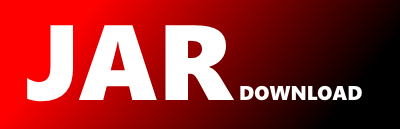
com.day.cq.replication.ReplicationOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.replication;
import java.util.HashSet;
import java.util.Set;
/**
* The ReplicationOptions
encapsulate optional
* configuration parameters for a replication.
*/
public class ReplicationOptions {
/**
* Should the replication happen synchronously? (default is false)
*/
private boolean synchronous = false;
/**
* Agent filter
*/
private AgentFilter filter;
/**
* the replication listener
*/
private ReplicationListener listener;
/**
* the revision to replicate
*/
private String revision;
/**
* if true
, the replication status properties are not updated
*/
private boolean suppressStatusUpdate;
/**
* if true
the implicit versioning should not be triggered
*/
private boolean suppressVersions;
/**
* if false
the replication is free to split the provided paths
* into chunks and replicate them in a non-atomic manner.
*/
private boolean useAtomicCalls = false;
/**
* if false
the replication pre-processors are disabled and won't be executed
*/
private boolean disablePreProcessors = false;
/**
* handler to use for generating aggregates
* @since 5.5
*/
private AggregateHandler aggregateHandler;
private boolean updateAlias;
/**
* @link {@link Set} to hold all alias which are already replicated.
*/
private Set aliasReplicated = new HashSet();
/**
* By default audit logs will be created
*/
private boolean enableAuditLog = true;
/**
* Create a new options object with default values
*/
public ReplicationOptions() {
// default values
}
/**
* Should the replication be done synchronous or asynchronous?
* The default is asynchronously.
* @return true
for synchronous replication
*/
public boolean isSynchronous() {
return synchronous;
}
/**
* Set the synchronous flag.
* @param synchronous true
for synchronous replication
*/
public void setSynchronous(boolean synchronous) {
this.synchronous = synchronous;
}
/**
* Set the enableAuditLog event flag
*
* @param enableAuditLog false
skips the creation of the audit log
*/
public void setEnableAuditLog(boolean enableAuditLog) {
this.enableAuditLog = enableAuditLog;
}
/**
* @return if audit logging is enabled
*/
public boolean getEnableAuditLog() {
return this.enableAuditLog;
}
/**
* Set the desired agent ids. Please note, that this method internally
* sets a new {@link AgentIdFilter}.
*
* @param desiredAgentId agent ids
* @deprecated use {@link #setFilter(AgentFilter)} instead.
*/
@Deprecated
public void setDesiredAgentIDs(String ... desiredAgentId) {
if (desiredAgentId != null) {
setFilter(new AgentIdFilter(desiredAgentId));
}
}
/**
* Sets the filter for selecting the agents for the replication.
* @param filter agent filter
*/
public void setFilter(AgentFilter filter) {
this.filter = filter;
}
/**
* Returns the filter for selecting the agents.
* @return the filter
*/
public AgentFilter getFilter() {
return filter;
}
/**
* Returns the revision to replicate
* @return the revision
*/
public String getRevision() {
return revision;
}
/**
* Sets the revision to replicate.
* @param revision the revision name.
*/
public void setRevision(String revision) {
this.revision = revision;
}
/**
* Returns the replication listener.
* @return the listener or null
*/
public ReplicationListener getListener() {
return listener;
}
/**
* Sets the replication listener. Please note that a listener is currently
* only called for synchronous replication.
*
* @param listener the replication listener.
*/
public void setListener(ReplicationListener listener) {
this.listener = listener;
}
/**
* If true
, the replication will not update the replication
* status properties after a replication.
* @return true
if status updates should be suppressed.
*
* @since 5.4
*/
public boolean isSuppressStatusUpdate() {
return suppressStatusUpdate;
}
/**
* If set to true
the replication will not update the
* replication status properties after a replication.
* @param suppressStatusUpdate true
if status updates should be suppressed
*
* @since 5.4
*/
public void setSuppressStatusUpdate(boolean suppressStatusUpdate) {
this.suppressStatusUpdate = suppressStatusUpdate;
}
/**
* If true
the replication will not trigger implicit versioning.
* @return true
if implicit versioning should be suppressed
*
* @since 5.4
*/
public boolean isSuppressVersions() {
return suppressVersions;
}
/**
* If set to true
the replication will not trigger implicit
* versioning.
* @param suppressVersions set to true
if implicit versioning should be suppressed
*
* @since 5.4
*/
public void setSuppressVersions(boolean suppressVersions) {
this.suppressVersions = suppressVersions;
}
/**
* Returns the defined aggregate handler or null
* @return the aggregate handler.
*
* @since 5.5
*/
public AggregateHandler getAggregateHandler() {
return aggregateHandler;
}
/**
* Sets the aggregate handler.
* @param aggregateHandler the aggregate handler
*
* @since 5.5
*/
public void setAggregateHandler(AggregateHandler aggregateHandler) {
this.aggregateHandler = aggregateHandler;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append("ReplicationOptions");
sb.append("{synchronous=").append(synchronous);
sb.append(", revision='").append(revision).append('\'');
sb.append(", suppressStatusUpdate=").append(suppressStatusUpdate);
sb.append(", suppressVersions=").append(suppressVersions);
sb.append(", filter=").append(filter);
sb.append(", aggregateHandler=").append(aggregateHandler);
sb.append(", updateAlias=").append(updateAlias);
sb.append(", useAtomicCalls=").append(useAtomicCalls);
sb.append(", disablePreProcessors=").append(disablePreProcessors);
sb.append('}');
return sb.toString();
}
/**
*
* @param updateAlias the boolean value to set the updateAlias to
* @since 5.9
*/
public void setUpdateAlias(boolean updateAlias) {
this.updateAlias = updateAlias;
}
/**
*
* @return the boolean value for the updateAlias
* @since 5.9
*/
public boolean isUpdateAlias() {
return updateAlias;
}
/**
* Allows the system to split the provided paths into multiple independent batches, thus
* not replicating all provided paths in an atomic fashion. By default atomic calls are configured.
* @param useAtomicCalls false
if a large batch of paths should be replicated, but
* atomicity is not required.
* @deprecated no longer required
*/
public void setUseAtomicCalls(boolean useAtomicCalls) {
// ignore
}
/**
* Allows the system to split the provided paths into multiple independent batches, thus
* not replicating all provided paths in an atomic fashion
* @return false
if a large batch of paths should be replicated, but
* atomicity is not required.
* @deprecated no longer required
*/
public boolean isUseAtomicCalls() {
return this.useAtomicCalls;
}
/**
* Add path to already replicated alias set.
* @param path the path
*/
public void addToAliasReplicated(String path) {
aliasReplicated.add(path);
}
/**
* Returns true if path is already replicated else false.
* @param path the path
* @return {@code true} if path is already replicated, else {@code false}
*/
public boolean isAliasReplicated(String path) {
return aliasReplicated.contains(path);
}
/**
* If pre processors disabled or enabled
*
* @return the boolean value for disablePreProcessors
*/
public boolean isDisablePreProcessors() {
return disablePreProcessors;
}
/**
*
* @param disablePreProcessors boolean value for disabling pre-processors
*/
public void setDisablePreProcessors(boolean disablePreProcessors) {
this.disablePreProcessors = disablePreProcessors;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy