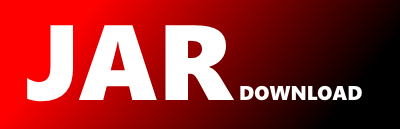
com.day.cq.reporting.AggregateManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.reporting;
import java.util.HashMap;
import java.util.Map;
/**
* This class is used for managing aggregates; mainly for mapping aggregate types
* to corresponding {@link Aggregate}s.
*/
// TODO: replace MedianAggregate and Percentile95Aggregate by Generics
public class AggregateManager {
/**
* The service to retrieve the aggregates from.
*/
private final ComponentProvider componentService;
/**
* Map that defines the aggregate on a column base
*/
protected Map aggregatePerColumn;
/**
* Creates a new AggregateManager.
*
* @param context The context for creating the report
*/
public AggregateManager(Context context) {
this.componentService = (context != null ? context.getComponentService() : null);
this.aggregatePerColumn = new HashMap(16);
}
/**
* Creates an {@link Aggregate} object for the specified aggregate type.
*
* @param type The type of the aggregate
* @return The aggregate
*/
private Aggregate createAggregateFromType(String type) {
Aggregate aggregate = this.componentService.acquireAggregate(type);
if (aggregate == null) {
throw new IllegalArgumentException("Aggregate type '" + type + "' is not "
+ "supported by this implementation.");
}
return aggregate;
}
/**
* Creates the aggregate for the specified column.
*
* @param col The aggregate for the specified column
*/
public void createForColumn(Column col) {
String type = col.getAggregateType();
if (type != null) {
Aggregate existingAggregate = this.aggregatePerColumn.get(col);
if (existingAggregate != null) {
this.componentService.releaseAggregate(existingAggregate);
}
this.aggregatePerColumn.put(col, this.createAggregateFromType(type));
}
}
/**
* Gets the aggregate for the specified column.
*
* @param col The column
* @return The aggregate; null
if no aggregate is available for the
* specified column
*/
public Aggregate getForColumn(Column col) {
return this.aggregatePerColumn.get(col);
}
/**
* Cleans up the aggregates that were used.
*/
public void cleanup() {
for (Column col : this.aggregatePerColumn.keySet()) {
Aggregate aggregateToRelease = this.aggregatePerColumn.get(col);
this.componentService.releaseAggregate(aggregateToRelease);
}
this.aggregatePerColumn.clear();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy