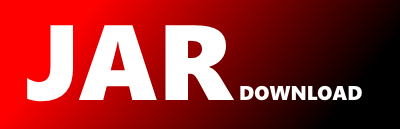
com.day.cq.replication.ReplicationContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.replication;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
import java.util.Collections;
/**
* This interface describes the assembled content to replicate.
*/
public interface ReplicationContent {
/**
* Implements a void replication content to be used for delete or flush
* actions.
*/
ReplicationContent VOID = new ReplicationContent() {
/**
* {@inheritDoc}
*
* @return null
*/
public InputStream getInputStream() throws IOException {
return null;
}
/**
* {@inheritDoc}
*
* @return null
*/
public String getContentType() {
return null;
}
/**
* {@inheritDoc}
*
* @return -1
*/
public long getLastModified() {
return -1;
}
/**
* {@inheritDoc}
*
* @return 0
*/
public long getContentLength() {
return 0;
}
/**
* {@inheritDoc}
*
* Does nothing.
*/
public void acquire(String agentName) {
}
/**
* {@inheritDoc}
*
* Does nothing.
*/
public void release(String agentName) {
}
/**
* {@inheritDoc}
*
* @return an empty collection
*/
public Collection getAcquiredBy() {
return Collections.emptySet();
}
/**
* {@inheritDoc}
*
* does nothing
*/
public void destroy() {
}
/**
* {@inheritDoc}
*
* @return null
*/
public ReplicationContentFacade getFacade() {
return null;
}
/**
* Returns "ReplicationContent.VOID"
* @return "ReplicationContent.VOID"
*/
@Override
public String toString() {
return "ReplicationContent.VOID";
}
};
/**
* Get the input stream for the content.
* @return return the input stream for this content or null
if the underlying data source does not
* exist anymore.
*
* @throws IOException if an I/O error occurrs
*/
InputStream getInputStream() throws IOException;
/**
* Get the content type
* @return The content type or null.
*/
String getContentType();
/**
* Return the content length if known
* @return Return the content length or -1 if the length is unknown.
*/
long getContentLength();
/**
* Returns the last modified time or -1 if unknown
* @return the last modified time
*/
long getLastModified();
/**
* Marks this content to be used by the given agent.
*
* @param agentName name of the agent
*/
void acquire(String agentName);
/**
* Mark that this replication content is not needed anymore for this agent.
* This must only be called when the replication is not present any more in any queues handled by this agent.
*
* @param agentName The name of the agent.
*/
void release(String agentName);
/**
* Returns the collection of agent names that use this content.
*
* @return the names
*/
Collection getAcquiredBy();
/**
* Destroy the cached content.
* This destroys the cached content regardless of the current
* value of the usage counter.
*/
void destroy();
/**
* Returns the facade for this content.
* @return the facade
*/
ReplicationContentFacade getFacade();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy