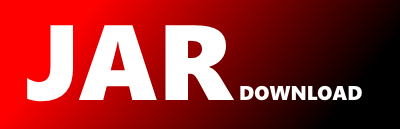
com.day.cq.search.eval.AbstractPredicateEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.search.eval;
import java.util.Comparator;
import javax.jcr.query.Row;
import com.day.cq.search.Predicate;
import com.day.cq.search.facets.FacetExtractor;
/**
* AbstractPredicateEvaluator
is a base implementation for
* predicate evaluators. Predicate evaluator implementations are encouraged
* to extend from this abstract base class to minimize migration efforts when
* new changes are added to the {@link PredicateEvaluator} API.
*
* This implementation basically does "nothing":
*
* - returns no xpath expression
* - does not filter
* - (implementations have to implement at least one of the two above)
* - provides no order by properties or order comparator
* - provides no facet extractor
* - automatically covers newer interface methods by implementing them using the old methods
*
*
* @since 5.2
*/
public abstract class AbstractPredicateEvaluator implements PredicateEvaluator {
/**
* Default implementation that always returns null
, ie. adds
* nothing to the XPath query. Subclasses can choose whether they want to
* implement this method or use the
* {@link #includes(Predicate, Row, EvaluationContext)} method for advanced
* filtering (or both).
*/
public String getXPathExpression(Predicate predicate, EvaluationContext context) {
// default implementation for subclasses that only implement includes()
return null;
}
/**
* Default implementation that always returns null
.
*/
public String[] getOrderByProperties(Predicate predicate, EvaluationContext context) {
return null;
}
/**
* Returns the inverted boolean value of the deprecated
* {@link #isFiltering(Predicate, EvaluationContext)} method (ie. if not
* overridden, true
).
*/
public boolean canXpath(Predicate predicate, EvaluationContext context) {
// capabilities were previously defined solely by isFiltering()
return !isFiltering(predicate, context);
}
/**
* Returns the same as the deprecated
* {@link #isFiltering(Predicate, EvaluationContext)} method (ie. if not
* overridden, false
).
*/
public boolean canFilter(Predicate predicate, EvaluationContext context) {
// capabilities were previously defined solely by isFiltering()
return isFiltering(predicate, context);
}
/**
* Default implementation that always returns false
, because
* the {@link #includes(Predicate, Row, EvaluationContext)} also always
* returns true
in this implementation and hence does no
* filtering at all.
*/
@Deprecated
public boolean isFiltering(Predicate predicate, EvaluationContext context) {
return false;
}
/**
* Default implementation that always returns true
, ie. it does
* not "touch" the result set at all.
*/
public boolean includes(Predicate predicate, Row row, EvaluationContext context) {
return true;
}
/**
* Default implementation that always returns null
.
*/
public Comparator getOrderByComparator(Predicate predicate, EvaluationContext context) {
return null;
}
/**
* Default implementation that always returns null
, ie. no
* facets will be extracted for the predicate.
*/
public FacetExtractor getFacetExtractor(Predicate predicate, EvaluationContext context) {
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy