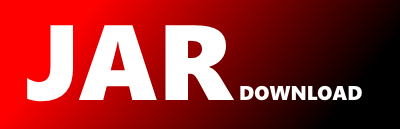
com.day.cq.replication.ReplicationStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.cq.replication;
import java.util.Calendar;
import java.util.List;
import java.util.Set;
import org.osgi.annotation.versioning.ProviderType;
/**
* Determines the replication status of an asset/page.
*/
@ProviderType
public interface ReplicationStatus {
/**
* The name of the property that records the date of the last replication.
*/
static final String NODE_PROPERTY_LAST_REPLICATED = "cq:lastReplicated";
/**
* The name of the property that records the userid of the last replication
*/
static final String NODE_PROPERTY_LAST_REPLICATED_BY = "cq:lastReplicatedBy";
/**
* The name of the property that records the last replication action
*/
static final String NODE_PROPERTY_LAST_REPLICATION_ACTION = "cq:lastReplicationAction";
/**
* Name of the mixin node type
*/
static final String NODE_TYPE = "cq:ReplicationStatus";
/**
* Extended mixin for new properties
*/
static final String EXTENDED_TYPE = "cq:ReplicationStatus2";
/**
* Name of the property that records if the content has been imported by golden-publish
*/
static final String NODE_IS_DELIVERED = "cq:isDelivered";
/**
* @deprecated use {@link #NODE_PROPERTY_LAST_REPLICATED}
*/
@Deprecated
static final String NODE_PROPERTY_LAST_PUBLISHED = "cq:lastPublished";
/**
* @deprecated use {@link #NODE_PROPERTY_LAST_REPLICATED_BY}
*/
@Deprecated
static final String NODE_PROPERTY_LAST_PUBLISHED_BY = "cq:lastPublishedBy";
/**
* Returns the time when the content was last published (i.e. activated or deactivated).
* @return last publish date or null
*/
Calendar getLastPublished();
/**
* Returns the user id who issues the last publish action.
* @return The publish user id or null
*/
String getLastPublishedBy();
/**
* Return the last replication action.
* @return The action type or null
*/
ReplicationActionType getLastReplicationAction();
/**
* Checks if the content is delivered.
*
* Note that this is calculated only by the logs of replication actions.
* It does not check if the content is physically on the server. a content is
* delivered if the last action was 'Activate' and the action is processed.
* of if the action was 'deactivate' and the action is still pending.
*
* @return true
if the content is delivered.
*/
boolean isDelivered();
/**
* @deprecated use {@link #isDelivered()}
* @return same as {@link #isDelivered()}
*/
@Deprecated
boolean isPublished();
/**
* Convenience method that checks if the last publish action was
* 'activate'. Note that !isActivated() does not automatically mean that
* the content is deactivated.
*
* @return true
if the last publish action was 'activate'
*/
boolean isActivated();
/**
* Convenience method that checks if the last publish action was
* 'deactivate'. Note that !isDeactivated() does not automatically mean that
* the content is activated.
*
* @return true
if the last publish action was 'activate'
*/
boolean isDeactivated();
/**
* Checks if the last publish action is pending, i.e. if it's still present
* in any of the queues.
* @return true
if the action is pending
*/
boolean isPending();
/**
* Checks if the last publish action is still pending, i.e. if it's still
* present in any of the queues.
* @return the list of pending queue entries
*/
List getPending();
/**
* Returns the queue status of this content. if this content is not queued at
* all, an empty list is returned.
* @return a queue status for each queue.
*/
List getQueueStatus();
/**
* Returns all agents for which a replication status is available; these agents must be
* present and active in the system.
* @return the agents for which a replication status is available, or an empty set if no
* status is available for an agent.
* @since 6.6.0
*/
public Set getAvailableAgents();
/**
* Return the replication status for exactly one agent.
* @param agentName the name of an existing agent (as returned by getAvailableAgents()
)
* @return the ReplicationStatus for that agent; null
if the replication agent
* does not exist or no status is available for it.
* @since 6.6.0
*/
public ReplicationStatus getStatusForAgent(String agentName);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy