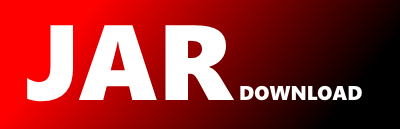
com.day.cq.replication.ReplicationResult Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.replication;
import static com.day.cq.replication.ReplicationResultInfo.NONE;
/**
* The ReplicationResult
is returned by the {@link TransportHandler}
* after sending a request to the recipient. The instance conveys information
* on the success of request delivery and request processing. Optionally it also adds
* additional information for the result through {@link ReplicationResultInfo}
*/
public class ReplicationResult {
/**
* OK result.
*/
public static final ReplicationResult OK = new ReplicationResult(true, 200, "OK");
/**
* Flag indicating whether the replication was successful.
*/
private final boolean success;
/**
* Status code.
*/
private final int code;
/**
* Status message.
*/
private final String message;
private final ReplicationResultInfo resultInfo;
/**
* Creates an instance of this class.
*
* @param success See {@link #isSuccess()}
* @param code See {@link #getCode()}
* @param message See {@link #getMessage()}
* @param resultInfo See {@link #getReplicationResultInfo()}
*/
public ReplicationResult(boolean success, int code, String message, ReplicationResultInfo resultInfo) {
this.success = success;
this.code = code;
this.message = message;
if (resultInfo == null) {
resultInfo = NONE;
}
this.resultInfo = resultInfo;
}
/**
* Creates an instance of this class.
*
* @param success See {@link #isSuccess()}
* @param code See {@link #getCode()}
* @param message See {@link #getMessage()}
*/
public ReplicationResult(boolean success, int code, String message) {
this.success = success;
this.code = code;
this.message = message;
this.resultInfo = NONE;
}
/**
* Returns true
If the replication action and optional content
* could be sent to the recipient. false
is returned if there
* was a transfer problem.
*
* Note that this flag indicates whether the recipient received the
* request for further processing. It does not indicate whether the
* recipient could do something useful with it.
*
* @return {@code true} If the replication action and optional content
* could be sent to the recipient. {@code false} is returned if there
* was a transfer problem.
*/
public boolean isSuccess() {
return success;
}
/**
* Returns some status code indication sent by the recipient about the
* success of processing the replication action and optional content.
*
* For example an HTTP based transport agent may set this field to the HTTP
* response status code.
*
* @return some status code indication sent by the recipient about the
* success of processing the replication action and optional content.
*/
public int getCode() {
return code;
}
/**
* Returns some status message providing clear text indication sent by the
* recipient about the success of processing the replication action and
* optional content.
*
* For example an HTTP based transport agent may set this field to the HTTP
* response status message.
*
* @return some status message providing clear text indication sent by the
* recipient about the success of processing the replication action and
* optional content.
*/
public String getMessage() {
return message;
}
/**
* Returns any additional information for replication action
*
* @return additional info
*/
public ReplicationResultInfo getReplicationResultInfo() {
return resultInfo;
}
@Override
public String toString() {
return "ReplicationResult{" +
"success=" + isSuccess() +
", code=" + getCode() +
", message=" + getMessage() +
", info={id=" + resultInfo.getArtifactId() + "}" +
'}';
}
}