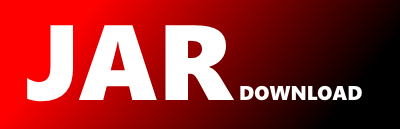
com.day.cq.rewriter.linkchecker.LinkInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.rewriter.linkchecker;
import java.util.Calendar;
import java.util.HashSet;
import java.util.Set;
/**
* Stores information about the validity of an external link.
*/
public class LinkInfo {
public static final int X_STATUS_NOT_CHECKED = 0;
public static final int X_STATUS_UNSUPPORTED = -1;
public static final int X_STATUS_URI_SYNTAX_ERROR = -2;
public static final int X_STATUS_CONNECT_ERROR = -3;
public static final int X_STATUS_NO_ROUTE_TO_HOST = -4;
public static final int X_STATUS_UNKOWN_HOST = -5;
public static final int X_STATUS_IO_ERROR = -6;
public static final int X_STATUS_SSL_ERROR = -7;
public static final int X_STATUS_READ_TIME_OUT_ERROR = -8;
private final String url;
private Calendar lastChecked;
private Calendar lastAccessed;
private Calendar lastAvailable;
private boolean valid;
private int lastStatus;
private final Set referencedBy = new HashSet();
/**
* Initializes a new instance.
*
* @param url The URL to store validity information about
*/
public LinkInfo(String url) {
this.url = url;
this.lastAccessed = Calendar.getInstance();
valid = true;
}
/**
* Initializes a new instance based on the given one.
* @param base info to copy from
*/
public LinkInfo(LinkInfo base) {
url = base.url;
lastChecked = base.lastChecked;
lastAccessed = base.lastAccessed;
lastAvailable = base.lastAvailable;
lastStatus = base.lastStatus;
valid = base.valid;
referencedBy.addAll(base.referencedBy);
}
/**
* Returns the date a check for validity of the URL has last been requested.
*
* @return The date the check for validity of the URL has last been requested
*/
public Calendar getLastAccessed() {
return lastAccessed;
}
/**
* Sets the date a check for validity of the URL has last been requested.
*
* @param lastAccessed The date the check for validity of the URL has last been requested
*/
public void setLastAccessed(Calendar lastAccessed) {
this.lastAccessed = lastAccessed;
}
/**
* Returns the date the URL has last been checked by the asynchronous LinkCheckerTask.
*
* @return The date the URL has last been checked
*/
public Calendar getLastChecked() {
return lastChecked;
}
/**
* Sets the date the URL has last been checked by the asynchronous LinkCheckerTask.
*
* @param lastChecked The date the URL has last been checked
*/
public void setLastChecked(Calendar lastChecked) {
this.lastChecked = lastChecked;
}
/**
* Returns the date the resource described by the URL has last been available.
*
* @return The date the resource described by the URL has last been available.
*/
public Calendar getLastAvailable() {
return lastAvailable;
}
/**
* Sets the date the resource described by the URL has last been available.
*
* @param lastAvailable The date the resource described by the URL has last been available.
*/
public void setLastAvailable(Calendar lastAvailable) {
this.lastAvailable = lastAvailable;
}
/**
* Returns the URL about which validity information is stored.
*
* @return The URL about which validity information is stored
*/
public String getUrl() {
return url;
}
/**
* Returns whether the resource described by the URL is currently available.
*
* @return true, if the resource described by the URL is currently available
*/
public boolean isValid() {
return valid;
}
/**
* Sets whether the resource described by the URL is currently available.
*
* @param valid Whether the resource described by the URL is currently available
*/
public void setValid(boolean valid) {
this.valid = valid;
}
/**
* Returns the last status
* @return the last status
*/
public int getLastStatus() {
return lastStatus;
}
public String getLastStatusAsString() {
switch (lastStatus) {
case X_STATUS_CONNECT_ERROR:
return "No Connection";
case X_STATUS_IO_ERROR:
return "I/O Error";
case X_STATUS_READ_TIME_OUT_ERROR:
return "Read Timeout";
case X_STATUS_SSL_ERROR:
return "SSL Error";
case X_STATUS_NO_ROUTE_TO_HOST:
return "No Route to Host";
case X_STATUS_NOT_CHECKED:
return "";
case X_STATUS_UNKOWN_HOST:
return "Unknown Host";
case X_STATUS_UNSUPPORTED:
return "Unsupported Protocol";
case X_STATUS_URI_SYNTAX_ERROR:
return "URI Syntax Error";
default:
return String.valueOf(lastStatus);
}
}
/**
* Sets the last status
* @param lastStatus the last status
*/
public void setLastStatus(int lastStatus) {
this.lastStatus = lastStatus;
}
/**
* Adds (if not already present) a referrer to this link.
* @param path the path of the node referencing this link
*/
public void addReferrer(String path) {
this.referencedBy.add(path);
}
/**
* Returns the paths referencing this link
* @return the paths referencing this link
*/
public String[] getReferrer() {
return this.referencedBy.toArray(new String[]{});
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LinkInfo linkInfo = (LinkInfo) o;
if (valid != linkInfo.valid) return false;
if (lastStatus != linkInfo.lastStatus) return false;
if (lastAccessed != null ? !lastAccessed.equals(linkInfo.lastAccessed) : linkInfo.lastAccessed != null)
return false;
if (lastAvailable != null ? !lastAvailable.equals(linkInfo.lastAvailable) : linkInfo.lastAvailable != null)
return false;
if (lastChecked != null ? !lastChecked.equals(linkInfo.lastChecked) : linkInfo.lastChecked != null)
return false;
//if (!referencedBy.equals(linkInfo.referencedBy)) return false;
return url.equals(linkInfo.url);
}
public boolean isSame(LinkInfo linkInfo) {
if (valid != linkInfo.valid) return false;
if (lastStatus != linkInfo.lastStatus) return false;
if (lastAvailable != null ? !lastAvailable.equals(linkInfo.lastAvailable) : linkInfo.lastAvailable != null)
return false;
if (lastChecked != null ? !lastChecked.equals(linkInfo.lastChecked) : linkInfo.lastChecked != null)
return false;
if (!referencedBy.equals(linkInfo.referencedBy)) return false;
return url.equals(linkInfo.url);
}
@Override
public int hashCode() {
int result = url.hashCode();
result = 31 * result + (lastChecked != null ? lastChecked.hashCode() : 0);
result = 31 * result + (lastAccessed != null ? lastAccessed.hashCode() : 0);
result = 31 * result + (lastAvailable != null ? lastAvailable.hashCode() : 0);
result = 31 * result + (valid ? 1 : 0);
result = 31 * result + lastStatus;
//result = 31 * result + referencedBy.hashCode();
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy