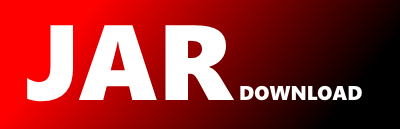
com.day.cq.search.eval.PermissionPredicateEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2014 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.search.eval;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import javax.jcr.UnsupportedRepositoryOperationException;
import javax.jcr.query.Row;
import javax.jcr.security.AccessControlManager;
import javax.jcr.security.Privilege;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.propertytypes.ServiceVendor;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.day.cq.search.Predicate;
/**
* Restricts the result to items where the current session has the specified
*
* JCR privileges.
*
*
* This is a filtering-only predicate and cannot leverage a search index.
* Does not support facet extraction.
*
*
Name:
* hasPermission
*
*
* Properties:
*
* - hasPermission
* - comma-separated JCR privileges that the current user session must ALL have
* for the node in question; for example
jcr:write,jcr:modifyAccessControl
*
*
* @since 6.1
*/
@ServiceVendor("Adobe Systems Incorporated")
@Component(factory = "com.day.cq.search.eval.PredicateEvaluator/hasPermission")
public class PermissionPredicateEvaluator extends AbstractPredicateEvaluator {
public static final String HAS_PERMISSION_PREDICATE = "hasPermission";
private static final Logger log = LoggerFactory.getLogger(PermissionPredicateEvaluator.class);
@Override
public boolean includes(Predicate p, Row row, EvaluationContext context) {
if (!p.hasNonEmptyValue(HAS_PERMISSION_PREDICATE)) {
return true;
}
try {
ResourceResolver resolver = context.getResourceResolver();
Session session = resolver.adaptTo(Session.class);
AccessControlManager acm = session.getAccessControlManager();
final Resource resource = context.getResource(row);
String permissions[] = p.get(HAS_PERMISSION_PREDICATE).split(",");
Privilege privileges[] = new Privilege[permissions.length];
for (int i = 0; i < permissions.length; i++) {
privileges[i] = acm.privilegeFromName(permissions[i]);
}
return acm.hasPrivileges(resource.getPath(), privileges);
} catch (UnsupportedRepositoryOperationException e) {
log.error("Exception occured while evaluating "
+ HAS_PERMISSION_PREDICATE + " predicate", e);
} catch (RepositoryException e) {
log.error("Exception occured while evaluating "
+ HAS_PERMISSION_PREDICATE + " predicate", e);
}
return false;
}
@Override
public boolean canXpath(Predicate predicate, EvaluationContext context) {
return false;
}
@Override
public boolean canFilter(Predicate predicate, EvaluationContext context) {
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy