolver);
/**
* Retrieves a metadata map (key-value pair) associated with a Scene7 asset
*
* @param s7Config
* The Scene7 cloud configuration object
* @param assetHandle
* Scene7 unique asset identifier
* @return Map key-value pairs metadata of Scene7 asset
* @throws AssertionError in case of IPS failures or undefined data format
*/
@Nullable
Map getS7AssetMetadata(S7Config s7Config, String assetHandle);
/**
* Retrieves a list of Scene7 video renditions with their names and asset
* handles
*
* @param s7Config
* The Scene7 cloud configuration object
* @param assetHandle
* Scene7 unique asset identifier
* @return List a list of Scene7Assets containing video rendition names and
* asset handles
* @throws AssertionError in case of IPS failures or undefined data format
*/
@Nullable
List getS7VideoRenditions(S7Config s7Config, String assetHandle);
/**
* Returns property {@code dam:scene7ID} of a given {@code Resource} object
*
* @param resource
* Resource object on which to get Scene7 metadata
* @return String value of {@code dam:scene7ID} associated to
* {@code Resource} or null if property is unavailable
*/
@Nullable
String getScene7ID(Resource resource);
/**
* Returns true if {@code Resource} object is linked to Scene7 based on its metadata
*
* @param resource
* Resource object on which to get Scene7 metadata
* @return true if {@code Resource} object has {@code dam:scene7ID} metadata
*/
boolean isResourceLinkedToScene7(Resource resource);
/**
* Returns property {@code dam:scene7CloudConfigPath} of a given
* {@code Resource} object
*
* @param resource
* Resource object on which to get Scene7 metadata
* @return String value of {@code dam:scene7CloudConfigPath} associated to
* {@code Resource} or null if property is unavailable
*/
@Nullable
String getLinkedScene7ConfigPath(Resource resource);
@Nullable
List getContainerAssets(Scene7Asset scene7Asset,
S7Config s7Config);
/**
* Moves an asset to a specific folder
*
* @param s7Config
* Scene7 S7Config object
* @param resource
* Resource object to be relocated
* @param folder
* Destination folder path
* @return true/false of the moveAsset() operation succeeded
*/
boolean moveAsset(S7Config s7Config, Resource resource, String folder);
/**
* Moves multiple assets independently of each other
*
* @param s7Config
* Scene7 S7Config object
* @param resourcesToFolders
* Mapping of AEM resource to destination folder
* @return true/false of the moveAssets() operation succeeded
*/
boolean moveAssets(S7Config s7Config,
Map resourcesToFolders);
/**
* Move a folder to a new location
*
* @param s7Config
* Scene7 S7Config object
* @param sourceFolder
* Path to source folder
* @param destinationFolder
* Path to destination folder
* @return true/false of the moveFolder() operation succeeded
*/
boolean moveFolder(S7Config s7Config, String sourceFolder,
String destinationFolder);
/**
* Finds and moves generated assets to a specific folder
*
* @param s7Config
* Scene7 S7Config object
* @param resource
* Resource object to be relocated
* @param folder
* Destination folder path
* @return true/false of the moveAssociatedAsset() operation succeeded
*/
boolean moveAssociatedAsset(S7Config s7Config, Resource resource,
String folder);
/**
* Rename an asset
*
* @param s7Config
* Scene7 S7Config object
* @param resource
* Resource object to be renamed
* @param name
* Asset's new name
* @return true/false of the renameAsset() operation succeeded
*/
boolean renameAsset(S7Config s7Config, Resource resource, String name);
/**
* Rename a folder
*
* @param s7Config
* Scene7 S7Config object
* @param folder
* Folder to be renamed
* @param name
* Folder's new name
* @return true/false of the renameFolder() operation succeeded
*/
boolean renameFolder(S7Config s7Config, String folder, String name);
/**
* Update one or more Smart Crop sub asset(s)
*
* @param s7Config
* Scene7 S7Config object
* @param updateSmartCropMap
* Map of owner handle, sub-asset asset handles and
* Scene7NormalizedCropRect objects
* @return true/false of the updateSmartCrops() operation succeeded
*/
@Deprecated
boolean updateSmartCrops(S7Config s7Config,
Map> updateSmartCropMap);
/**
*
* @param s7Config Scene7 S7Config object
* @param asset asset for updating associated smart crops
* @param smartCrops list of smart crops to be updated
* @return true/false if the update is successful
*/
boolean updateSmartCrops(S7Config s7Config, com.day.cq.dam.api.Asset asset,
List smartCrops);
/**
* Set the video thumbnail with a specifed thumbnail asset, not only for master video, all generated encoded videos and AVS files are
* also been set together
* @param s7Config the Scene7 configuration
* @param assetHandle the S7 assetHandle of the master video that you want to set thumbnail
* @param thumbAssetHandle the S7 assetHandle of the thumbnail asset
* @return
*/
Map setVideoThumbnail(S7Config s7Config, String assetHandle, String thumbAssetHandle);
/**
* Retrieves an XMP Metadata packet for the specified asset.
* @param s7Config the Scene7 configuration
* @param assetHandle Handle to the asset you want to get XMP metadata
* @return XMP metadata for the given assetHandle in XML format
*/
String getXMPPacket(S7Config s7Config, String assetHandle);
/**
* Sets or updates an XMP metadata packet for an asset.
* @param s7Config the Scene7 configuration
* @param assetHandle Handle to the asset you want to get XMP metadata
* @param xmpPacketAsXml updated XMP packet to update asset metadata in XML format
*/
void updateXMPPacket(S7Config s7Config, String assetHandle, String xmpPacketAsXml);
/**
* Checks the login in Scene7 with the given credentials
* @param resourceResolver
* resource resolver used to access Scene7 configuration
* @param email Email id of the user
* @param password Password of the user
* @param region the user's region
* @return the login status for the user.
*/
String getLoginStatus(ResourceResolver resourceResolver, String email, String password, String region);
/**
* Sets the password for a given user with an expiry of 100 years ahead from the current date.
* @param s7Config
* Scene7 configuration object
* @param userHandle
* combination of IPS user handle and email id
* @param password
* Password to be set
* @param validityInDays Password validity in number of days. Set it to null/zero for default expiry of 100 years.
*/
void setPassword(S7Config s7Config,String userHandle, String password, @Nullable Integer validityInDays);
/**
* Forwards the supplied list of URLs to the Scene7 CDN (Content Distribution Network) provider
* to invalidate their existing cache of HTTP responses
* @param s7Config Scene7 configuration object
* @param urlArray List of up to 1000 URLs to invalidate from the CDN cache
* @return CdnCacheInvalidationReturn IPS api response
*/
CdnCacheInvalidationReturn cdnCacheInvalidation(S7Config s7Config, UrlArray urlArray);
/**
* Forwards the supplied list of companyAliasURLs to the Scene7 CDN (Content Distribution Network) provider
* to invalidate their existing cache of HTTP responses.
* @param s7Config Scene7 configuration object
* @param companyAliasUrlArray List of up to 1000 URLs to invalidate from the CDN cache
* @return CdnCacheInvalidationReturn IPS api response
*/
CdnCacheInvalidationReturn flushCdnCacheByAlias(S7Config s7Config, UrlArray companyAliasUrlArray);
/**
* Gets the token for a given company to access secure preview IS and stores in repository to cache token.
* @param s7Config
* Scene7 configuration object
*/
String getAndStoreSecureISAuthToken(S7Config s7Config);
/**
* Deletes the sub assets for an asset
* @param asset Asset whose smart crops need to be removed
* @param subAssetHandles list of scene7 handles to the sub assets
* @param s7Config the scene7 configuration
*
* @return true if successfully deleted all subassets.
*/
boolean deleteSubAssets(Asset asset, List subAssetHandles, S7Config s7Config);
/**
* Gets the value of the ipsImageUrl property for an asset
* @param asset Asset whose ipsImageUrl need to be fetched
* @param s7Config Scene7 configuration object
* @return ipsImageUrl for the asset. It may return null if ipsImageUrl is not present.
*/
@Nullable
String getIpsImageUrl(Asset asset, S7Config s7Config);
/**
* Returns the Company object
* @param companyName company name
* @param s7Config Scene7 configuration object
* @return the Company object corresponding to the companyName
*
* @throws AssertionError in case of IPS failures or undefined data format
*/
Company getCompanyInfoForCompanyName(String companyName, S7Config s7Config);
/**
* Returns the manifest url for videos
* @param resource video resource
* @param manifestType type of video streaming manifest being requested
* @param onlyIfPublished return a manifest only if the video is published
* @return the manifest url for videos
*
* @throws Exception
*/
@Nullable
String getVideoManifestURI(Resource resource, ManifestType manifestType, boolean onlyIfPublished) throws Exception;
/**
* Update audio metadata and avs id for video asset node which were processed before multi audio / caption changes
* @param resource video resource
*/
void setAvsHandleAndOriginalAudioMetadata(Resource resource);
/**
* Parse AVS asset handle and original audio metadata from received AVS data
* @param metadata map where default metadata will be updated
* @param asset Scene7 asset parsed from AVS response
* @return metadata map
*/
Map parseAvsHandleAndOriginalAudioMetadata(Asset asset, Map metadata, Scene7Asset scene7Asset);
/**
* Create a template on Scene7 server
*
* @param templateProperties the Scene7 template properties
* @param s7Config the Scene7 configuration
* @return template asset handle
* @throws AssertionError in case of IPS failures or undefined data format
*/
String createTemplate(Map templateProperties, S7Config s7Config);
/**
* Update the URL Modifier for a template on Scene7 server
*
* @param assetHandle the Scene7 template asset handle
* @param modifier the URL modifier to set
* @param s7Config the Scene7 configuration
* @throws AssertionError in case of IPS failures
*/
boolean setUrlModifier(String assetHandle, String modifier, S7Config s7Config);
/**
* Parse AVS content and returns list of file paths contained in video manifest
* @param avsFileName avs name
* @param s7Config Scene7 config
* @return List of file paths present in video manifest i.e. m3u8, mpd
*/
public List getVideoManifestAssociatedFiles(String avsFileName, S7Config s7Config) throws IllegalArgumentException, URISyntaxException, IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy