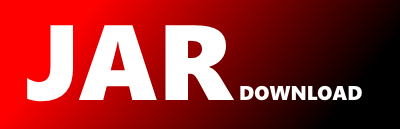
com.day.cq.mcm.campaign.GenericCampaignConnector Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.mcm.campaign;
import java.util.Map;
/**
* A service that is used to access a remote Adobe Campaign instance.
*/
public interface GenericCampaignConnector extends CampaignConnector {
/**
* Calls a remote JSSP on the campaign instance.
*
* Note that the caller is required to use {@link CallResults#destroy()} after
* processing the result of the remote function call.
*
* This method supports generic namespaces, hence the namespace must be included
* in the name of the function (parameter name
).
*
* @param name The name of the function to be called (including the namespace)
* @param fctParams The parameters of the function call (name/value parameters)
* @param credentials The credentials to be used for the call
* @return The result of the function call
* @throws ACConnectorException If the remote function could not be called or returned an unexpected status
*/
CallResults callGeneric(String name, Map fctParams,
CampaignCredentials credentials) throws ACConnectorException;
/**
* Posts data to a remote function on the campaign instance.
*
* Note that the caller is required to use {@link CallResults#destroy()} after
* processing the result of the remote function call.
*
* This method supports generic namespaces, hence the namespace must be included
* in the name of the function (parameter name
).
*
* @param name The name of the function to be called
* @param data The data to post (name/value)
* @param credentials The credentials to be used for the call
* @return The result of the function call
* @throws ACConnectorException If the remote function could not be called or returned an unexpected status
*/
CallResults postGeneric(String name, Map data,
CampaignCredentials credentials) throws ACConnectorException;
/**
* Gets data from a given URL with the credentials passed as Basic Authentication
* headers.
*
* Note that the caller is required to use {@link CallResults#destroy()} after
* processing the result of the remote function call.
*
* This is (for example) the counterpart for Campaign .next's query retrieval.
*
* @param path The (absolute) path to call
* @param queryString The query string (without leading '?')
* @param credentials The credentials
* @return The result of the function call
* @throws ACConnectorException If the remote function could not be called or returned an unexpected status
*/
CallResults callGenericWithBasicAuth(String path, String queryString,
CampaignCredentials credentials)
throws ACConnectorException;
}