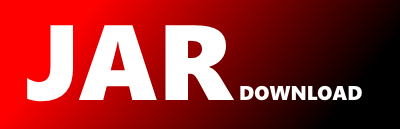
com.day.cq.search.facets.FacetExtractor Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.search.facets;
import javax.jcr.Node;
import javax.jcr.RepositoryException;
import com.day.cq.search.Query;
import com.day.cq.search.eval.PredicateEvaluator;
import com.day.cq.search.facets.extractors.FacetImpl;
/**
* FacetExtractor
extracts a {@link Facet} from a result set from a
* {@link Query}. For each node in the result, {@link #handleNode(Node)} will be
* called and the implementation should keep track of the values or rather
* {@link Bucket Buckets}. After the result set was scanned, {@link #getFacet()}
* will be called to retrieve the final {@link Facet}.
*
*
* Performance Note: In order to minimize the number of
* FacetExtractors
and Facets
, all implementations
* should properly implement the {@link #equals(Object)} (and thus also
* {@link #hashCode()}) methods so that the framework can remove duplicate
* FacetExtractors
before scanning the result nodes. The reason is
* that some {@link PredicateEvaluator Predicates} will create Facets
of the
* same type, since they follow the same definition, but have different
* user-chosen values to check against. As a Facet
tries to find
* all different values present in a search result, for the same type of
* PredicateEvaluator
or Facet
, they will return the same set
* of values / Buckets
.
*
*/
public interface FacetExtractor {
/**
* Called for each node of the result set.
*
* @param node
* node in the result
* @throws RepositoryException
* if access to the node failed, ie. one of the methods on node
* threw an exception
*/
void handleNode(Node node) throws RepositoryException;
/**
* Called after the result set was scanned (and {@link #handleNode(Node)}
* was called for each node in the result) to retrieve the final
* {@link Facet} object. Please note that this might be called without
* {@link #handleNode(Node)} ever being called, if the result was empty.
*
*
* Implementations can use the simple {@link FacetImpl} implementation
* of the {@link Facet} interface.
*
* @return an implementation of the {@link Facet} interface with all buckets
* found in the result or null
if nothing was found in
* the result
*/
Facet getFacet();
}